You can reverse a string in Python using slicing or the reversed() method. A recursive function that reads each character in a string and reverses the entire string is another common way to reverse a string. There is no function explicitly designed to reverse a string.
When you’re working in Python, you may have a string that you want to reverse. For instance, say you are creating a game. You may want to allow users to generate a username by submitting their name to the program and having the program reverse it.
String reversal is not a common operation in programming, so Python does not have a built-in function to reverse strings. However, reversing strings is a topic that comes up in job interviews, and it does have a few real-world applications.
In this tutorial, we will discuss three methods you can use to reverse a string n Python. We’ll start with a Python string refresher. Following that, we’ll discuss string slicing. Then, we’ll explore how to use the reversed() and join() functions. Finally, we’ll look at recursion.
How to Reverse a String in Python
There are three methods you can use to reverse a string:
- Slicing the string with the [::-1] syntax
- Using the reversed() function to create a reverse iterator that reads a string and reverses its contents
- Using a recursive function
Let’s discuss all three of these methods.
Python Reverse String Using Slicing
When you’re slicing a Python string, you can use the [::-1] slicing sequence to create a reversed copy of the string. This syntax retrieves all the characters in a string and reverses them.
Suppose we have a string with the value “Python”. We want to see this string in reverse order. We could do so using the [::-1] syntax:
string = "Python" reversed = string[::-1] print(reversed)
Our code returns: nohtyP.
We declare a Python variable called reverse which stores our reversed string. The [::-1] syntax is added at the end of the variable that contains our “Python” string value.
This is an effective method to use because it is short and simple. You only need to add [::-1] to the end of a string to reverse it.
This method can appear confusing because the syntax does not clearly convey that it can be used to reverse strings. As a result, many developers avoid using this technique.
Reverse String Python Using reversed()
Python includes a built-in function that is used to create a reverse iterator: the reversed() function. This iterator works because strings are indexed, so each value in a string can be accessed individually.
The reverse iterator is then used to iterate through the elements in a string in reverse order.
Here’s an example that utilizes reversed() to create a reverse iterator that prints out our string in reverse order:
for char in reversed("Python"): print(char)
Our code returns:
n o h t y P
In our code, we specify a Python for loop that iterates through each item in the reversed() method. You can see that our code has printed out our string in reverse order.
Our code printed each character on a new line. This is because we used reversed() with a for loop. If we wanted our string to appear on a single line, we would need to use reversed() with the join() method.
We can use the join() method to merge all the characters from our reversed() iterator into one string.
Here’s an example that uses reversed() and join() to reverse a Python string:
"".join(reversed("Python"))
Our code returns: nohtyP.
This method of reversing strings is useful because, like the slicing method discussed above, it is compact and concise. This method has the added benefit of being easy to read and understand. Even if you hadn’t seen the reversed() or join() methods before, you would be able to guess what was going on.
Reverse a String Python: Recursion
Recursion is a term used in computer science to describe a function that calls itself, either directly or indirectly. Here’s an example of reversing a string via Python recursion:
def reverse_string(string): if len(string) == 0: return string else: return reverse_string(string[1:]) + string[0] reverse_string("Python")
Our code returns: nohtyP.
This method uses slicing to reverse our string. Instead of using a single slice operation like we did earlier, we used a Python function called reverse_string.
This function slices our string and returns a reversed version. This method is very effective. As a result, many Python developers favor it.
Our function accepts the string we want to reverse. If there are no characters in the string, the value of the string is returned. Otherwise, we call the function again using the string[1:] syntax as an argument. We add the first character in our string to the end of what this function returns.
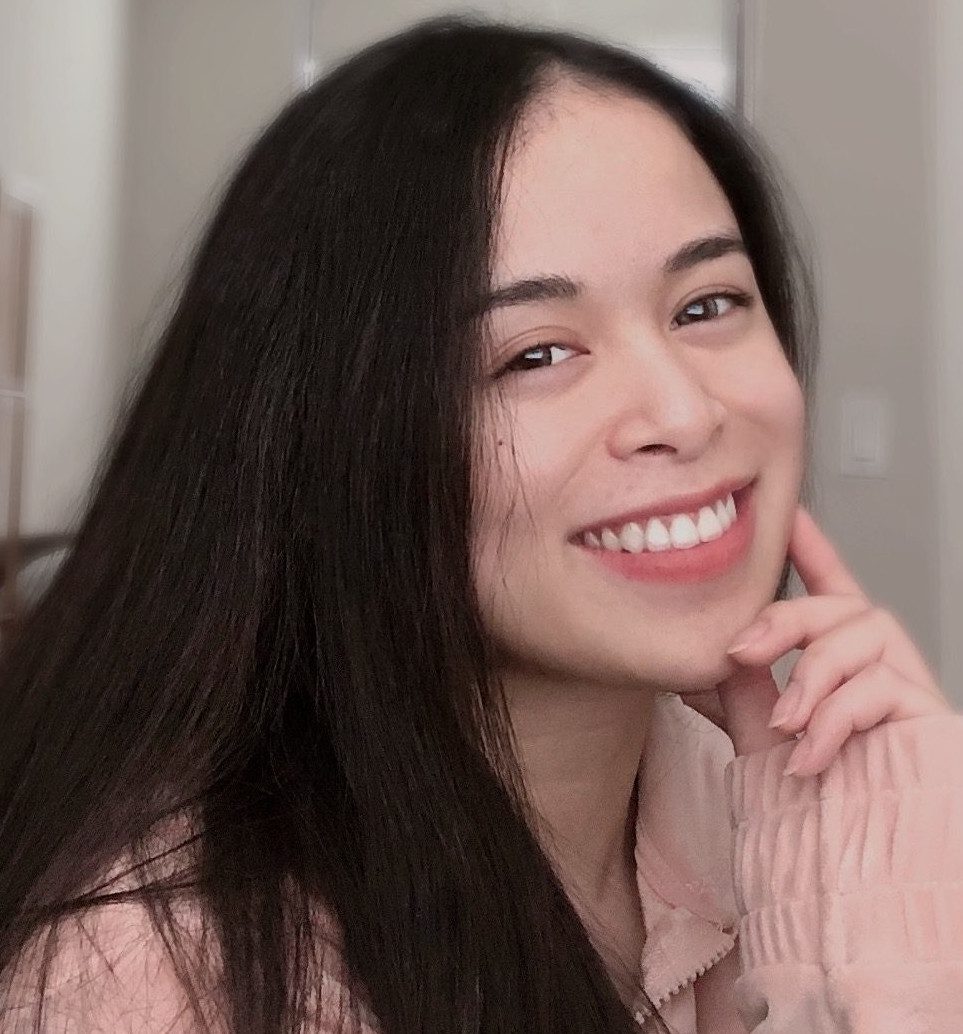
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
While Python does not include a built-in reverse function, there are a few approaches we can use to reverse a string.
We can use the [::-1] slicing method to reverse a string through slicing, the reversed() and join() functions to reverse a string through reverse iteration, or recursion to reverse a string using a recursive function.
In this tutorial, we discussed using slicing, reversed() and join(), and recursion to reverse a string in Python. We explored an example of each of these string methods in action.
To learn more about writing Python code, read our comprehensive How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.