The Python os.rename() method changes the name of a file. os.rename() accepts two arguments: the path of the old file and the path of the new file. The new file path should end in a different file name.
When you’re working with files in Python, you may decide that you want to rename a particular file. For instance, if you have a file called raw_data.csv, you may want to rename it old_data.csv when your program runs.
That’s where the os.rename() method comes in. The os.rename() method allows you to rename an existing file in Python.
This tutorial will discuss, with examples, the basics of the os.rename() method, and how you can use it to rename files in Python.
Python Rename File
The Python os.rename() method renames a file. The file you are renaming should already exist. You need to specify the path of the file you are renaming as well as the new path for the file. The new path should have a different name to the file you are renaming.
The syntax for os.rename() is as follows:
os.rename(file, destination)
As you can see, rename() accepts two parameters. These are:
- file: The path of the file you want to rename, followed by the file name (e.g. “/home/career_karma/file.txt”).
- destination: The path of the file, followed by the new file name (e.g. “/home/career_karma/file_new.txt”).
The os.rename() method is part of the Python os library. This library provides functions that relate to your computer’s operating system, such as creating and deleting files.
To work with the os.rename() method, we need to import the os library:
import os
Let’s walk through an example to show the os.rename() method in action.
Python Rename File Example
Suppose we want to rename the file raw_data.csv to old_data.csv. The file raw_data.csv is stored in the directory /home/career_karma. We could change the name of our file using this code:
import os
old_file_name = "/home/career_karma/raw_data.csv"
new_file_name = "/home/career_karma/old_data.csv"
os.rename(old_file_name, new_file_name)
print("File renamed!")
Our code returns: File renamed! Our code has also renamed our file.
First, we import the os module. This allows us to access the os.rename() method.
Then, we declare two Python variables. The first variable (“old_file_name”) contains the path of the file we want to rename, and the second variable (“new_file_name”) contains the new path name for the file.
Because we want to change our file name to old_data.csv, our new_file_name variable ends in old_data.csv, instead of raw_data.csv.
Next, we use os.rename() to change the name of our file. Then, our code prints “File renamed!” to the console, so we know our program has executed.
Python Rename Multiple Files
Now, suppose we want to rename multiple files. We can also accomplish this goal using the os.rename() method.
Let’s say that we want to rename every file in the /home/career_karma directory and add old_ to the start of each file name. Right now, this directory contains the following files:
- data.csv
- raw_data.csv
- program.py
We could use the following program to add old_ to the start of each filename:
import os
for file in os.listdir("/home/career_karma"):
os.rename(file, f"/home/career_karma/old_{file}")
Our code renames our files. Here is a list of the new files in our directory:
- old_data.csv
- old_raw_data.csv
- old_program.py
Let’s break down how our code works. First, we import os into our code, so that we can work with the os.rename() and os.listdir() methods. Then, we create a for loop that iterates through a list of all files in the /home/career_karma folder. This list is generated using the os.listdir() method.
Then, our code uses os.rename() to rename each file in the /home/career_karma folder. We use a Python f string to replace each file name with /home/career_karma/old_{FILE_NAME}, where FILE_NAME is the name of our old file.
As you can see, old_ has been added to the start of every file in the /home/career_karma folder.
Conclusion
The os.rename() method allows you to rename files in Python. When used with the os.listdir() method, you can use os.rename() to rename all the files in a folder.
This tutorial discussed, with examples, the basics of the os module and how to use the os.rename() method. Now you’re ready to start using os.rename() to rename files like a Python professional!
Do you want to learn more about coding in Python? Check out our How to Learn Python guide. You’ll find top tips on how to learn Python as well as a list of expert-curated learning resources to help you on your journey.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
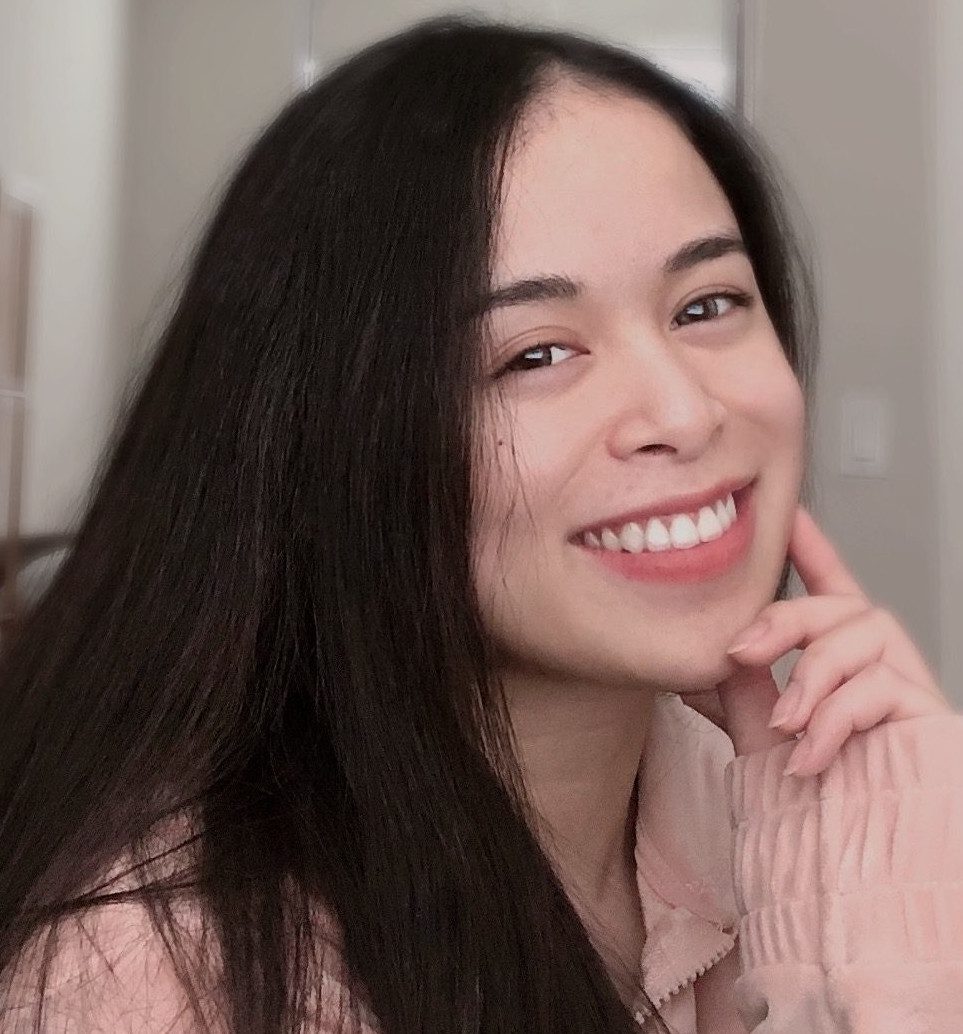
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot