The Python sort() method sorts a list in ascending order by its values. You can sort a list in descending order by using the reverse parameter. sort() optionally accepts a function that lets you specify a custom sort.
All coders eventually encounter a situation where they have to sort items or data. Sorting is critical in many contexts. You might need to sort usernames in reverse alphabetical order or get a list of email recipients in alphabetical order.
Python has a built-in function to help developers sort lists: sort(). The sort function offers many features to customize list ordering so you can arrange Python lists in the best way for your program.
In this tutorial, we’ll cover the basics of sorting in Python and discuss how to use the sort() function.
Python Sort List
The sort() function orders lists in ascending order. You can use a custom sort or sort in descending order by specifying additional parameters. These parameters are optional.
Let’s take a look at the syntax for the sort() method:
sort(reverse=True, key=function)
Our sort() method accepts two optional arguments:
- reverse: Specifying the value True sorts a list in descending order.
- key: A function that sorts the list.
Python Sort List: Ascending Order
Below, we define a Python array. This array contains a list of numbers. These numbers are not in any particular order. To sort our array, we can use the sort() function:
data = [9, 12, 8, 4, 6] data.sort() print(data)
Our code returns the following:
[4, 6, 8, 9, 12]
On the first line of our code, we declared a list of numbers that appear in random order.
Next, we use the sort() function with no arguments. This orders our original list in ascending alphabetical order. Then, on the final line, our code prints the sorted data.
The sort() function does changes our original array.
Sort List in Python: Descending Order
The reverse argument sorts a list in descending order when it is set to True. By default, reverse is set to False, which means our final list will be in ascending order.
Below, we have a list of student names. We want to sort this list in reverse alphabetical order. To do so, we’ll use the sort() method with the reverse argument:
students = ['Hannah', 'Arnold', 'Paul', 'Chris', 'Kathy'] students.sort(reverse=True) print(students)
Our code returns the list of student names in reverse alphabetical order:
['Paul', 'Kathy', 'Hannah', 'Chris','Arnold']
Sort Python Keys
We can sort our lists using our own methods of sorting. For example, we can sort an array by the length of the strings in the array.
Sort By String Length
By using the key parameter, we can define our own custom sort. Here is an example of the key argument in action that will sort our list by the length of its component strings:
students = ['Sophie', 'Alexander', 'Paul', 'Chris', 'Ann'] sortedStudents = sorted(students, key=len) print(sortedStudents)
Our code returns the following:
['Ann', 'Paul', 'Chris', 'Sophie', 'Alexander']
Here, our code has sorted our list by the length of the strings. Ann, a three-letter-long name, comes first, and Alexander, whose name consists of nine characters, comes last in our list.
In our above example, we tell our code to sort by len(), which is the built-in Python function for the length of an object. Each string in our array is run through len(). Then, the final list is ordered.
Similarly, we could have used str.upper to sort in alphabetical order by the uppercase letter in each string. Or, we could have used another Python function entirely to sort our list.
Sort by JSON Contents
Keys also allow you to define your own functions that state how a sort should be performed. Here’s an example of a sort that will reverse a JSON array by a certain value. In this case, the test scores of certain students:
def sortFunction(value): return value["test_score"] students = [ { "name": "Sophie", "test_score": 75 }, { "name": "Ann", "test_score": 67 }, { "name": "Chris", "test_score": 73 } ] sortedStudents = sorted(students, key=sortFunction) print(sortedStudents)
Our code returns the following:
[{'name': 'Ann', 'test_score': 67}, {'name': 'Chris', 'test_score': 73}, {'name': 'Sophie', 'test_score': 75}]
Our code has sorted the students by their test scores.
Firstly, our program defines a function called sortFunction, which will sort our JSON array based on the value of test_score when called. Then we declare a JSON array called students with student names and their test scores.
Next, we sort our students using our custom sort function by calling sortFunction as our key. On the final line, our code prints out our sorted list.
Conclusion
In this tutorial, we have broken down how to use the sort() function in Python to sort lists. We discussed how to use sort() on different data types and the reverse parameter to reverse list order. We also covered how to use keys to conduct more granular sorts.
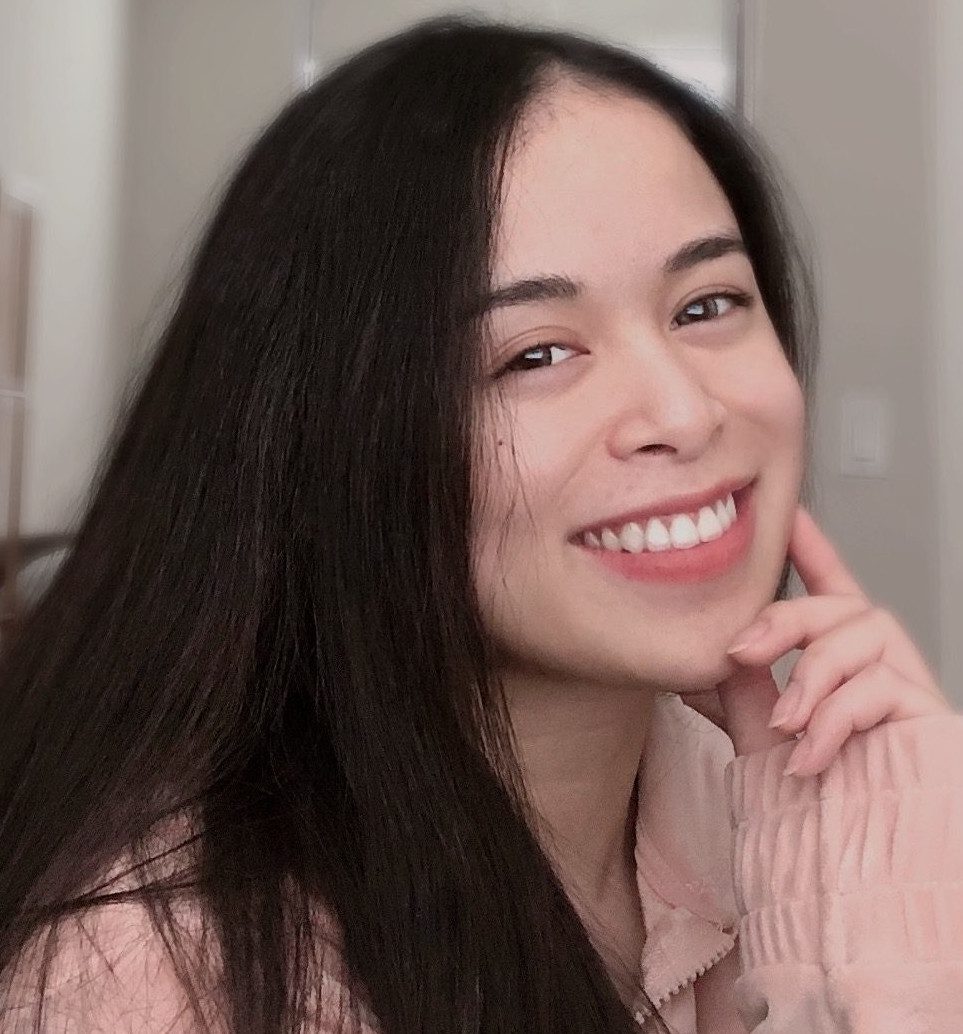
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
To learn more about coding in Python, read our complete guide on how to learn Python.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.