How to Send an Email Using Python
Python is a language of many features. It can be used for data analysis, web development, and more. That’s not all, Python has a hidden feature: you can use it to send emails. This means that you can send password reset emails, forgot password emails, user notifications, and whatever other emails you want to send, from a Python program.
In this guide, we’re going to discuss how to send an email using Python. We’ll walk through the email and smtplib libraries, how they work, and write an example program to send an email.
Without further ado, let’s get started!
Sending Emails Using Python
When you send an email from a computer program, your program will send the message using a protocol called Simple Mail Transfer Protocol (SMTP). This protocol is used by email services and clients around the world to send messages.
To send an email from a computer program, you’ll need to have an SMTP server. You can set one up by yourself, but you don’t always have to do this. Services like Gmail and Outlook provide SMTP services so you can use your existing email accounts to send an email.
For this guide, we’re going to assume that you are sending an email from Gmail’s SMTP server. You can find out more about their SMTP server in the Gmail official documentation. To find out whether your email provider supports SMTP, search online for ‘[name of your provider] SMTP credentials’.
We’ve got three steps to follow to send an email:
- Configure our SMTP connection
- Create a message object
- Send the message over SMTP
Configuring an SMTP Connection
To start, let’s set up our SMTP connection. We can do this using a library called smtplib which provides all the code we need to manage the connection. Thanks to this library it only takes a few lines of code to send an email.
Start by importing the smtplib library into your code:
import smtplib
We’ve now got to set up variables which store the credentials for our SMTP server. Storing these values in variables will help us maintain the readability of our code. Here are the variables we’re going to use:
sender = 'test@careerkarma.com' password = '123456' server = 'smtp.gmail.com' port = 465
This code contains all the configuration we need to create an SMTP connection. Now that we have this set up, we can log into our SMTP server:
server = smtplib.SMTP_SSL(server, port) server.login(sender, password)
Our code creates an SSL connection to our SMTP server. This means that we are using Secure Socket Layer (SSL) to connect to our server. SSL is more secure than a traditional connection and as a result it has become a standard on SMTP servers.
Our SMTP connection is now configured!
Create a Message Object
Our code doesn’t do very much right now: it definitely does not send an email. That’s because we have not yet created a message object. Let’s do this by using the email library. While you can use smtplib to create a message object, the email library is more concise.
Let’s start by importing the necessary email packages:
from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText
With these libraries imported, we can create our message object:
message = MIMEMultipart() body = 'This is an email sent from Python!' message['From'] = sender message['To'] = 'test@careerkarma.com' message['Subject'] = 'This is a test email' message.attach(MIMEText(body, 'plain'))
We’ve started by initializing an object called ‘message’. This object references the MIMEMultipart class from the email library. We’ve then specified the body of our email and the sender, recipient, and subject.
Our final line of code attaches the body of our message to our email.
Send the Message
Now all that’s left to do is send our message:
server.send_message(message)
When we run all of our code together, an email is sent! An email with the title ‘This is a test email’ is sent from test@careerkarma.com to test@careerkarma.com.
Reading a Template from a File
The body of our email is only one line long. This means that it’s more practical for us to write our email body inside Python. Most emails are longer than this, and so it’s better to create a template which stores the text for a particular email.
We’ll start by creating a file called email.txt and pasting in the following contents:
Hello ${NAME}, This is a test email! Thanks, Career Karma
This template contains a variable called “NAME”, which is enclosed within curly braces ({}) and is preceded by a dollar sign ($). This variable will be substituted with the name of the recipient later in our code.
We’re now going to have to read this template into our code. We can do this by creating a class called read_email which uses the open() method to read our file.
We will import the Template object from the string library. We’ll use this object to create an object that can be read by our email. Paste in the following line of code at the top of your Python program:
from string import Template
Below all of your import statements, paste in this code:
def read_email(): with open('email.txt', 'r') as file: contents = file.read() return Template(contents)
This function will read the file called “email.txt” into the variable “contents”. Its value is then converted into a Template object using the Template method from the “string” library. You can learn more about reading files in our Python read file tutorial.
