Often, when you’re working with data, you’ll want to store a list of data that cannot contain any duplicate values, and whose values cannot be changed. For instance, if you’re making a list of purchase IDs at a grocery store, you would want each ID to be unique, and you would not want anyone to be able to change the values in your list.
That’s where the Python set object comes in. A set is an unordered collection of items that can only contain unique values.
This tutorial will discuss, with reference to examples, the basics of Python sets, and how you can perform common operations like adding and removing values on a set.
Python Sets
The Python built-in set data structure is similar to the idea of sets in mathematics.
Python sets can contain zero or more elements, and there is no defined order for each element in the set. Every element stored within a set is unique, so no duplicates can exist, and each individual value in the set cannot be changed.
Although individual values in a set cannot be changed, the set itself can be. This means that we can add or remove items from our set.
The set structure is useful because it comes with a number of mathematical operations that we can use to work with the data we have stored in a set.
Create a Python Set
In Python, sets are defined as a list of items (or “elements”) that are separated by commas. The list is then enclosed within curly braces ({}).
Python sets can store any number of items, and each item within a set can use different data types. So, a set can store both an integer or a string, or a string, float, and a tuple. However, sets cannot store mutable data types, like another set, a dictionary, or a list.
Suppose we want to create a set that stores a list of homework assignments a student has handed in for an English class. We could create this set using the following code:
assignments = {"Worksheet #4", "Worksheet #5", "Worksheet #7", "End of Topic Review #2"} print(assignments)
Our code returns:
{‘Worksheet #5’, ‘Worksheet #4’, ‘Worksheet #7’, ‘End of Topic Review #2’}
In our code, we defined a set called assignments
which has four values. Then, we printed out the contents of the assignment set to the console. You can see that our values are returned in a random order because sets do not store their items in any particular order.
Changing a Set
When you have created a set, you cannot change the values of the items in the set. However, you can add items to and remove items from a set.
Add Items to a Set
To add an element to a Python set, you can use the add()
method. Suppose a student has just handed in Worksheet #8
and we want to update our set to keep track of the student’s assignment being handed in. We could do so using this code:
assignments = {"Worksheet #4", "Worksheet #5", "Worksheet #7", "End of Topic Review #2"} assignments.add("Worksheet #8") print(assignments)
Our code returns:
{‘Worksheet #8’, ‘Worksheet #4’, ‘Worksheet #5’, ‘End of Topic Review #2’, ‘Worksheet #7’}
As you can see, Worksheet #8
has been added to our set.
In addition, if you want to add multiple items to a set, you can use the update()
method. The update()
method accepts a list of items to which you want to add to a set as a parameter, then adds those items to a set.
Suppose a student has handed in both worksheets #8 and #9. We could change our set to reflect these new assignments being handed in using this code:
assignments = {"Worksheet #4", "Worksheet #5", "Worksheet #7", "End of Topic Review #2"} assignments.update(["Worksheet #8", "Worksheet #9"]) print(assignments)
Our code returns:
{‘Worksheet #9’, ‘End of Topic Review #2’, ‘Worksheet #8’, ‘Worksheet #7’, ‘Worksheet #4’, ‘Worksheet #5’}
Now our set contains two new values: Worksheet #8
and Worksheet #9
.
Remove Elements from a Set
To remove an element from a set, you can use discard()
or remove()
.
The discard()
function removes an item from a set and returns nothing if an item does not exist in a set. The remove()
function will attempt to remove an item from a set, and if that item does not exist, an error will be raised.
