Dictionaries store data in key-value pairs. This means each value in a dictionary is associated with a key. This key acts as a reference point.
If you want to view the contents of a dictionary on the console, there are a few approaches you can take. You can print out a dictionary directly, or print out the key-value pairs individually.
In this guide, we discuss how to print a dictionary in Python. We walk through a few examples to help you figure out how to print a dictionary in your own code.
Python: Print Dictionary Using a for Loop
We’re going to build a program that prints out the contents of a dictionary for a baker to read. This dictionary contains the names of ingredients and the quantities of those ingredients that are needed to bake a batch of scones.
Let’s create a dictionary with information about our recipe:
scone = { "self-raising flour": "350g", "baking powder": "1 tbsp", "butter": "85g", "caster sugar": "3 tbsp", "milk": "175ml", "egg": "1 whole" }
Our dictionary contains six keys and values. Each key represents the name of an ingredient and each value tells us the quantity of that ingredient needed to cook a batch of scones.
Next, we use a for loop to print the contents of this dictionary. We can do this using the items()
method like this:
for key, value in scone.items(): print(value, key)
Let’s run our code and see what happens:
350g self-raising flour 1 tbsp baking powder 85g butter 3 tbsp caster sugar 175ml milk 1 whole egg
Our code successfully prints out all of the keys and values in our dictionary. The items()
method returns two lists: all the keys in a dictionary and all the values in a dictionary. Every time we iterate over the method, we can access a new key-value pair.
In the first iteration, “value” is “350g” and “key” is “self-raising flour”. Our for loop continues to iterate until every key-value pair has been printed to the console.
Python: Print Dictionary Using the json Module
In our last example, we printed out a dictionary to the console manually using a for loop. This is ideal for the last use case because we wanted the list of ingredients to be readable by a baker.
The json module lets you work with dictionaries. The json.dumps method makes a dictionary easily readable by developers. This is ideal for developers who want to see the values in a dictionary on the console.
We’re going to tweak our program from earlier to use the json module to print a dictionary.
To start, import the json module so that we can work with it in our code:
import json
Next, let’s define our dictionary:
scone = { "self-raising flour": "350g", "baking powder": "1 tbsp", "butter": "85g", "caster sugar": "3 tbsp", "milk": "175ml", "egg": "1 whole" }
This dictionary is the same as the one in our last example. The next step is to use the json module to print out our dictionary to the console. We’re going to use a method called json.dumps to format our dictionary:
formatted = json.dumps(scone, indent=4) print(formatted)
We specify two parameters when we call the json.dumps()
method: the name of the dictionary we want to format and how many spaces should constitute each indent. In this example, each indent will be four spaces long.
Next, use a print()
statement to view the formatted dictionary. Let’s execute the program so we can see our dictionary:
{ "self-raising flour": "350g", "baking powder": "1 tbsp", "butter": "85g", "caster sugar": "3 tbsp", "milk": "175ml", "egg": "1 whole" }
Our code shows us our list of ingredients.
Python: Print a Nested Dictionary
“Nested dictionary” is another way of saying “a dictionary in a dictionary”.
You can print out a nested dictionary using the json.dumps()
method and a print()
statement, or you can use a for loop. The for loop method is similar to our earlier example but we’ll need to change our code a little bit.
Suppose that the ingredients in a dictionary within a dictionary:
recipes = { "scone": { "self-raising flour": "350g", "baking powder": "1 tbsp", "butter": "85g", "caster sugar": "3 tbsp", "milk": "175ml", "egg": "1 whole" } }
To access the items in our “scone” dictionary, we need to first reference the “scone” key. To print out the dictionary to the console, we use two for loops:
for key, value in recipes.items(): print(key) for k, v in value.items(): print(k, v)
The first for loop iterates over our “recipes” dictionary. The second for loop iterates over each dictionary in our “recipes” dictionary, Let’s run our code:
scone self-raising flour 350g baking powder 1 tbsp butter 85g caster sugar 3 tbsp milk 175ml egg 1 whole
Our code successfully prints out the contents of our “recipes” dictionary and the contents of the “scone” dictionary.
Conclusion
You can print a dictionary in Python using either for loops or the json module. The for loop approach is best if you want to display the contents of a dictionary to a console whereas the json module approach is more appropriate for developer use cases.
You can use both of these methods to print a nested dictionary to the console.
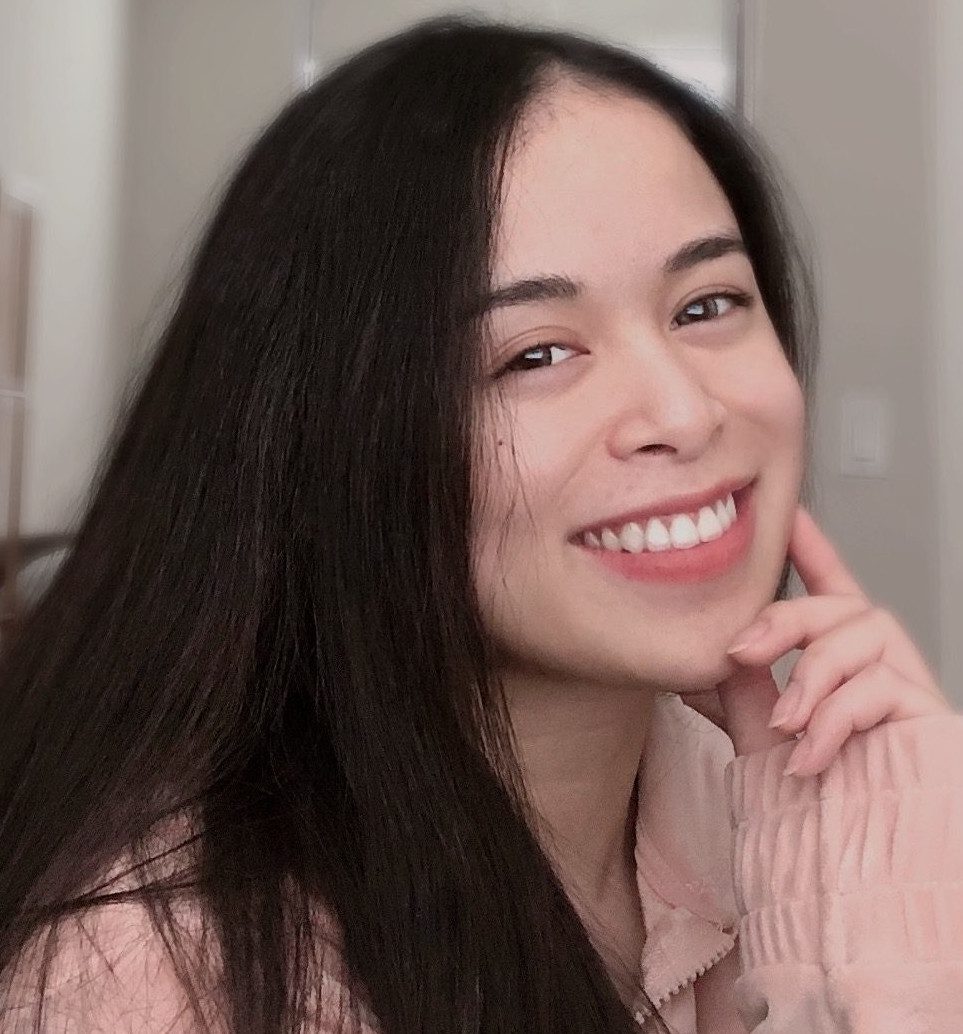
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Now you’re ready to print a dictionary to the Python console like an expert developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.