Modules are a feature of Python that allow you to divide your code into multiple files. Python modules are files that end in the “.py” extension.
In this guide, we’ll provide examples and you’ll learn how to create a Python module.
Python Modules: A Primer
A module is another word for a Python program file. This means that any file in a Python project that ends in the .py
extension can be treated as a module. Modules can include functions, variables and classes, and can also import their own libraries.
There are three main types of modules in Python:
- Built-in Modules: These are modules that are part of the Python Standard Library. They are packaged with your Python installation. Some examples include logging and time.
- External Modules: These are modules you have installed using pip, Python’s package management tool.
- User-Defined Modules: These are functions defined by you in your Python program.
Writing modules can help you preserve the readability of your code. While it’s technically possible to write all of the code for a program in one file, it’s not the best idea. Knowing where and how to make changes to a file with potentially thousands of lines of code is difficult.
We recommend reading our tutorial on the Python import statement if you’re not familiar with how it works already.
How to Write a Python Module
In this guide, we’re going to write a program for a bank that stores the balances of their customers. Let’s begin.
To start, we are going to create a function that welcomes the user to the bank’s balance tracking system. We are going to write this function in a file called bank.py, which will store the code for our bank tracking system.
def welcome_message(): print("Welcome to Python Bank!")
When we run this program, nothing will happen. This is because we haven’t called our function. To use our code, we are going to create a new file called main.py. This will store the main code for our program:
import bank bank.welcome_message()
In our code, we have imported the bank
module from our bank.py
file. We have then called the welcome_message()
function in our bank
module. Our code returns:
Welcome to Python Bank!
While our print()
statement is in the bank.py
file, using the import
keyword we can call that code in our main program. This is a basic example of how you can run code from a different file in Python, but there’s a lot more you can do with a module.
Using Classes with Python Modules
Suppose we want to create a class which stores the data of a customer in our bank. We’re going to define this class in our bank.py
file, so it is away from our main program:
def welcome_message(): print("Welcome to Python Bank!") class Customer: def __init__(self, name, balance): self.name = name self.balance = balance def show_customer(self): print("Name: " + self.name) print("Balance: $" + int(self.balance))
Let’s go back to our main.py file so we can use this code. We’re going to add in the following code which creates an instance of the Customer class. This instance will store the name and balance of Lucy, a new customer to the bank.
Our main.py file now looks like this:
import bank bank.welcome_message() lucy = bank.Customer("Lucy", 75) lucy.show_customer()
Our code returns:
Welcome to Python Bank!
Name: Lucy
Balance: $75
In our code, we have declared an instance of the Customer class called “lucy”. Lucy’s account has the name “Lucy” and a balance of $75.
As you can see, the code for our Customer class is not stored in our main program.
Instead, this code is in our bank.py
file. The “import” statement allows us to retrieve all the code in the bank.py
file so we can use it in our code.
How to Import Modules from Another Folder
In Python, you can use modules that are stored in different directories.
In our last example, our “bank.py” module was in the same folder as our “main.py” folder.
This is because our program is short and so we don’t have a need for a separate module folder. But, if you are writing a larger program, you may want to store modules in separate folders.
To do so, you can use the “from” keyword. This will allow you to specify the folder from which you want to import a module.
Let’s say we are moving our bank.py
module into a folder called bank_details
. We could then import specific modules in our code by changing our import statement from “import bank” to:
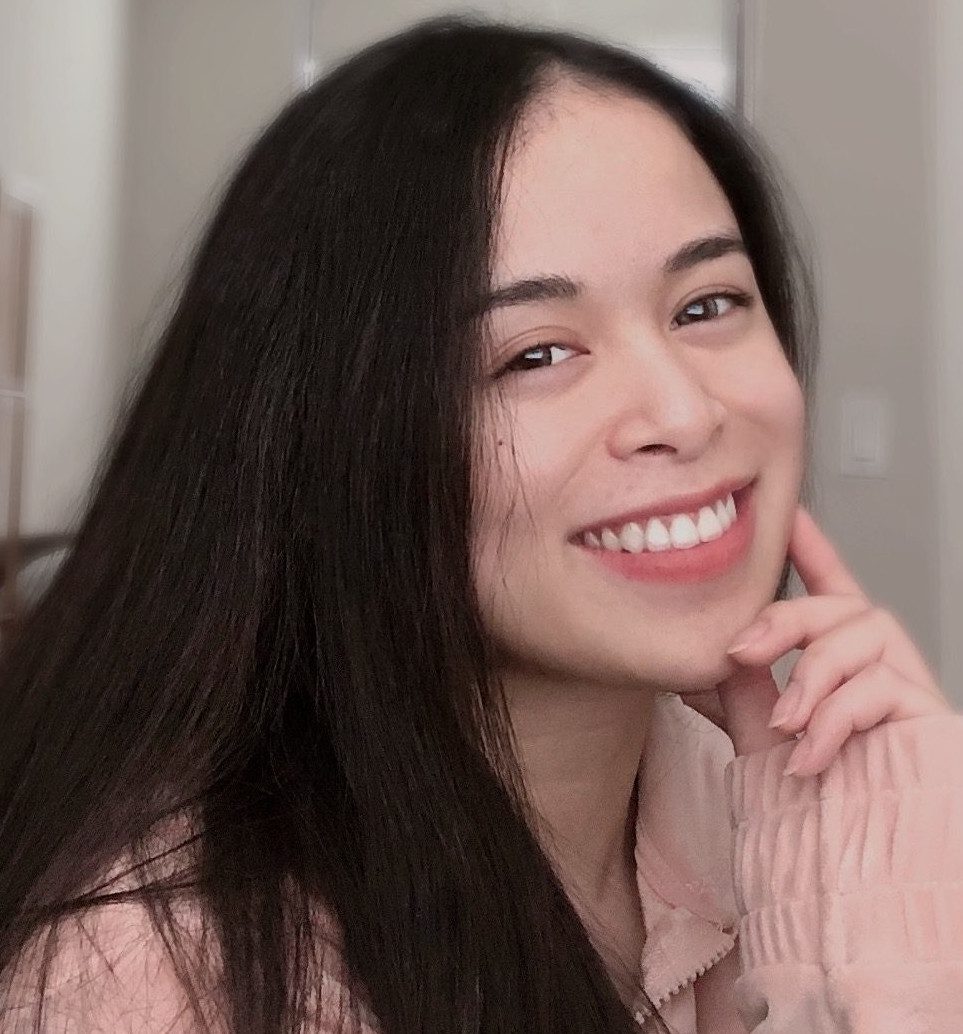
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
from bank_details import bank
This will allow us to import the contents of our bank.py
file into our program. from bank_details
tells our program where to find the module, which is often called the module namespace. In this case, bank_details
is the module namespace and is the file containing the Python code that we use in our program.
Conclusion
There’s no difference between writing a module and any other Python file. A module is simply a file in Python. When used with an “import” statement, you can use modules to break up your code. This is useful because it means you don’t need to store all the code for your program in one file; you can split it up into multiple files and categorize your code accordingly.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.