The Python ord() method converts a character into its Unicode code. The ord() method takes one argument: a string containing a single Unicode character. This method returns an integer that represents that character in Unicode.
You may encounter a situation where you need to convert a character into its corresponding Unicode code. For example, you may be creating a profile update form in Python that needs to check each string for emojis and other special characters. These characters are not allowed to be used in the form.
Python has a built-in function that cconvertsa character into an integer that represents the Unicode code of the character: ord().
In this tutorial, we are going to discuss how to use the Python ord() method to convert a character into its Unicode code. We will also explore a few examples of the ord() method being used in a Python program.
Unicode Refresher
Computers, on a fundamental level, deal with numbers. Letters that appear on computers are stored by computers as a list of numbers.
In the past, there were hundreds of different ways that characters could be stored. Often, these methods did not contain enough characters to cover international languages or special characters.
This all changed In 1991. In this year, an organization called the Unicode Consortium published a standardized specification for how characters could be represented with computers.
The Unicode Standard provides a unique number for every character and supports multiple languages, and all special characters. Today, it has been adopted by all modern software providers. In addition, the Python language uses Unicode to represent strings in the programming language.
Unicode assigns each character that can be represented on a computer — including symbols, spaces, and emojis — with a unique code. This code makes it easy for computers to understand the text it is reading.
For example, here are the Unicode values for lowercase characters a through e in the Basic Latin alphabet:
97 98 99 100 101
Python Ord()
The Python ord() method returns the Unicode code of a specific character. This value is represented as a number. You can only use the ord() method on a single character at one time.
For example, say you want to check if each character in a string includes a special character. You could use ord() to do this.
The Python ord() function accepts one argument: the character whose Unicode code value you want to retrieve. The function returns an integer representing the Unicode code value of the character you have passed into the function.
Here’s the syntax for the ord() method:
ord(character)
ord() only accepts one character. If you want to run ord() on multiple characters, you will need to retrieve each individual character from a string and use ord().
ord() is the opposite of the chr() method. Whereas chr() returns the character correlated with a Unicode value, ord() returns the Unicode value of a particular character.
It is worth noting that ord() works with all Unicode characters, not just numbers and letters. You can use symbols with this method, for instance.
ord Python Examples
Let’s use a few examples to show how ord() can be used in Python.
ord() Python Example: One Character
Say that we are creating a reference application that makes it easy for high school computer science students to learn Unicode. Our program takes in one character and returns the Unicode code value of that character. Here’s the code we could use to make such a program:
character = input("The character you want to convert to Unicode") unicode_value = ord(character) print("The Unicode value of the character", character, "is", str(unicode_value))
When we run our code and insert the value K (uppercase) whose Unicode code point we want to retrieve, we receive the following output:
What character do you want to convert to Unicode? K The Unicode value of the character K is 75.
On the first line of code, we use the Python input() function to request a character to convert into its Unicode code value. Then, on the next line, we use ord() to convert the user’s input into its Unicode object value.
We print out a statement that tells the user the Unicode value of the character they have inserted into the program. In this case, the character’s code point is 75, which is returned by our program.
Unicode, as discussed earlier, includes numbers for every character. So, if we were to use ord() on the left square bracket character ([), for example, our code would return:
What character do you want to convert to Unicode? The Unicode value of the character [ is 91.
ord() Python Example: Multiple Characters
The ord() method only takes in one character at a time. Here’s what happens if you pass two characters through the ord() method:
print(ord("AB"))
Our code returns:
TypeError: ord() expected a character, but string of length 2 found
If you want to check the Unicode value of all characters within a longer string, you’ll need to split up your string.