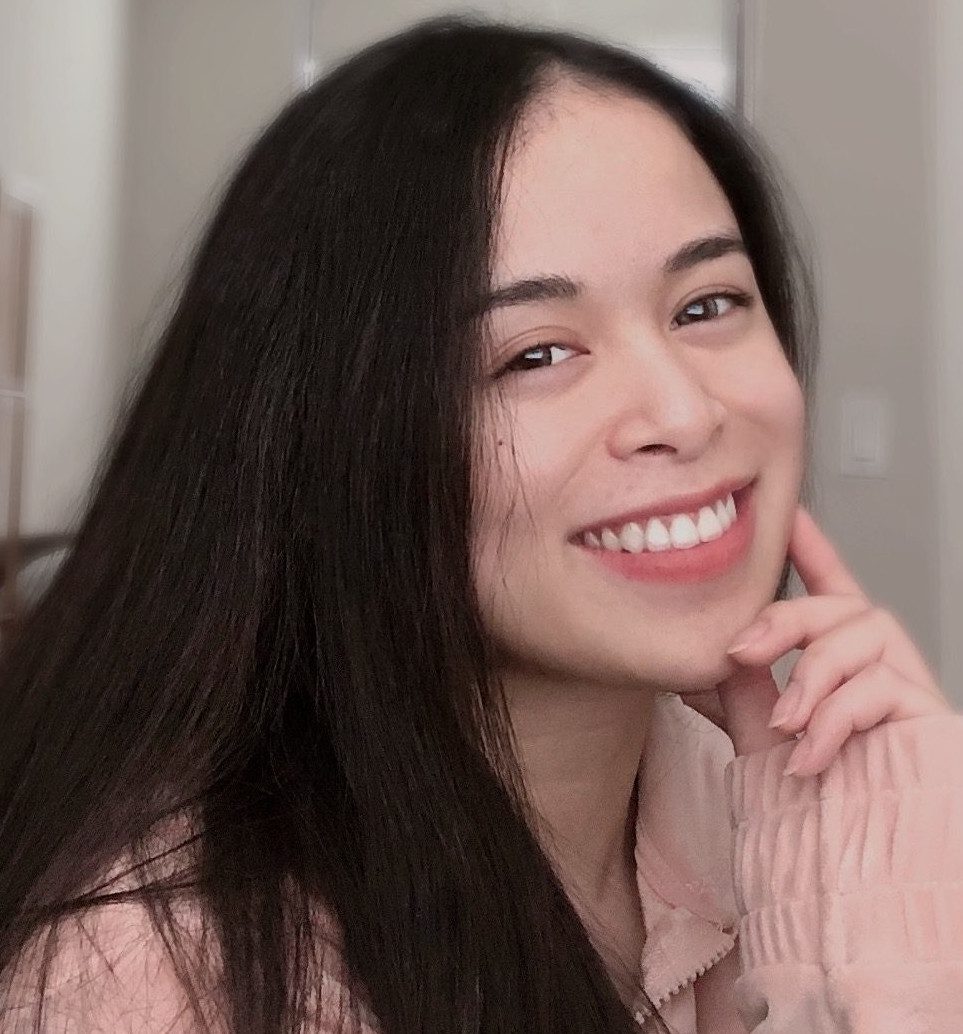
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Change the following line of code in your program:
body = 'This is an email sent from Python!'
To use this code:
email_content = read_email() body = email_content.substitute(NAME="Test")
This code calls the read_email()
function in our code to read the contents of the “email.txt” file. Then, the value of NAME inside our email template is substituted with the value “Test”. Together, our code looks like this:
import smtplib from email.mime.multipart import MIMEMultipart from email.mime.text import MIMEText from string import Template def read_email(): with open('email.txt', 'r') as file: contents = file.read() return Template(contents) sender = 'test@careerkarma.com' password = '123456" server = 'smtp.gmail.com' port = 465 server = smtplib.SMTP_SSL(server, port) server.login(sender, password) message = MIMEMultipart() email_content = read_email() body = email_content.substitute(NAME="Test") message['From'] = sender message['To'] = 'test@careerkarma.com' message['Subject'] = 'This is a test email' message.attach(MIMEText(body, 'plain'))
When you run this program, substituting in your SMTP server credentials, an email will be sent. You’ve just written a program to send an email.
Conclusion
Sending an email in Python doesn’t have to be difficult. Without the use of a template, sending an email only takes a few lines of code. The email and smtplib modules do most of the heavy-lifting. With that said, you can use the email module more extensively to create templates for your emails that can substitute values.
Are you up for a challenge? Change the program from above to support sending emails to multiple recipients. Hint: You’ll want to use a for loop to send these emails.
Now you’re ready to start sending emails in Python like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.