The Python shutil.move() method moves a file to another location on your computer. This method is part of the shutil model, which you must import before using this method.
Moving files is a common operation in Python programs. For instance, say you are creating a program that generates files. You may want to move all existing files from a folder somewhere else, to make room for the new files you want to create.
That’s where the shutil.move() function comes in. The shutil.move() function allows you to move a file from one folder to another on your system. This tutorial will discuss, with reference to examples, how you can use the shutil.move() function to move your code.
Python shutil
The shutil module provides a wide range of high-level operations that you can execute on files. Unlike the os library, shutil comes with functions that can be executed on collections of files.
For this tutorial, we’re going to focus on the shutil.move() function, which allows us to move a file using Python.
Before we explore the move() function, we must first import the shutil library into our code. We can do so using this Python import statement:
import shutil
Python Move File
The shutil.move() function moves a file on your computer. This method accepts the file path of the file you want to move and the new file path as arguments.
The syntax for this function is as follows:
shutil.move(source, destination)
shutil.move() accepts two parameters, which are:
- source path: The file path of the file you want to move.
- destination path: The file path where you want to move your file.
The move() function returns the path of the file you have moved.
If your destination matches another file, the existing file will be overwritten.
The file paths you specify can be either absolute or relative.
Absolute file paths are complete paths that lead directly to a file (i.e. /home/career_karma/file.txt). Relative file path refers to a location that is relative to the directory in which your Python program is being run (i.e. file.txt).
If you specify a destination directory that does not exist, a new directory will be created.
The os library contains the os.rename() method which is often used to rename files. This method can also move files. But, the shutil method was designed specifically for moving files. The syntax shutil.move() is easier to understand than os.rename() if you are moving a file.
Python Move File Examples
Let’s explore a few examples of how to use the shutil.move() function.
Move a Single File
Suppose we have a file called raw_data.csv which we want to move into a directory called data in our current working directory. We could do so using this code:
import shutil source = "raw_data.csv" destination = "data" new_path = shutil.move(source, destination) print(new_path)
Our code returns:
data/raw_data.csv
First, we have imported the shutil library. Next, we have declared two Python variables. The source variable stores the name of the file we want to move. Our destination variable stores the name of the directory in which we want to move our file.
In this example, we have specified relative file paths for our source and destination. This means that the file raw_data.csv and the directory data refer to those in the same directory as our Python file. If our Python file is stored in /home/career_karma/program, the file and directory we reference will be the ones stored in that directory.
Next, we use shutil.move() to move our file. We assign the result of the operation — the path of the moved file — to the variable new_path. Next, we print out the value of new_path, which returns the path of our new file.
We’ve successfully moved a file in Python.
Note: The same syntax we used to move a file can also be used to move a folder.
Move a File and Change Its Name
The shutil.move() function allows you to change the name of a file once it has been moved.
Suppose we want to move raw_data.csv into a folder called data, and change the name of our file to raw_data_2019.csv. We could do so using this code:
import shutil source = "raw_data.csv" destination = "data/raw_data_2019.csv" new_path = shutil.move(source, destination) print(new_path)
Our code returns:
data/raw_data_2019.csv
When we specify the destination for our new file, we also specify a new name for our file. We specify the destination data/raw_data_2019.csv. This means that when our file is moved, it will be moved into the data directory. The new file will be given the name raw_datra_2019.csv.
