The Python random.choice() method returns an element selected at random from a list. random.choice() works on a list with items in any data type. random.choice() accepts one argument: the list from which you want to select an item.
You may encounter a scenario where you want to choose an item randomly from a list. For instance, say you’re building a color guessing game. You may want the color the user is guessing to be chosen at random from a list of potential options.
To implement a random choice selector in Python, you can use the random.choice() and random.choices() function. These functions allow you to retrieve a single random item and multiple random items from a sequence of items, respectively.
This tutorial will discuss, with examples, the basics of the Python random library. We’ll talk about how to use the random.choice() method to retrieve a random element from a list.
Python Random Choice Module
The Python random module allows you to access functions related to random numbers.
One of the most common uses of this module is to generate a random number, which you can do by using the randint() function. The module also provides a function called choice(), which allows you to choose, at random, an item from a list.
In addition, random also provides a function called choices(), which allows you to return multiple random choices from a list.
Here are a few examples of applications which would use the random library:
- A tool that picks a random number.
- A tool that picks a random color from a list of colors.
- A tool that chooses a random card from a deck.
- An application that flips a coin.
We need to import the random library into our code before we can start using the functions in the library. We can do so using the following Python import statement:
import random
Python random.choice()
The Python random.choice() function returns a random element from a sequence. You must specify the list from which you want to retrieve a random element as an argument. random.choice() returns one value.
The syntax for this function is as follows:
random.choice(sequence)
The random.choice() method can return an item of any data type. So, random.choice() could return a JSON object from a list, or a number.
Let’s walk through an example to illustrate how the random.choice() method works.
random.choice() Python Example
Suppose we are building an application that chooses a song at random from our list of favorite songs. This application will help us overcome the dreaded “what should I listen to?” question that comes up when choosing a song.
To build this application, we will start with a list of our favorite songs:
favorite_songs = ["Ring of Fire", "9 to 5", "Come on Eileen", "Everybody Wants to Rule the World"]
This list contains four of our favorite songs. Then, we can use random.choice() to select a random song from this list. Here’s the code we would use:
import random favorite_songs = ["Ring of Fire", "9 to 5", "Come on Eileen", "Everybody Wants to Rule the World"] song_to_play = random.choice(favorite_songs) print("The song you should play is:", song_to_play)
To illustrate how this program works, we will execute it three times. Here is the response from all three of the occasions on which we executed our program:
The song you should play is: Ring of Fire The song you should play is: Come on Eileen The song you should play is: Ring of Fire
The random.choice() method has allowed us to choose a random item from our list of favorite songs.
Let’s break down our code. First, we imported the random module, which includes the choice() function that we use later in our program. Then, we declared a list of our favorite songs from which our program should choose one.
Next, we used the choice() method to select a random item from our list of favorite songs. We assigned that choice to the variable “song_to_play”. Finally, we printed to the console “The song you should play is:”, followed by the randomly-selected song we should play.
Random Choice Python: Using random.choices()
So far, we’ve selected one random choice from a list using the random.choice() method. But what if we want to select multiple random choices?
To do so, we can use the random.choices() method. This method allows you to return one or more random choices from a list. The syntax for the choices() method is:
random.choices(sequence, weights, accumulate, k=number_to_choose)
The choices() method accepts the following parameters:
- sequence: The sequence from which you want to choose a random item. This can be a string, list, or a tuple.
- weights: A list for you to weigh the likelihood each value appears.
- accumulate: A list with weights for each value. The possibility of each weight accumulates.
- number_to_choose: The number of random options you want to select from your sequence.
We’re going to focus on the “sequence” and “number_to_choose” arguments in our examples.
Python random.choices() Example
Suppose we want to select three songs at random from our list of favorite songs to play. These will all be added to our new playlist of top songs.
