The Python return statement instructs Python to continue executing a main program. You can use the return statement to send a value back to the main program, such as a string or a list.
Functions are blocks of instructions that perform a specific task in programming. Once a function has been declared, it can be reused multiple times. The main benefit of using functions is that it can reduce the number of lines of code you write.
When you’re working with functions in Python, you will encounter the return statement. This statement stops a function from executing and returns values from a function to the main program.
In this tutorial, we are going to discuss how the return statement works and using return to send values to a main program.
Python Function Refresher
Python includes a number of built-in functions—such as print(), str(), and len()—which perform specific tasks.
You can declare your own Python function using the def keyword. After using the def keyword, you should state the name of your function. Then, you should specify by a set of parentheses. The parenthesis can hold any parameters that you want your function to accept.
Here’s the syntax for declaring a function in Python:
def example_function(): yourcodehere
Python Return
The Python return keyword exits a function and instructs Python to continue executing the main program. The return keyword can send a value back to the main program. A value could be a string, a tuple, or any other object.
This is useful because it allows us to process data within a function. Then, we can return the data to our main program, so we can use it outside our function.
Data processed within a function is only accessible within the function. This is only the case unless you use the return statement to send the data elsewhere in the program.
Here’s the syntax for the return keyword:
def example_function(): return "Example"
The return keyword can appear anywhere within a function, and when the code executes the return statement, the function will be exited.
The return statement returns a value to the rest of the program, but it can also be used alone. If no expression is defined after the return statement, None is returned. Here’s an example of this in action:
def example_function(): return example_function()
Our code returns: None.
You may decide not to specify a value if you only want a function to stop executing after a particular point. This is a less common use case of the Python return statement.
Return Python: An Example
Say that we are operating a restaurant. We want to create a program that returns the cost of the sandwich meals we have on the menu. This task should be contained within a function because we intend to use it more than once.
The waitress should enter the name of the sandwich a customer has ordered, and be presented with the price of the sandwich. We could accomplish this task using the following code:
sandwich = "Ham and Cheese" def calculate_price(product): if product == "Ham and Cheese": return 2.50 elif product == "Tuna and Cucumber": return 2.25 elif product == "Egg Mayo": return 2.25 elif product == "Chicken Club": return 2.75 else: return 2.50 calculate_price(sandwich)
Let’s run our program and see what happens:
2.50
On the first line of code, we declare the name of the sandwich whose price we want to find out. In this case, our sandwich Python variable stores the value Ham and Cheese.
Next, we declare a function called calculate_price() which accepts one parameter: the name of the sandwich the customer would like to purchase. We have used Python if statements to check the name of the product against our list of sandwiches. When the product name is found, our program returns the price it has defined.
Here are the potential Python floating-point values our program can return:
- “Ham and Cheese”: 2.50
- “Chicken Club”: 2.75
- Another sandwich: 2.50
On the final line, we call our calculate_price() method and pass the sandwich variable as an argument. When our calculate_price() method is called, our program executes the method and returns the price for the sandwich we have specified.
In this case, the sandwich our customer has ordered is Ham and Cheese, so our program returns 2.50.
As soon as the return statement is executed, our function stops and our program continues to run.
Return in Python: Another Example
Let’s use another example of the return statement to illustrate how it works. Say that we are creating a program that calculates the change a customer is due. We could accomplish this task using the following code:
price = 2.50 def calculate_change(amount_given): return amount_given - price calculate_change(4.00)
Our code returns:
1.50
We declared a function called calculate_change that subtracts the price of the sandwich from the amount given by the customer. In this case, the price of the sandwich is $2.50, and the user has given the waitress $4.00. So, our program returns $1.50.
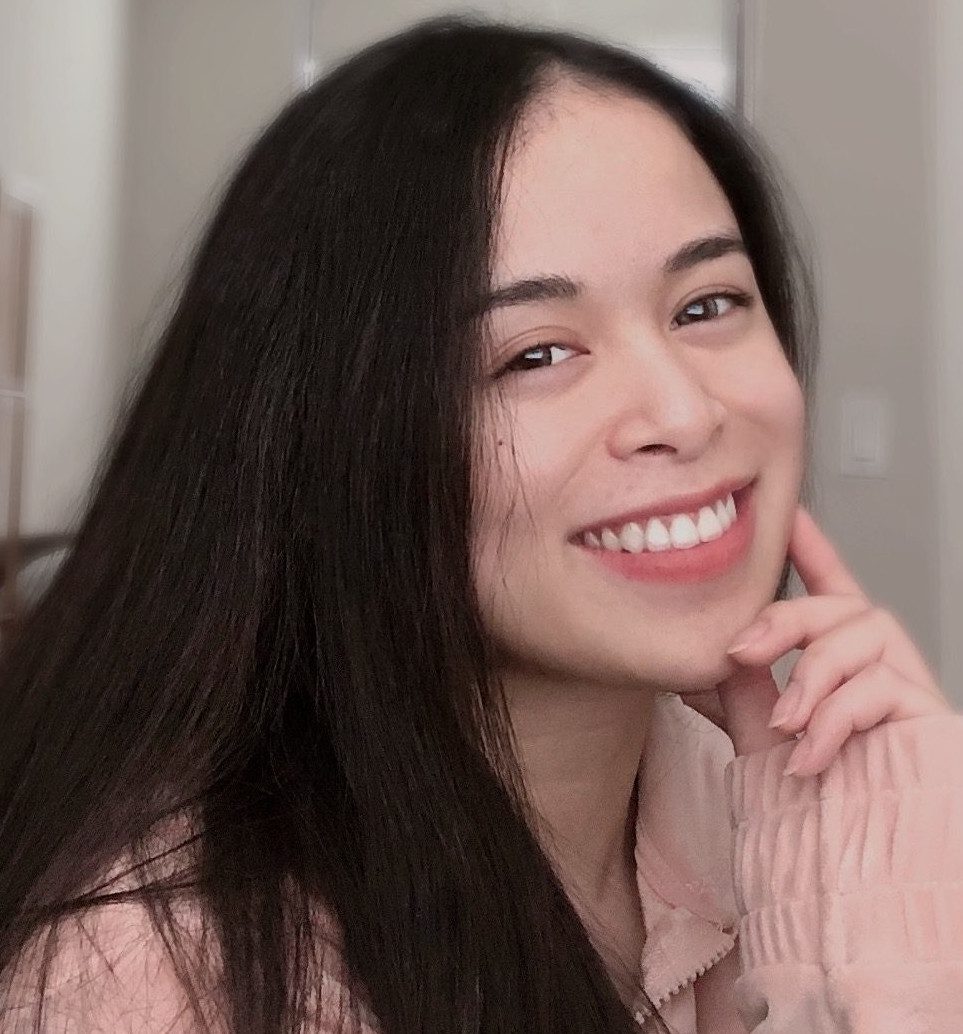
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
The return keyword in Python exits a function and tells Python to run the rest of the main program. A return keyword can send a value back to the main program.
While values may have been defined in a function, you can send them back to your main program and read them throughout your code.
In this tutorial, we delved into the basics of the Python return statement. We also walked through two examples of Python functions that utilized the return keyword to illustrate how the statement works.
For courses, learning resources, and more information about Python, check out our complete How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.