The Python round() method rounds a number to a specified number of decimal places. By default, round() rounds a number to zero decimal places. round() is useful if you want to work with numbers with a certain precision.
You may encounter a scenario where you want to round a number to a certain decimal place.
You can use the round() function in Python to do this. For example, you may be running a butcher shop and want to round the prices of your products to the nearest dollar.
In this tutorial, we will explore how to round numbers using the round() function in Python. We’ll also go through a few examples to illustrate how this function would work in an actual program.
Python round() Method
The Python round() method rounds a number to a specific decimal place. This method returns a floating-point number rounded to your specifications. By default, the round() method rounds a number to zero decimal places.
Let’s take a look at the syntax for the round() built-in function:
round(number_to_round, decimal_places)
round() accepts two arguments:
- The value you want to round.
- The number of decimal points to which the value should be rounded (optional, default zero decimal places).
round() returns a floating-point because the method can return decimal values. Thus, you should plan to accept a float instead of an integer number. This is important to consider if you decide to perform math on the result of the round() method.
Python does have two methods that let you round up and down. The floor() method rounds down to the nearest integer. ceil() rounds up to the nearest integer. We’ll focus on the round() method in this guide.
You can read more about ceil() and floor() in our Python guide to ceil() and floor().
One of the most common uses of rounding numbers is when you’re working with money. This is because many people prefer not to work with fractions of pennies and decimal fractions when working with money.
Rounding numbers is also common when you’re working with mathematical calculations that return unrounded values. You’ll find rounding values comes up a lot in data science and machine learning for this reason.
round() Python: Example
Let’s use an example to illustrate how this function works. Say that we manage a produce stand and are looking to calculate how we should price individual oranges.
We buy oranges in bundles of fifty but sell single oranges to customers. So, we want to divide the price of fifty oranges by fifty to get the wholesale price of an individual orange.
Here’s an example Python script we could use to accomplish this task:
fifty_oranges = 15.52 individual_orange = fifty_oranges / 50 print(individual_orange) rounded_value = round(individual_orange, 2) print(rounded_value)
Our code returns a Python floating-point number followed by a rounded number:
0.3104 0.31
First, we declared the price of fifty oranges. We set this value as a Python variable, whose value is $15.52 in this case. Then, we calculated the price of an individual orange by dividing the price of fifty oranges by fifty.
However, the price of an individual orange turned out to be $0.3104, which is not a price that we can charge consumers. So, we used the round() function to return the price of an individual orange rounded to the nearest penny.
Our program returned the rounded number 0.31, which tells us that we want to charge more than $0.31 per orange to make a profit.
In our example, we specified 2 in our round() method, which tells our program to round our number to two decimal places. However, if we wanted the round() function to return the nearest integer, we would not need to specify this parameter.
Python Round to The Nearest Whole Number
To round to the nearest whole number in Python, you can use the round() method. You should not specify a number of decimal places to which your number should be rounded.
Here’s an example of using round() without the second parameter to round a number to its nearest whole value:
number = 24.28959 print(round(number))
Our Python code returns: 24.
The round() function is usually used with floating-point numbers, which include decimal places. You can also pass integers into round() without returning an error. With that said, our program will return the same values because integers are already round numbers.
Optionally, you can specify 0 as a second argument instead of omitting it entirely. This will also round a value to the nearest whole number. But since the 0 is the default value, we did not specify it in this example.
The round() function can also be used to round a negative number. Here’s an example of this in action:
print(round(-102.22))
Our code returns: -102.
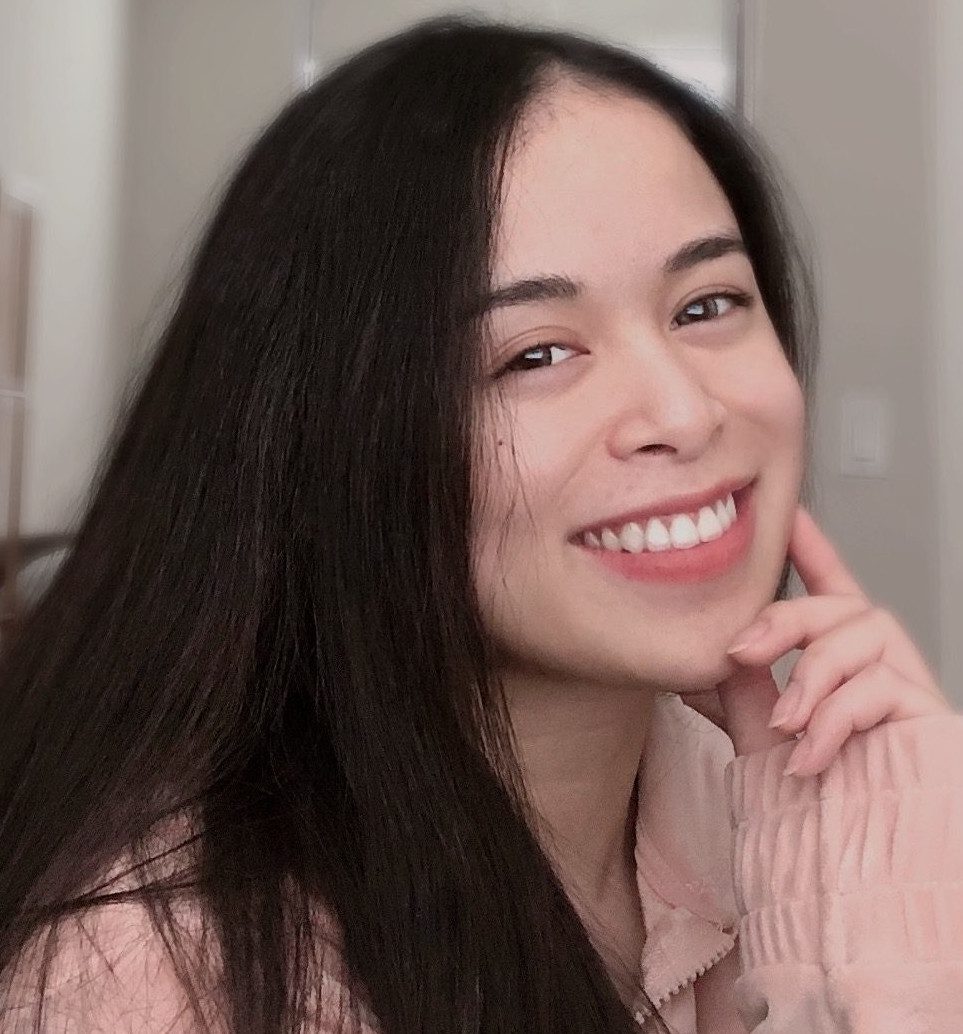
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
You can use the Python round() function to round a number to a certain decimal place. This can be helpful if you’re working with mathematical calculations that return numbers with unnecessary decimal places. Or, round() can be helpful if you are working with values like money which typically do not show more than two decimal places.
In this tutorial, we discussed how to use the round() function to round numbers in Python. We then explored a few examples using the round() function to show how it works. Now you’re ready to start rounding numbers in Python like an expert!
To learn more about Python programming, read our complete How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.