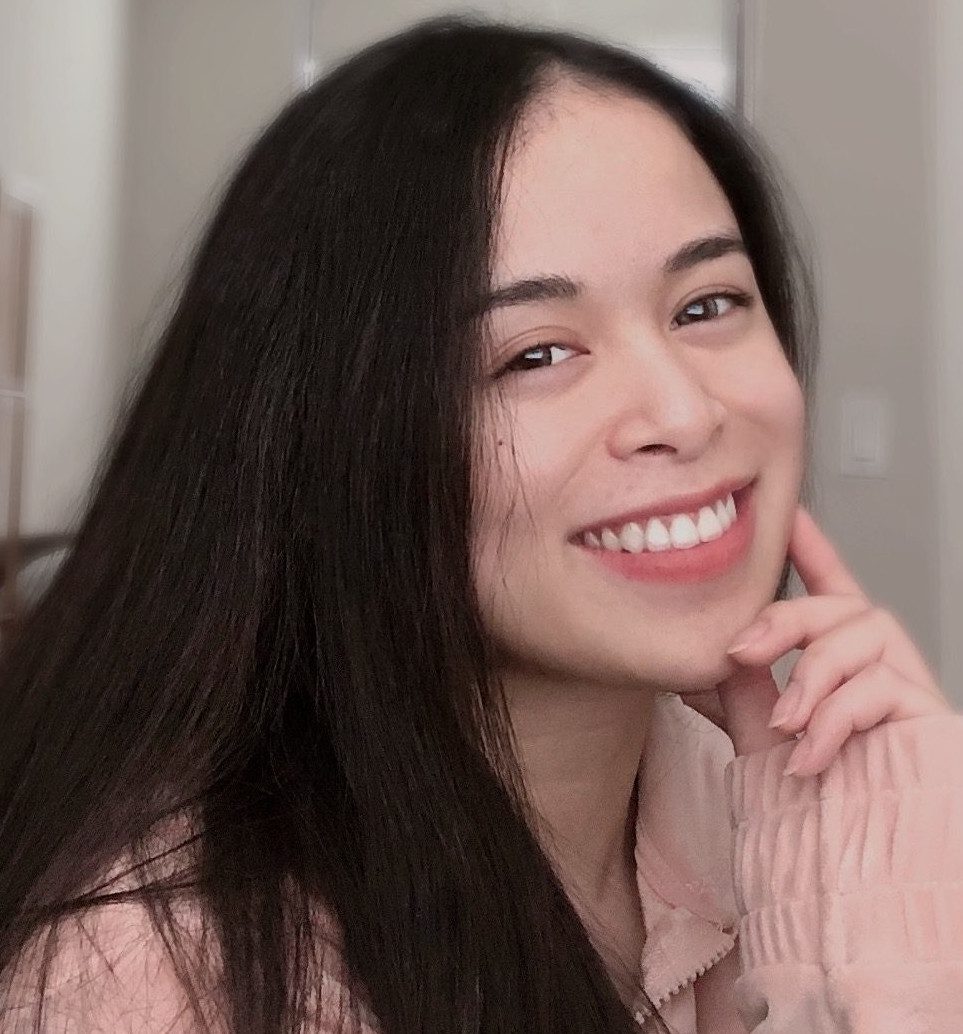
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Move Multiple Files
We can also use the shutil.move() function to move multiple files. To do so, we are also going to reference the os library. We can use the os.listdir() method to get a list of files in a directory.
Suppose we want to move all the files in the /home/career_karma/data directory to a new directory called /home/career_karma/old_data. The data directory contains the following files:
- /home/career_karma/data/data.csv
- /home/career_karma/data/old_data.csv
We could do so using this code:
import shutil import os source = "/home/career_karma/data" destination = "/home/career_karma/old_data" files = os.listdir(source) for file in files: new_path = shutil.move(f"{source}/{file}", destination) print(new_path)
Our code returns:
/home/career_karma/old_data/data.csv /home/career_karma/old_data/old_data.csv
Let’s break down our code. First, we import the shutil and os libraries into our program. Then, we specify the absolute paths for the folder whose contents we want to move. We also specify the path for the destination in which the contents of our folder should be moved.
Next, we use os.listdir() to retrieve a list of all the files in the folder whose contents we want to move. We use a Python for loop to iterate through each of these files. Then, we use shutil.move() to move each individual file to our destination folder.
In our move() function, we use an f string to specify the full file path for the file we want to move. Our code displays the file path of our newly-moved files.
Conclusion
The shutil.move() function moves a file from one location to another on your computer. You must specify the path of the file you want to move as well as the new path for the file.
Do you want to learn more about coding in Python? Check out our How to Learn Python guide. You’ll find expert advice on how to learn Python in this guide. Our guide also contains a list of top learning resources to help you expand your knowledge.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.