Suppose we want to remove Worksheet #9
from our record of a student’s homework assignments because they have been asked to re-do the assignment. We could do so using this code:
assignments = {'Worksheet #9', 'End of Topic Review #2', 'Worksheet #8', 'Worksheet #7', 'Worksheet #4', 'Worksheet #5'} assignments.discard("Worksheet #9") print(assignments)
Our code returns:
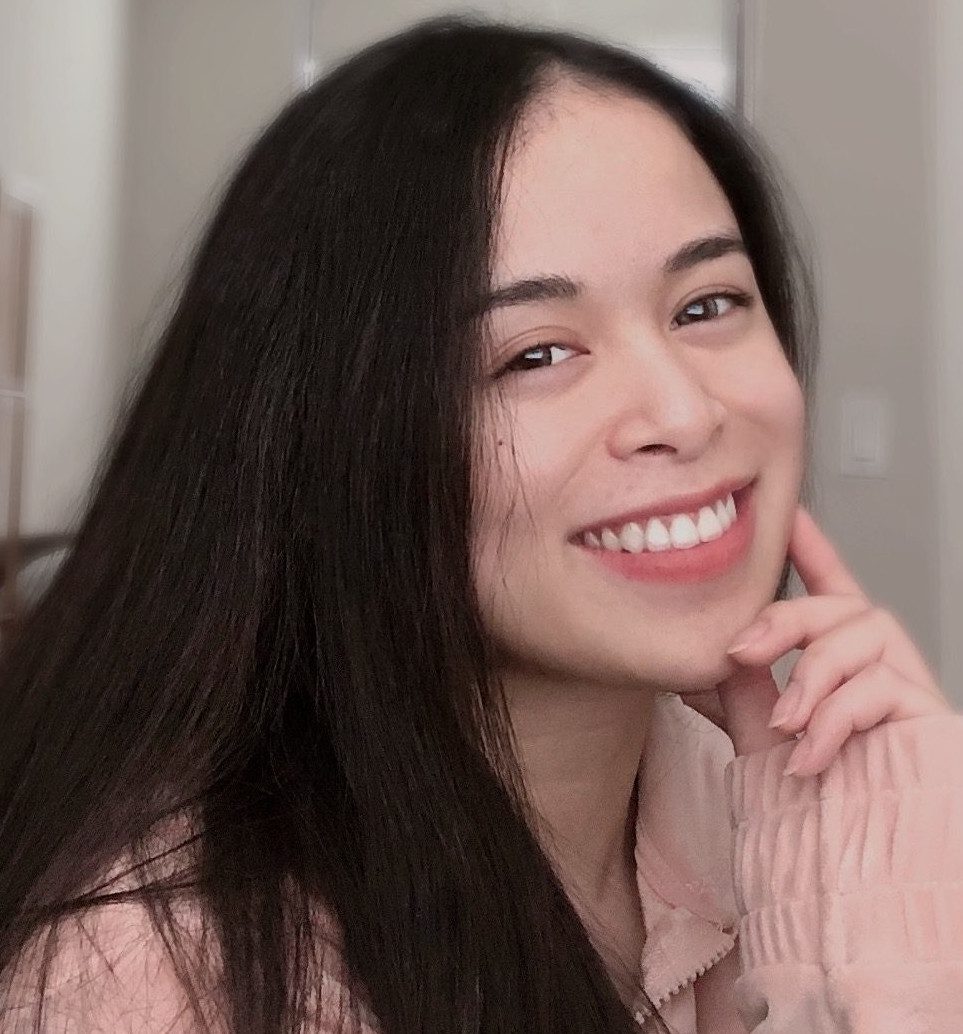
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
{‘End of Topic Review #2’, ‘Worksheet #5’, ‘Worksheet #4’, ‘Worksheet #8’, ‘Worksheet #7’}
You can see that the value Worksheet #9
has been removed from our set. We accomplished this using the discard()
method.
Clear a Set
To clear a set, you can use the clear()
method. Suppose we want to erase a record of a student’s assignments because it is the end of the year and we no longer need to store the information. We could do so using this code:
assignments = {'Worksheet #9', 'End of Topic Review #2', 'Worksheet #8', 'Worksheet #7', 'Worksheet #4', 'Worksheet #5'} assignments.clear() print(assignments)
Our code returns: {}. This reflects our empty set.
Return Length of a Set
To find out how many items are stored in a set, you can use the len()
method.
Suppose we want to find out how many homework assignments a student has handed in. We could do so using this code:
assignments = {'Worksheet #9', 'End of Topic Review #2', 'Worksheet #8', 'Worksheet #7', 'Worksheet #4', 'Worksheet #5'} print(len(assignments))
Our code returns: 6. In our code, we first declare a set called assignments
. Then, we use the len()
method to find out how many items are stored in the assignments
set.
