The Python not keyword returns True if a value is equal to False and vice versa. This keyword inverts the value of a Boolean. The not keyword can be used with an if statement to check if a value is not in a list.
How do I check if something is not in a list? How do I check if a condition is not met? The answer to these questions lies in one word: the not keyword.
The not keyword inverts the value of Booleans. Booleans are a data type that can be either one of two values: True or False. They are used to control the logic in a program.
Python if statements depend on Boolean logic. If a statement is True, the if statement will run; otherwise, an elif or else statement will run, or nothing will happen.
In this guide, we’re going to talk about what the not keyword is and how it works. We’ll walk through three examples of where this keyword is used. Let’s get started!
What is the Python not Keyword?
The not keyword inverts the value of a Boolean expression. For instance, the not keyword flips the value of True to False. You’ll see the not keyword used to check if a value is not in a string or another iterable.
We can use the not keyword to check whether a condition is not met.
The not keyword is one of Python’s logical operators, alongside and and or. Read our article on Python logical operators to learn more about these types of operators.
How to Use the Not Keyword
The not keyword has a number of uses. It can be used to:
- Invert the value of a Python Boolean.
- Check if a condition is not met with a Python “if” statement.
- Check if a value is not inside a statement with an “in” statement.
Let’s walk through an example of each of these.
Invert a Boolean
The simplest use of the not keyword inverts a Boolean. This will set the value of a Boolean to be the opposite of the value it has been assigned.
We’re building a program that tracks whether a customer is a loyalty card holder. This program should have a feature that sets the customer’s loyalty card status to inactive if they decide to cancel their membership.
Let’s start by declaring a Python variable to store whether the customer has a loyalty card:
loyalty_card = True
A customer has notified us that they want to cancel their loyalty card. Next, we use the not keyword to do this:
loyalty_card = not loyalty_card print(loyalty_card)
Our code returns: False. This code has successfully flipped the value of “loyalty_card”. If loyalty_card was False, it would turn to True. In this example, loyalty_card was True so it inverted to False.
if not Python
The Python if not keywords check whether an expression returns False. If an expression returns False, the if statement executes. Otherwise, the program does not execute the if statement.
One of the great things about the not keyword is that the keyword makes Python so easy to read:
If not means if an item is not equal to.
We are going to build a tool that checks if a customer is not a loyalty card holder at a clothing store. First, let’s declare whether the customer has a loyalty card:
loyalty_card = True
Our customer is a loyalty card holder. If a customer is not a loyalty card holder, they are not eligible for a discount. Otherwise, they should receive a 5% discount on every purchase. We could use this code to check if a customer is eligible for a discount:
if not loyalty_card: print("This customer is not eligible for a discount.") else: print("This customer is eligible for a 5% discount.")
The “if not loyalty_card” checks if the value of “loyalty_card” is False. In this example, loyalty_card evaluates to True. This means that our expression evaluates to False and the contents of our else statement are executed. This prints the following message to the console:
This customer is eligible for a 5% discount.
Our customer is a loyalty card holder, so they are eligible for a discount.
Not In Python
The not in Python keywords check if an item is not inside a list. An in statement checks if a value is in a list. Then, the not statement inverts the value returned by the in statement.
You can use the not in operators in Python to check if an item is not inside a list. Let’s take the following list as an example:
presidents = ["George Washington", "John Adams", "Thomas Jefferson"]
This is a list of the first three Presidents of the United States. We can use the not in keyword to check if a particular person was in this select group.
A friend has just asked whether Alexander Hamilton was one of the first three presidents. We can check this using the following code:
presidents = ["George Washington", "John Adams", "Thomas Jefferson"] if "Alexander Hamilton" not in presidents: print("Alexander Hamilton was not one of the first three presidents.") else: print("Alexander Hamilton was one of the first three presidents.")
This expression checks whether “Alexander Hamiltion” is not in our list of presidents. If he is not in the list, the contents of our if conditional statement are run; otherwise, the contents of our else clause are run.
