You can return multiple values by bundling those values into a dictionary, tuple, or a list. These data types let you store multiple similar values. You can extract individual values from them in your main program. Or, you can pass multiple values and separate them with commas.
Python functions can return multiple values. To return multiple values, you can return either a dictionary, a Python tuple, or a list to your main program.
This guide discusses how to return multiple values to a main program using these two methods. Let’s begin.
Python: Return Multiple Values
You can return multiple values from a Python function using:
- A list that contains multiple values.
- A tuple with multiple values.
- A dictionary with multiple records.
- Multiple values separated by commas.
All the above data types let you store multiple values. The solution of returning multiple values separated by commas is the most elegant. This is because this approach makes your intent clear: to return multiple separate values back to your main program.
A Python return statement sends values from a function to a main program. This statement is most commonly used to return one value to a main program. It can be used to return multiple values to a main program.
Python: Return Multiple Values with Commas
You can return multiple values by separating the values you want to return with commas. These values should appear after your Python return statement.
We’re going to write a program that calculates the number of sales made at an electronics store. We’re only going to count sales worth more than $500. Our program will also calculate the average value of each sale.
To start, let’s define a Python array that contains a list of sales:
sales = [59.99, 240.00, 655.25, 75.99]
Next, write a Python function that calculates the number of sales worth more than $500 and the average value of a purchase:
def calculate_data(sales): over_limit = 0 for s in sales: if s > 500: over_limit += 1 average_purchase = sum(sales) / len(sales) return over_limit, average_purchase
Our function iterates over every sale in our list using a for loop. If a sale is worth more than $500, our “over_limit” Python variable is incremented by one. We then calculate the average value of a purchase by dividing the total value of all sales by the number of sales made.
At the end of our function, use a return statement to return the values of “over_limit” and “average_purchase” to our main program.
All that’s left to do is call our function and display the data our program calculates to the console:
over_limit, average_purchase = calculate_data(sales) print("{} sales were made over $500. The average purchase was ${}.".format(over_limit, round(average_purchase)))
We round the average purchase to two decimal places using the round() method. Our code prints out a message informing us of the values we have calculated. Let’s run our program:
1 sales were made over $500. The average purchase was $258.
Our program successfully tells us how many sales were made worth more than $500 and what the average price of a purchase was.
This code works because the “return” statement turns our values into a tuple and returns a tuple list to our main program. We then unpack this tuple into two variables on this line of code:
over_limit, average_purchase = calculate_data(sales)
Python: Return Multiple Values with a List or Tuple
Both lists and tuples let you store multiple values. This means we can use them to return multiple values to our main program.
Using a list or a tuple is best if the values you are returning have some kind of relationship. But, you can use this approach to return any collection of values.
We’re going to return the average purchase size and the number of purchases worth over $500 to our main program. To do so, let’s refer back to our example from earlier and make a small change to use a list:
def calculate_data(sales): over_limit = 0 for s in sales: if s > 500: over_limit += 1 average_purchase = sum(sales) / len(sales) return [over_limit, average_purchase]
We have just separated our values into a single list. Our list contains the number of purchases worth over $500 and the value of an average purchase, respectively.
We could also return a tuple data structure. To do so, we would replace our square brackets with curly brackets. The big factor to consider is that tuples cannot contain duplicate values. If there is a chance two values you pass to your main program will be equal, avoid using a tuple.
To access these values in our main program, we have to use indexing:
values = calculate_data(sales) print("{} sales were made over $500. The average purchase was ${}.".format(values[0], round(values[1])))
We use the values[0] syntax to access the first value in the list our calculate_data() function returns. This corresponds to the value of “over_limit” in our function. We use the values[1] syntax to access the value of the average purchase.
Our code returns:
1 sales were made over $500. The average purchase was $258.
Our code works just like our last example, but this time we’ve used a list to separate the values in our code.
Python: Return Multiple Values with a Dictionary
We can return multiple values from a function using a dictionary.
In our last example, we only returned two values to our main program. This made it convenient to use unpacking syntax (where you “unpack” values into multiple variables).
