For numerous reasons, Python has become one of the most powerful programming languages in existence. The fact that it’s easy to learn has made it an enormously popular language for beginners, but it isn’t a toy. Python has found application just about everywhere that computers are used, from embedded systems programming to high-performance computing.
One of the things that makes any programming language more useful is the kinds of modules that are available for it. In this piece we’re going to discuss how modules are used and how to install one.
What Are Python Modules Used For?
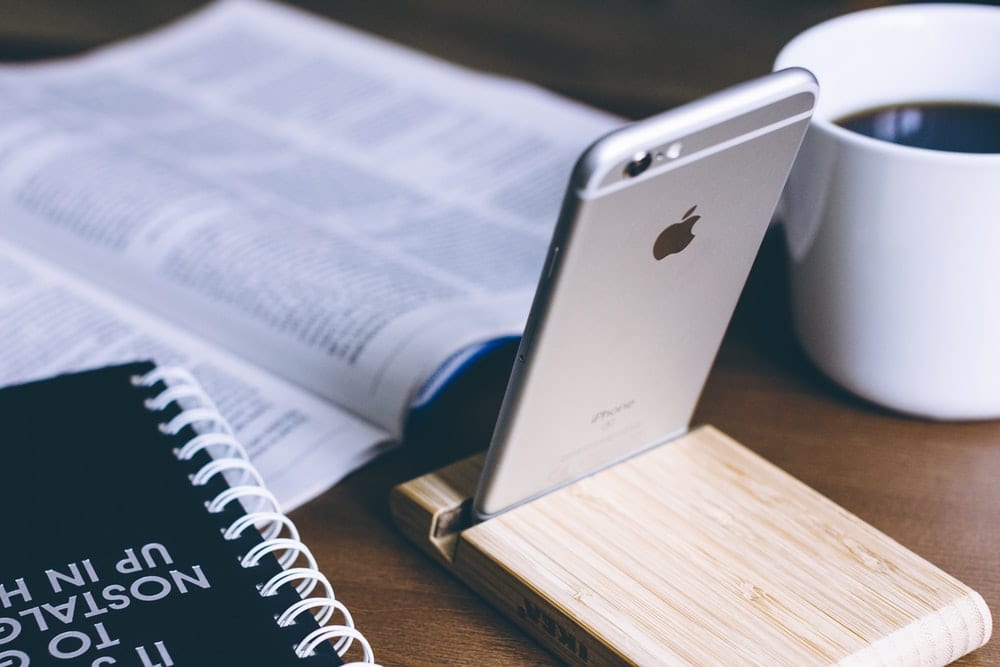
In the simplest possible terms, a module is just a file containing code, and a Python module contains Python code. Every major language has additional modules which can be deployed in a coding project, and languages with thriving communities have a wide array of 3rd-party modules you can tap into as well.
Modules are usually thematic; they focus on solving some particular problem. Python’s urllib module, for example, is for working with URLs. If you’ve never tried to do this the long way, trust me when I tell you it’s a real pain. Luckily for us, some kind soul wrote urllib to make the whole process quicker.
Difference Between a Module and a Library
From everything I’ve seen, these two terms are used interchangeably. You might see a module strictly defined as offering one function while a library offers lots of functions. But in practice, nobody is going to insist on this distinction.
Technically urllib is a library, for example — ‘library’ is right there in the name. But if you Google ‘urllib module’ you’ll find lots of results discussing urllib as a module.
Working with Modules
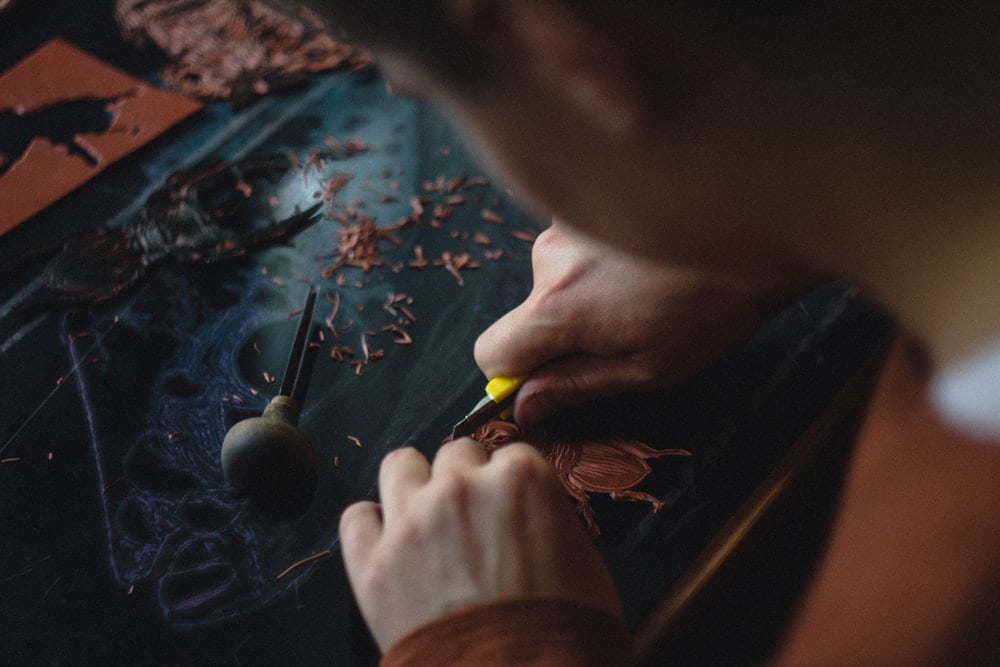
In Python, there are two basic ways to interact with a module. You can import the whole file:
import some_module
Or you can import a specific piece of the module:
from some_module import a_specific_function
These import statements almost always go at the top of your program. To the best of my knowledge, there isn’t a single compelling reason for using one approach over the other. If you need lots of methods from a module you should probably import the whole thing, if you only need one piece of it you can get away with just grabbing that.
Importing individual functions does have the advantage of reducing the amount of typing you have to do. If you import a whole module, anytime you want to access a function you’ll need to type the entire module name first, like this:
some_module.a_specific_function()
This isn’t necessary if you import a function by name.
I’ve sometimes seen people split the difference by importing the entire module and also, simultaneously, importing specific functions from it:
import some_module
from some_module import this_function, that_function
This way, they have access to everything the module has to offer while avoiding the need to do so much typing when using a function that appears frequently in their program.
But in order to even make it this far, we first have to know whether or not the module is available as a core part of the language or if we’ll have to install it. Python, the language I’m most familiar with, comes pre-loaded with hundreds of modules for accomplishing all sorts of everyday tasks. These are available as soon as I import them into a program. But others must be downloaded from somewhere, and there are two major ways to do this: pip and conda.
Pip is the most popular package management system for python. It is used is for installing non-standard modules. By and large, using it is as simple as typing pip install your_module_name.
One of the major competitors to pip is Conda, which works across many languages and performs a similar package-management function.
I’m more inclined to use pip, but either should work fine for getting you the modules you need to complete your project.
With this, you should be well on your way to using python modules like a pro!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.