Python Set Operations
One of the advantages of using a set to store data is that sets support mathematical set operations. Let’s walk through how three of the main set operations work.
These set operations do not change the contents of a set. Instead, they perform a calculation on an existing set.
Set Union
The union()
method allows us to create a set of all elements from two sets.
Suppose we have two lists of a student’s assignments that we want to merge together. One list contains data on a student’s homework, and the other contains a list of the essay assignments a student has completed. We could merge these lists using this code:
assignments = {"Worksheet #4", "Worksheet #5", "Worksheet #7"} essays = {"Essay #1", "Essay #2"} homework = assignments.union(essays) print(homework)
Our code returns:
{‘Worksheet #7’, ‘Essay #2’, ‘Worksheet #4’, ‘Essay #1’, ‘Worksheet #5’}
In our code, we use the union()
method to merge our two sets together. We assign the value of the newly-merged set to the variable homework
. Then, we printed out the value of the homework
variable.
Alternatively, we could have used the following code:
assignments = {"Worksheet #4", "Worksheet #5", "Worksheet #7"} essays = {"Essay #1", "Essay #2"} print(assignments | essays)
The “|” operator performs a union set operation. Our code returns a merged set:
{‘Essay #2’, ‘Worksheet #4’, ‘Worksheet #5’, ‘Essay #1’, ‘Worksheet #7’}
Set Intersection
The intersection()
method allows you to generate a list of elements that are common between two sets.
Suppose we have a list of essays a student has handed in, and a list of essays that have been issued. If we wanted to know which essays a student has not yet handed in, we could perform an intersection method.
Here’s the code we would use:
essays_handed_in = {"Essay #1", "Essay #2"} essays_issued = {"Essay #1", "Essay #2", "Essay #3"} due = essays_handed_in.intersection(essays_issued) print(due)
Our code returns:
{‘Essay #1’, ‘Essay #2’}
In this example, we use the intersection()
method to find all elements that exist in both the essays_handed_in
and essays_issued
sets. We assign the result of the intersection operation to the variable due
, which we then print to the console.
In addition, we can use the &
operator to perform a set intersection operation. So, if we wanted to perform an intersection on our two sets from earlier, we could use this code:
essays_handed_in = {"Essay #1", "Essay #2"} essays_issued = {"Essay #1", "Essay #2", "Essay #3"} print(essays_handed_in & essays_issued)
Our code returns:
{‘Essay #1’, ‘Essay #2’}
Set Difference
The difference()
method allows you to find out which elements are present in one set that are not present in another set.
Suppose we wanted to generate a list of all essays that a student has handed in and that have not yet been graded. We could do so using this code:
essays_handed_in = {"Essay #1", "Essay #2"} essays_graded = {"Essay #1"} final = essays_handed_in.difference(essays_graded) print(final)
Our code returns:
{“Essay #2”}
In this code, we used the difference()
method to find the difference between the essays_handed_in
and essays_graded
sets. Alternatively, we could use the -
operator, like so:
essays_handed_in = {"Essay #1", "Essay #2"} essays_graded = {"Essay #1"} print(essays_handed_in - essays_graded)
Our code returns:
{“Essay #2”}
The same result is returned in our code. But instead of using the difference()
set method, we used the -
operator, which performs a check to find the difference between two sets.
Conclusion
The Python set data structure allows you to store unordered collections of items.
Each item in a set must be unique, so no duplicates can exist, and the contents of a set cannot be changed once they are added to the set.
This tutorial discussed, with reference to examples, the basics of Python sets and the main operations offered by sets.
We only scratched the surface of what you can do with sets, though—there’s still more for you to explore—but we did cover the main skills you need to know to effectively work with sets. Now you’re ready to start using the Python set()
method like a pro!
Python is used in professional development environments around the world for everything from data analysis to web development. Download the free Career Karma app today to learn more about career paths in Python programming.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.