Let’s say that we are creating a program that checks if a user’s name contains a special character. This program first needs the Unicode value of each character in the string. \
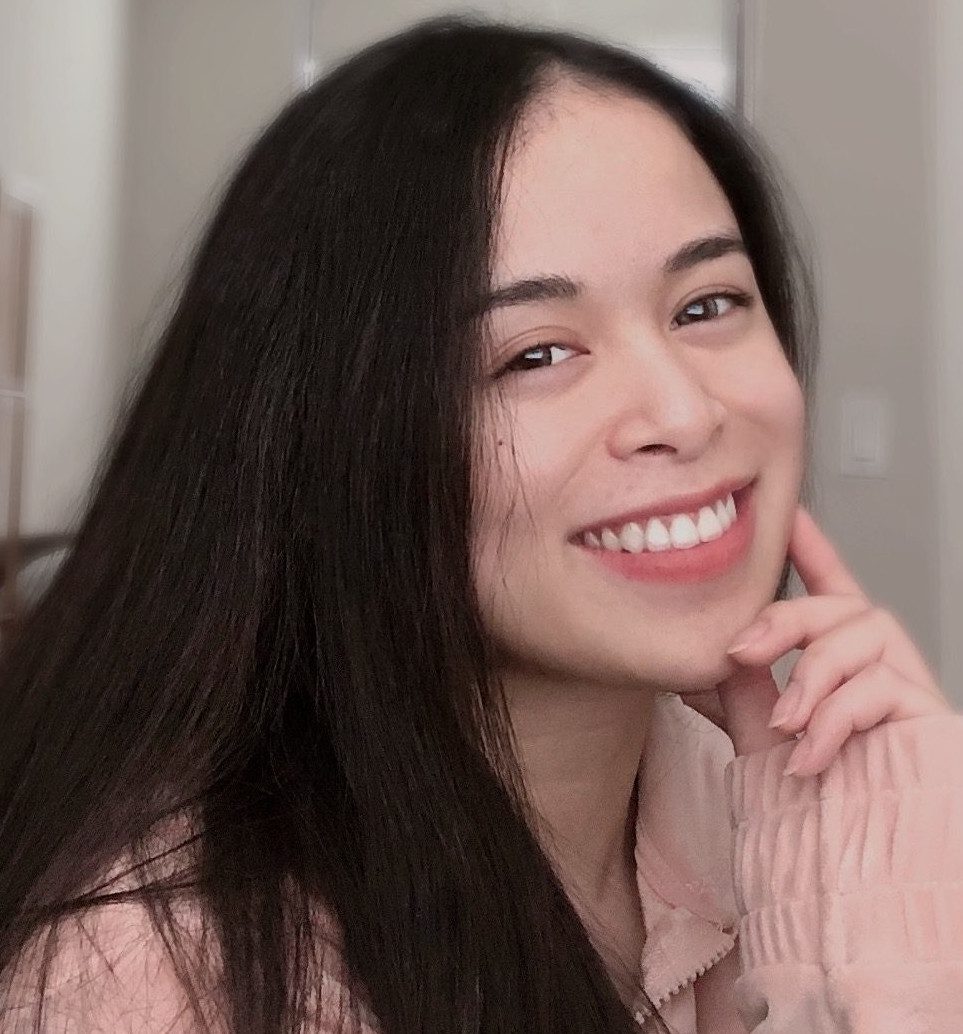
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
We could use a Python for loop to iterate through each character in our string and get the character’s Unicode code. Here’s the code we could use:
user_name = "John" for char in range(0, len(user_name)): print(ord(user_name[char]))
Our code returns:
74 111 104 110
Let’s elaborate. First, we declare a Python variable called user_name which stores the name of our user. Then, we create a for loop that iterates through every letter in the user_name string.
Our program uses ord() to get the Unicode code value of each character in our string, and prints out that value.
As you can see, our program has retrieved the Unicode code values for each character in our string and printed them to the console.
Conclusion
The ord() method in Python converts a character into its Unicode code value. This method accepts a single character. You will receive the numerical Unicode value of the character as a response.
The ord() method is useful if you want to check whether a string contains special characters. This is because each character has a specific Unicode value. You can use these values to compare whether a particular character is alphanumerical or whether it is a special chracter.
Are you interested in learning more about Python? Check out our How to Learn Python guide. You’ll find actionable advice on how to learn Python in this guide so you can chart your path to becoming a Python master.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.