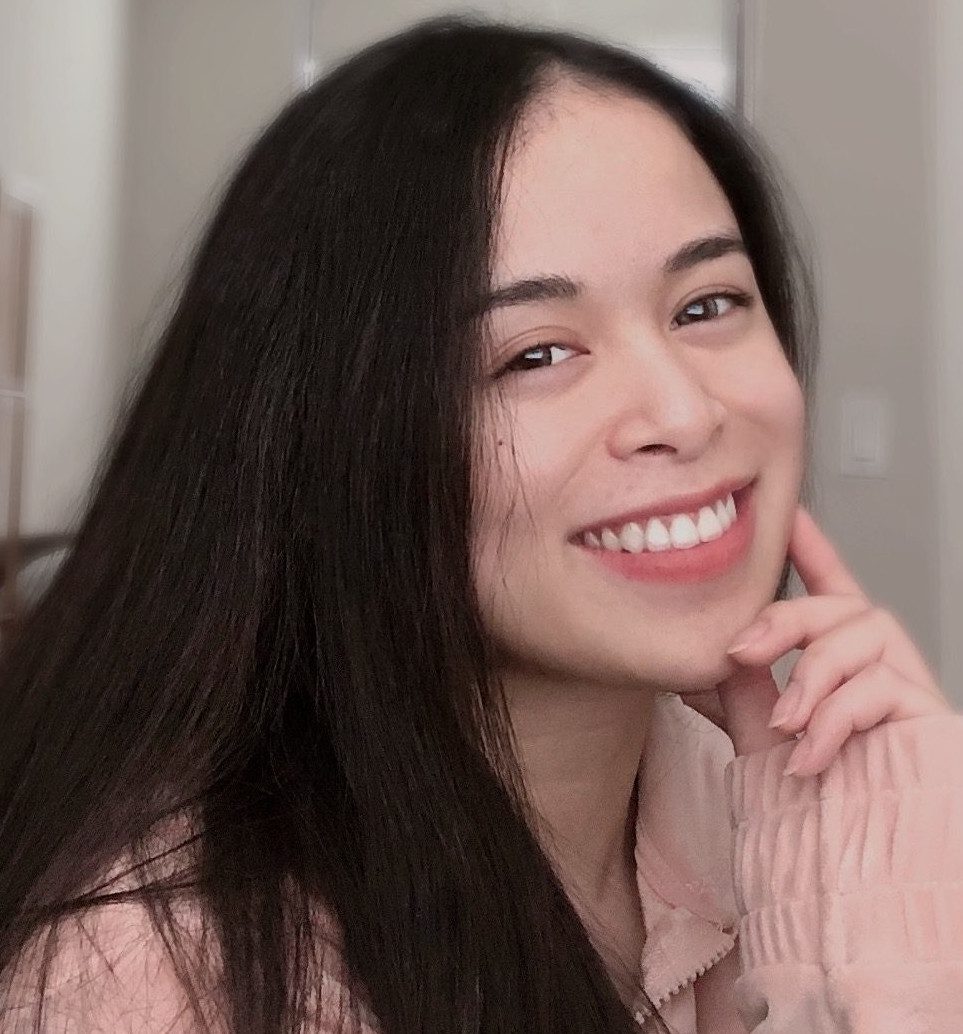
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
If we were working with more values, it may be easier to return a dictionary that associates each value with a label. Let’s revise our last function to use a dictionary to return multiple values to our main program:
def calculate_data(sales): over_limit = 0 for s in sales: if s > 500: over_limit += 1 average_purchase = sum(sales) / len(sales) return { "limit": over_limit, "average": average_purchase }
Our function returns a dictionary with two keys and values. The keys, “limit” and “average”, can be used to uniquely identify each value. Now we have to revise our function call and our print statement to support this new syntax:
values = calculate_data(sales) print("{} sales were made over $500. The average purchase was ${}.".format(values["limit"], round(values["average"])))
We return a dictionary back to our main program. We assign this dictionary to the variable “values”. Then, we use indexing to retrieve the values of “limit” and “average”.
Let’s run our program:
1 sales were made over $500. The average purchase was $258.
Our program returns the same response as earlier. This is expected because all we have done is changed the way in which we return values to our main program. Our calculation logic remains the same.
Conclusion
You can return multiple values from a function using either a dictionary, a tuple, or a list. These data types all let you store multiple values. There is no specific syntax for returning multiple values, but these methods act as a good substitute.
The comma-separated method is more concise and easier to understand if you are working with a small set of values. Say you are returning a larger range of values from a function. You may want to use a dictionary to send multiple values back to the main program.
Do you want to accelerate your journey learning the Python programming language? Check out our How to Learn Python guide for advice on top Python courses and online learning resources.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.