The following program allows us to accomplish this task:
import random favorite_songs = ["Ring of Fire", "9 to 5", "Come on Eileen", "Everybody Wants to Rule the World"] songs_to_play = random.choices(favorite_songs, k=3) for s in songs_to_play: print(s)
Our code returns:
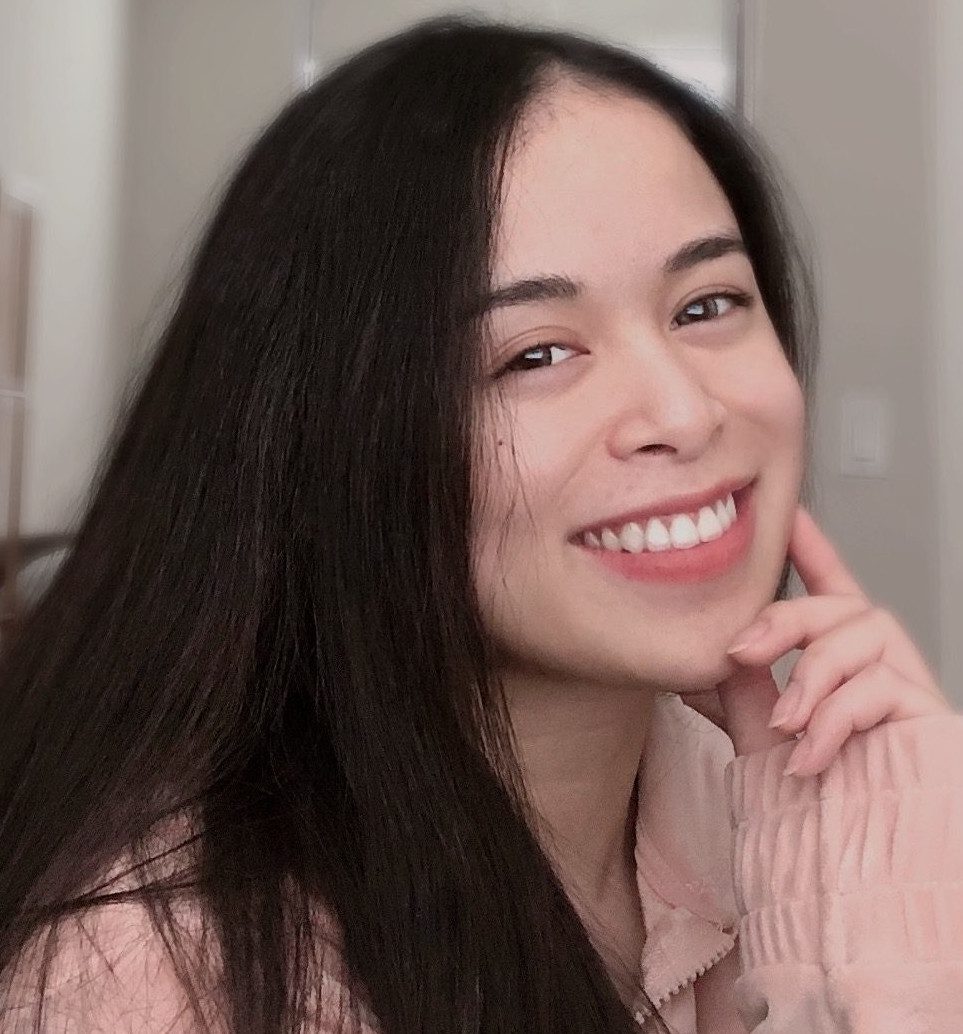
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Come on Eileen 9 to 5 Ring of Fire
First, we have imported the random library into our code. Then, we declared a Python variable called “favorite_songs”. This variable stores a list of our favorite songs.
Next, we used the random.choices() method to select three songs at random from our list. The random.choices() method returned a list of songs.
We used a Python for loop to go through each item in the list of randomly-generated song options. This loop prints out each option to the console. As you can see, our code returned a list of three songs that we can play, at random.
Conclusion
The random.choice() method selects an item at random from a list. random.choices() method selects multiple items at random from a list. Both of these methods can return a value of any data type, such as another list or a number.
This tutorial explored, with reference to examples, how to use these methods to retrieve random items from a list. Now you’re ready to start using these methods like a Python pro!
For advice on top online Python resources, books, and learning resources, check out our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.