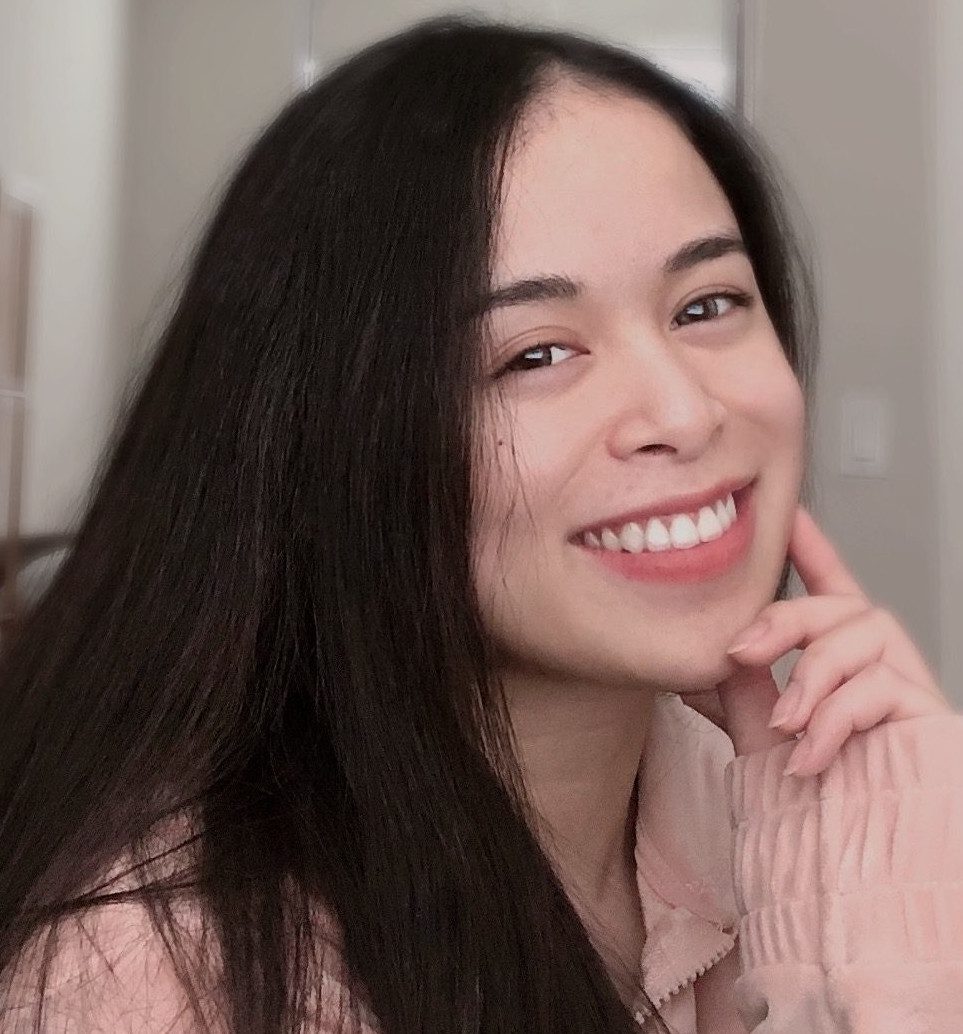
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Alexander Hamilton is not in our list so our statement else prints the following to the console:
Alexander Hamilton was not one of the first three presidents.
Conclusion
The not keyword is used in Boolean logic to control the flow of a program. This keyword inverts the value of a Boolean. You can use the not keyword to check if a value is not inside another value.
Are you up for a challenge? Write a program that checks whether your favorite food is in a dictionary of foods. You can make up this dictionary of foods for yourself. To learn more about dictionaries, read our tutorial on Python dictionary values.
Do you want to learn more about Python programming? Check out our complete How to Learn Python guide. In this guide you’ll find expert advice on learning Python and a list of top online courses and learning resources.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.