Some numbers need to begin with a zero or multiple zeros. For instance, a user ID may need to have a certain number of zeros at the start if it is under a certain number so all ID numbers are the same length.
You can pad a string with zeros using the zfill() function. You can pad a number with zeros using string formatting. This guide walks you through an example of how to pad zeros to a string and an integer.
Python: Pad Zeros to a String
Write a program that verifies whether a raffle ticket number is a winner. Each raffle ticket number contains three numbers like this:
022
Our program will ask a user to insert a number. If the number a user inserts is less than 100, a zero will be added to the start of the number. This will ensure our program can compare the number a user inserts to the winning ticket numbers.
Start by defining a list of winning ticket numbers:
winners = ["033", "087", "183", "173", "012"]
Each ticket number is formatted as a string. Next, ask the user to insert a number using the input() method:
to_check = input("Insert a number that you want to check: ")
The number the user inserts is the one that you’ll try to find in the list of winners. This value is formatted as a string.
A user may not insert a number that is three characters long. To make sure that the raffle number that a user inserts into the program is correctly formatted, use the zfill()
method. This method adds zeros to the start of a number.
Because the raffle ticket numbers are three characters long, you’re going to use the value 3 as a parameter with the zfill() method:
to_check = to_check.zfill(3)
This method will make sure the value of “to_check” is correctly formatted. The value 3 reflects how many numbers should be in “to_check”. It does not reflect how many zeros should be padded to the value.
Next, use an “if” statement with the “in” operator to check if the value of “to_check” appears in the list of winning raffle ticket numbers:
if to_check in winners: print("Ticket #{} is a winner.".format(to_check)) else: print("Ticket #{} is not a winner.".format(to_check))
If the ticket corresponds to a winning number, the if
statement runs; otherwise, the else
statement runs. Execute the code and see what happens:
Insert a number that you want to check: 33 Ticket #033 is a winner.
Our code has successfully identified that ticket #033 is a winner. See what happens if the number you insert into the program does not correspond to a winning ticket:
Insert a number that you want to check: 182 Ticket #182 is not a winner.
The program informs you that the ticket is not a winner.
Python: Pad Zeros to a Number
Note: The below example only works in Python 2.7 and in later versions of Python.
In the first example, you padded zeros to a value that was stored as a string. If you want to pad zeros to a number, there is another approach to use.
Suppose you are generating a list of employee identifier codes. These are used to identify each employee who works for a business. Each ID number should start with three numbers and end in the surname of an employee.
To start, ask a user to insert the numbers that should appear at the start of an employee ID. You’ll also ask the user for the name of an employee:
id_numbers = int(input("Enter the ID number of the employee: ")) surname = input("Enter the surname of the employee: ")
Next, format these values into a string. Use the .format() method to do this:
print("The employee identifier for {} is {:03d}{}.".format(surname, id_numbers, surname))
The curly braces represent values to be substituted in your string.
The first set of curly brackets will be replaced with the surname of an employee. The second set of curly braces will be replaced with the value of “id_numbers”. If the number a user inserts is less than three numbers long, zeros will be added to this value.
The last set of curly braces represents the final part of an employee identifier which is their surname. Let’s run our program:
Enter the ID number of the employee: 823 Enter the surname of the employee: SMITH The employee identifier for SMITH is 823SMITH.
Our program works. In this case, you’ve inserted an ID number that is three digits long. Let’s see what happens if you try to insert an ID number that is only two digits long:
Enter the ID number of the employee: 78 Enter the surname of the employee: JONES The employee identifier for JONES is 078JONES.
Our program still generates an identifier in the format described. Each identifier contains three numbers followed by the surname of an employee.
Conclusion
You can use the zfill()
method to pad zeros to the front of a Python string. You can use the .format()
method to pad zeros to an integer value.
Now you’re ready to add zeros to the start of value in Python like an expert coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
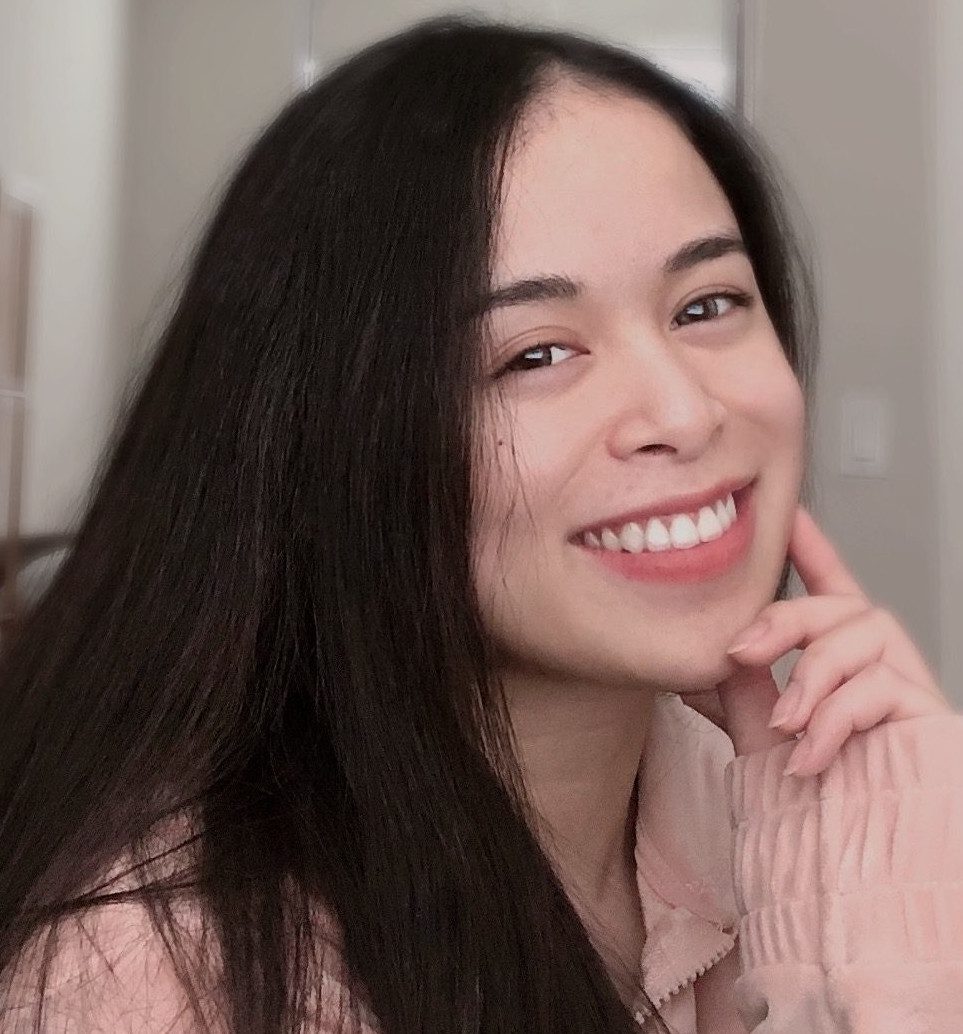
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot