The Python split() method divides a string into a list. Values in the resultant list are separated based on a separator character. The separator is a whitespace by default. Common separators include white space and commas.
Dividng the contents of a string into a Python list is a common operation. For instance, you may have a list of ingredients in a recipe that you want to split up into a list.
That’s where the Python string split() method comes in. The split() method allows you to break up a string into a list of substrings, based on the separator you specify.
This tutorial will discuss, with examples, the basics of the Python split() function. By the end of reading this tutorial, you’ll be a master at using the split() string function.
Python Strings
Strings are collections of characters. They allow you to store and manipulate text-based data in your Python programs. Strings are one of Python’s sequence types. This is because they store data sequentially.
Here is an example of a string in Python:
example_string = "I am an example string!"
In this code, we assign the string “I am an example string!” to the variable example_string.
We can use a number of Python string methods to break our string up into smaller components. split() is one example of a string method that allows us to divide our string. Let’s explore how this method works.
Python String split()
The split() method splits a string into a list. A string is separated based on a separator character. By default, this character is a white space.
string_name.split(separator, maxsplit)
split() is a built-in function. This means you do not need to import any libraries to use the split() function. Here are the main components of the split() method:
- separator is the character the split() function will use to separate out the characters in the string. By default, any white space character will be used as a separator (space, newline). This parameter is optional.
- maxsplit defines the maximum number of splits that should occur. By default, maxsplit is equal to -1, which means an infinite number of splits will run. This parameter is also optional.
The Python split() string method is appended at the end of a string. This is common for string methods. You do not enclose the string that you want to split in the split() method like you would if you were printing a string. split() accepts two parameters:
The split() method returns a copy of the string represented as a list. This is because strings are immutable, which means they cannot be changed. split() does not modify your original string.
Let’s walk through a few examples of the split() Python function to demonstrate how it works.
Python Split String: Walkthrough
Suppose you have a string of ingredients for a chocolate chip muffin that you want to divide into a list. Each ingredient is separated by a comma in your string. By using the split() method, you can divide the string of ingredients up into a list.
Let’s divide our list of ingredients into a string:
ingredients = "2 eggs, 125ml Vegetable oil, 250ml Semi-skimmed milk, 250g golden caster sugar, 400g self-raising flour, 100g chocolate chips" list_of_ingredients = ingredients.split(",") print(list_of_ingredients)
Our code returns:
['2 eggs', ' 125ml Vegetable oil', ' 250ml Semi-skimmed milk', ' 250g golden caster sugar', ' 400g self-raising flour', ' 100g chocolate chips']
First, we define a variable called ingredients. This variable stores our list of ingredients. Each ingredient in our list is separated using a comma (,).
Next, we use the split() method to split up our ingredients string using commas. We use the separator parameter to tell Python that our string should be separated with a comma. Then, we assign the result of the split() method to the variable list_of_ingredients.
We print out the variable list_of_ingredients to the console. You can see that our code has turned our string into a list with six values: one for each ingredient.
Controlling the Number of Split Strings
We can use the maxsplit parameter to limit how many times our string should be split. Suppose we only want to split up the first three ingredients in our list. We could do so using this code:
ingredients = "2 eggs, 125ml Vegetable oil, 250ml Semi-skimmed milk, 250g golden caster sugar, 400g self-raising flour, 100g chocolate chips" list_of_ingredients = ingredients.split(",", 3) print(list_of_ingredients)
Our code returns:
['Two eggs', ' 125ml Vegetable oil', ' 250ml Semi-skimmed milk', ' 250g golden caster sugar, 400g self-raising flour, 100g chocolate chips']
We define a value for the maxsplit parameter in our code. The value of this parameter is set to 3. We have defined a comma as our separator character. We did this in our first example.
The maxsplit parameter our string will be divided into a maximum of three items. Then, the rest of the string will be added to the end of our list.
Our list now only has four items. The first three items are individual ingredients, and the last item in our list contains the rest of the items from our original string.
Conclusion
The Python string split() method allows you to divide a string into a list at a specified separator. For instance, you could use split() to divide a string by commas (,), or by the letter J.
This tutorial discussed the basics of strings in Python and how to use the string split() method. Now you’re ready to start using the split() method like a Python professional!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
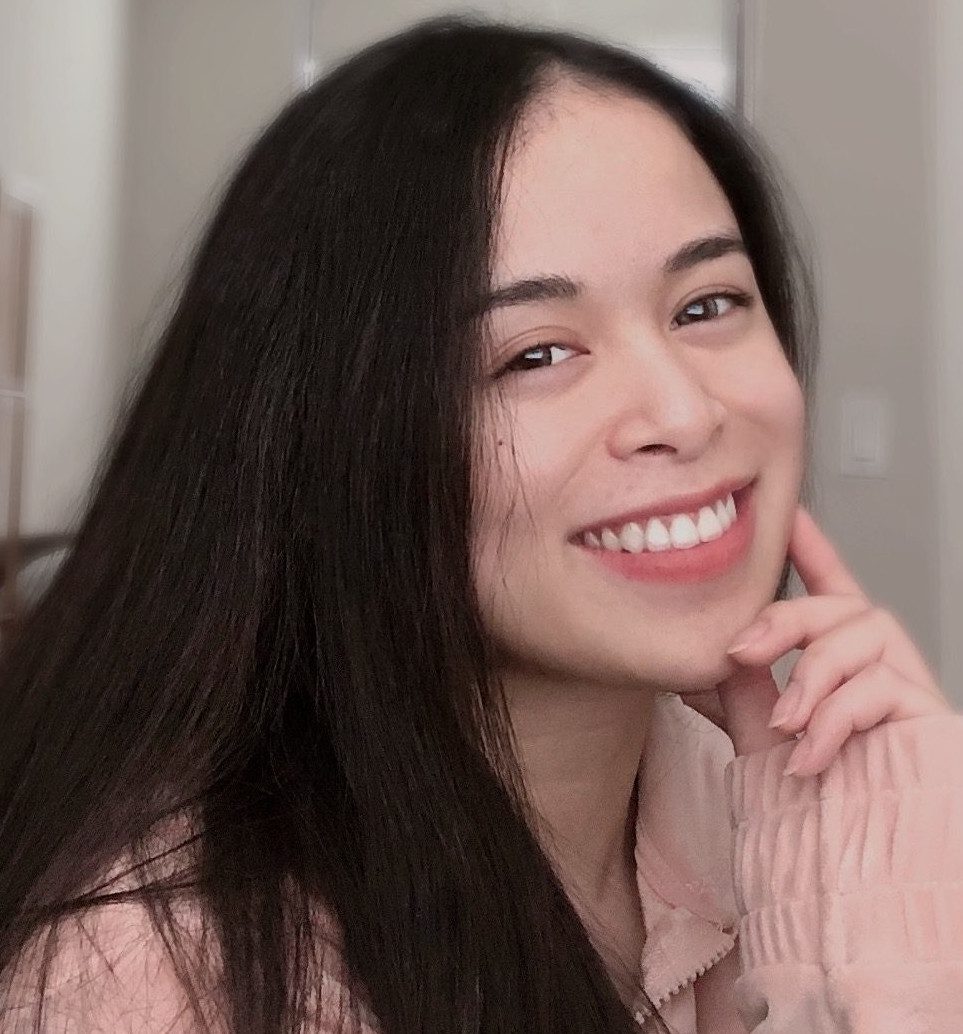
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot