The Python pass statement is a placeholder keyword. It is used in empty code blocks such as a for or if statement to prevent against a syntax error. Python recognizes the section of code in which a pass statement is written as blank.
You may encounter a situation where you want to include a placeholder in a class, function, or procedure. For instance, say you are writing a large program and want to include a for loop. This loop does not yet function because there is other code you need to write first.
That’s where the Python pass statement comes in. The pass statement is used as a placeholder for the future implementation of functions, classes, loops, and other blocks of code.
This tutorial will discuss how to use the pass statement in Python. We’ll walk through a few examples of the pass statement being used in a Python program.
Python pass Statement
The Python pass statement tells Python that a section of code is blank but should not return a syntax error. The Python interpreter reads and processes the pass statement. But, the pass keyword does not disrupt the flow of your program.
Here’s the syntax for the pass statement:
pass
The pass statement takes in no arguments or variables. pass is a standalone keyword, similar to the Python return keyword.
There are a few scenarios where using the pass statement can be helpful.
While there are no specific guidelines around how pass statements should be used, the statement is required syntactically to:
- Define empty classes and functions.
- Pass over code within a try except block.
- Pass over code in a loop.
None of these code blocks can contain no code. If you specify no code in any of these blocks, Python will return a syntax error.
pass Python Examples
Let’s look at a few examples of the Python pass statement.
Bank Account Class Example
Say you’re working on a program that stores bank account information for savings and checking accounts. We define the structure of the bank account information in Python classes.
Before you start working on the savings account class, you want to focus on the checking accounts class.
To remind yourself to come back to the savings account class later, you could add a placeholder in the savings account class.
Let’s add a pass statement to the savings account class. The pass statement is a placeholder while you finish working on the checking accounts class. Then, you can remove the placeholder and fill in the class with your code. Here’s the placeholder in action:
class CheckingAccount(name): def __init__(self, name): self.name = name class SavingsAccount: pass
When our code runs, both the CheckingAccount and SavingsAccount classes are created. However, the SavingsAccount class has no purpose yet. The pass keyword is used to serve as a placeholder until we get around to adding code to execute in our class.
Bookstore for Loop Example
Let’s look at an example of the pass statement being used to pass over code in a loop. Say we are a bookstore owner, and we want to print out every title in our list of habit formation books to the console. But, we don’t want to print out Atomic Habits, which is out of stock. We could use the following code to accomplish this task:
books = ['The Power of Habit', 'Atomic Habits', 'Hooked: A Guide to Building Habit Forming Products', 'Mini Habits', 'The Miracle Morning'] for i in range(0, len(books)): if books[i] == 'Atomic Habits': pass else: print(books[i]) print('Program complete.')
Our code returns:
The Power of Habit Hooked: A Guide to Building Habit Forming Products Mini Habits The Miracle Morning Program complete.
On the first line of code, we declare a Python array. This array contains the books in the habit formation category of our store.
We then declare a Python for loop which loops through every book in the books array.
If the title of the book is Atomic Habits, our code should skip over it. Otherwise, our program should print out the title of the book to the console. Then, once our loop has finished running, our program prints out a message stating “Program complete.” to the console.
pass acts as a placeholder. This keyword allows us to skip over printing the name of the book Atomic Habits to the console.
If we did not include a pass statement, our code would look like this:
... for i in range(0, len(books)): if books[i] == 'Atomic Habits': else: print(books[i]) …
When we run this code, Python returns an error because if statements cannot be empty. The error we receive is as follows:
IndentationError: expected an indented block
Perhaps, later on, we intend on adding additional code here. For instance, we may want to print a message saying that the book is out of stock. Or perhaps we end up leaving the code as it is.
Conclusion
The Python pass statement is a placeholder that indicates you intend to add code to a section of your program later on. The pass statement syntax is: pass. You can use the pass statement in loops, if statements, or classes.
This tutorial discussed how to use the pass statement in Python to include placeholder statements. We also talked about why a pass statement may be used.
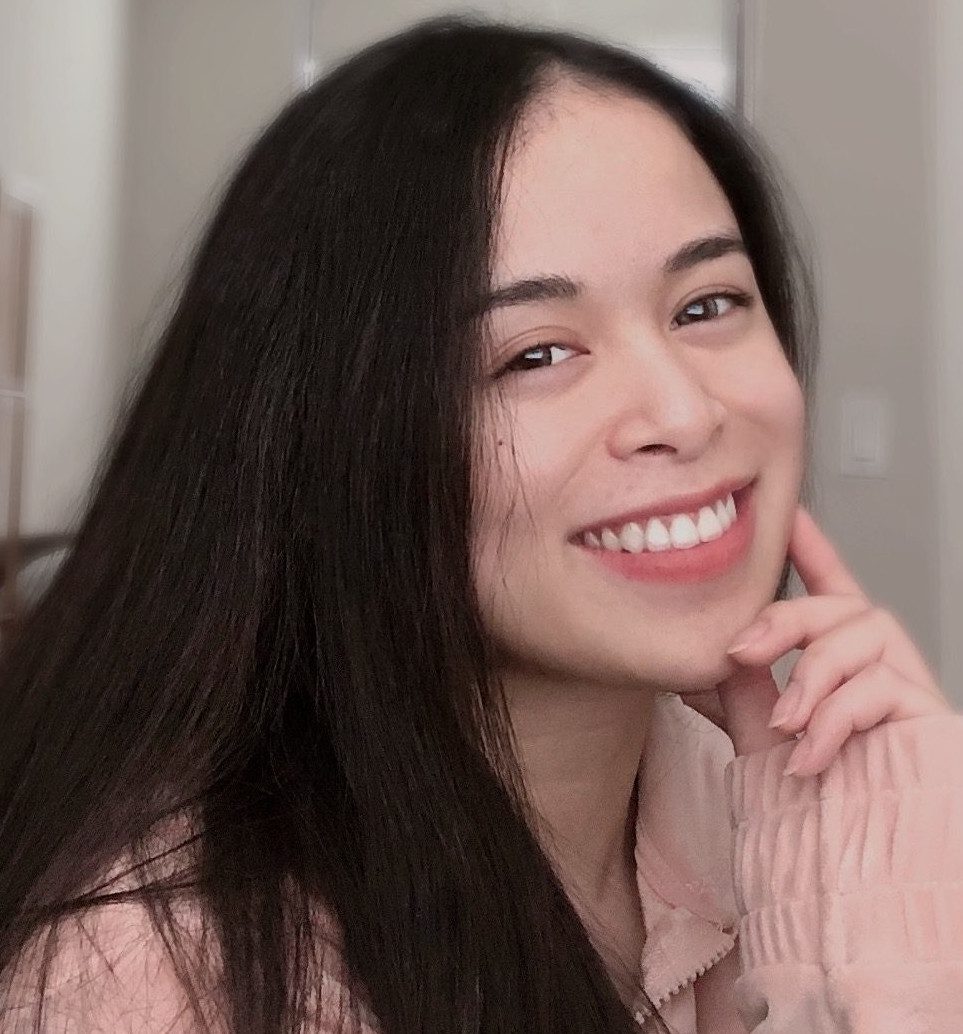
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Are you passionate about learning how to code in Python? We have a How to Learn Python guide that we wrote to help beginner and intermediate developers start or continue their learning. Check out the guide on our How to Learn Python page.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.