You can reverse a list in Python using the built-in reverse()
or reversed()
methods. These methods will reverse the list without creating a new list. Python reverse()
and reversed()
will reverse the elements in the original list object.
Reversing a list is a common part of any programming language. In this tutorial, we are going to break down the most effective Python reverse list methods.
Let’s say you have a list in Python of customer names in reverse alphabetical order but want to see them in alphabetical order. You would have to reverse the list to do so. If you have a long list and want to see what’s at the bottom, you could just scroll down. Or, you could reverse to get the data quickly.
Python Lists and List Slicing: A Refresher
In Python, lists are arrays of items. Your list could include strings, booleans, numbers, objects, or any other data type in Python, and can hold as many as you want. When working with a lot of data, it can be useful to declare a list instead of declaring multiple variables.
List Slicing
While you don’t need to know about list slicing to perform a reverse list operation, it can be helpful.
This is how you would declare a list in Python:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"]
We have just declared a variable, studentNames
, and assigned a list of values—in this case, the names of students—to the variable.
If we want to get a particular value from our list, we can use its index numbers and slice the list. That’s a lot to take in, so let’s break it down. Each item in our list has an index value, starting from 0. We can use them to get a certain value from our list. Here are the index values for our array:
Hannah | Imogen | Lewis | Peter |
0 | 1 | 2 | 3 |
If we want to get Imogen
from our list, we would do so using the following code:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"] print(studentNames[1])
We have declared our variable with student names. Next, we said we want to get the list item with the index value of 1
. In this case, the value was Imogen
.
We can also change the index number to get a range by adding another one. Here’s an example:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"] print(studentNames[1:3])
The output for our code would be as follows:
["Imogen", "Lewis", "Peter"]
Now we know the basics of list slicing, we can start to discuss reversing lists in Python.
Python Reverse List Tutorial
The three main ways that you can reverse a list in Python are:
- Reverse using slicing
- Reverse using Reverse()
- Reverse using Reversed
Reverse Using Slicing
The first is to use a slicing trick to reverse your list. Here is the Python syntax:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"] print(studentNames[::-1])
The output for our code is:
["Peter", "Lewis", "Imogen", "Hannah"]
In our code, we have declared our variable studentNames
, and then created a slice function that will reverse the list. This is a common way to slice lists. But it can be inefficient because it creates a copy of the list, which takes up memory. In addition, this function can be a little advanced in relation to other methods of reversing lists.
Reverse Using Reverse()
The second option we can take is to use the built-in function, reverse()
. The reverse()
method can be called to reverse the contents of a list object in-place. This means you won’t be creating a new list. Instead, we will modify the original list object.
The following code shows the Python syntax for reverse()
in action:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"] studentNames.reverse() print(studentNames)
The output for our code is as follows:
["Peter", "Lewis", "Imogen", "Hannah"]
It’s important to note that the reverse()
function itself returns a value of None
. So, if we want to get our revised list, we need to print it out again, as we did above. This is an efficient way of reversing a list and does not require a lot of memory, unlike our example. The syntax is also clear and simple.
Reverse Using Reversed()
The third and final approach we can use to reverse a list is to use the reversed()
function. This is a built-in function we use by creating a reverse iterator to create a reverse order of our list in Python. This means that a new list isn’t created, and our old list is not modified. Instead, the function will cycle through all of the elements in our list and return them in reverse order.
The following syntax shows the reversed()
function in action:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"] for i in reversed(studentNames): print(i)
The output for our code is:
"Peter" "Lewis" "Imogen" "Hannah"
If we really wanted to create a reversed copy of the list, we could use this code:
studentNames = ["Hannah", "Imogen", "Lewis", "Peter"] list(reversed(studentNames))
This would return:
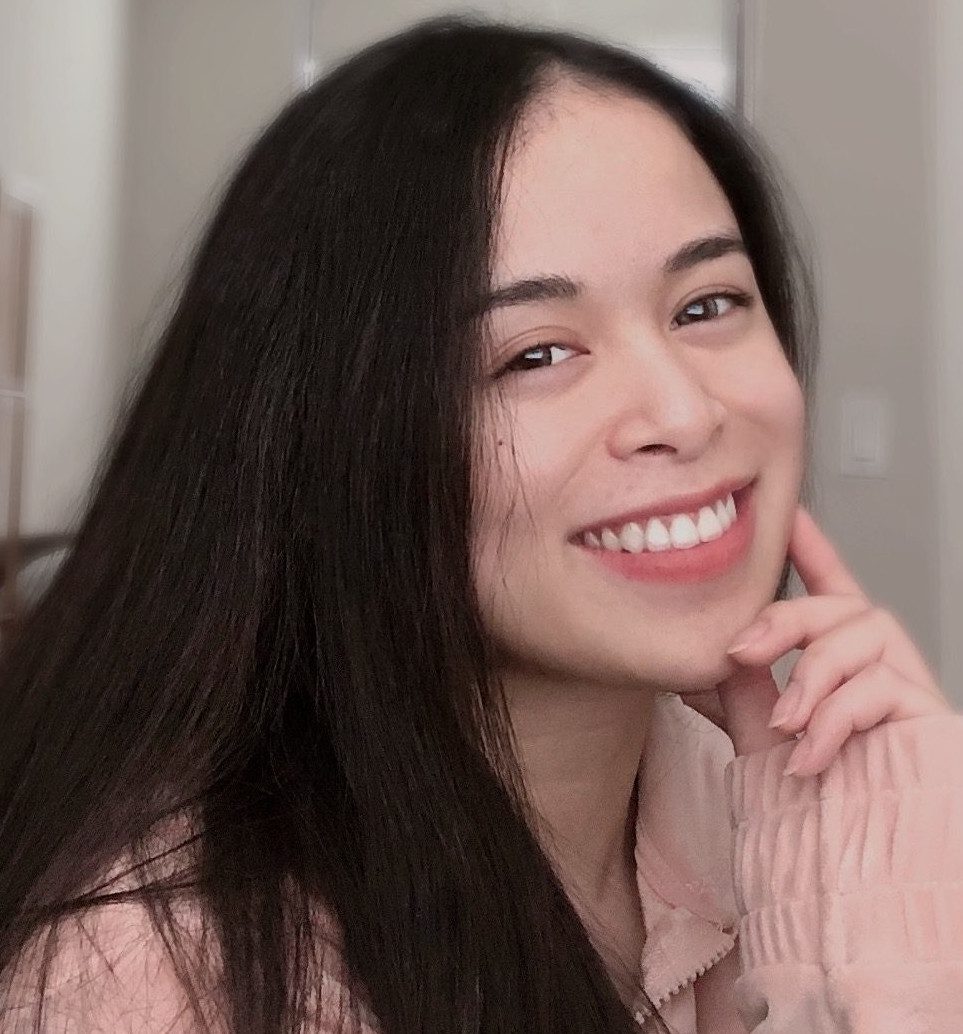
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
["Peter", "Lewis", "Imogen", "Hannah"]
This is another easy way to reverse lists. It clearly shows what is going on. A new list is being created that will include all the values in our other list, but reversed.
Conclusion
That’s how the various reverse list methods work. In this tutorial, we have explored the basics of lists, which are arrays of items such as strings or objects, and discussed index values. We have also outlined three methods you can use to reverse a list.
Of course, you only need to know one. But being aware of all three may help you if others have different approaches and use them in code you read.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.