The Python max() function is used to find the largest value in a list of values. The Python min() function is used to find the lowest value in a list. The list of values can contain either strings or numbers.
You may encounter a situation where you want to find the minimum or maximum value in a list or a string. For instance, you may be writing a program that finds the most expensive car sold at your dealership. That’s where Python’s built-in functions min() and max() come in.
In Python, you can use min() and max() to find the smallest and largest value, respectively, in a list or a string. This guide will explore how to use the min() and max() methods in Python and will walk you through a few examples of each.
Python min() Function
The Python min() function returns the lowest value in a list of items. min() can be used to find the smallest number in a list or first string that would appear in the list if the list were ordered alphabetically.
Here’s the syntax for the Python min() method:
min(object)
In this syntax, the min() method only takes in one parameter: the object whose minimum value you want to find.
We can also specify individual values as arguments with the min() function:
min(value1, value2...)
The largest value out of all the values we specify as arguments are returned if you choose to specify individual values.
You can pass an iterable like a list or a list tuple as an argument to the min() method. If an iterable is empty, a ValueError is raised like this:
ValueError: max() arg is an empty sequence
Read our article on the “ValueError: max() arg is an empty sequence” (which also applies to the min() method) for more information.
min Python Example
Here’s a simple example of using the min() method to find the lowest value in a list:
example_list = [21, 22, 19, 14] print(min(example_list))
Our code returns: 14.
On the first line of our code, we define a list called example_list that stores four values. On the next line, we use min() to find the smallest value in that list and print that value to the console.
Let’s use a more detailed example to show this method in action. Say that we are a coffee shop owner, and we made a list of potential milk vendors. We found six suppliers and created an array that stores the price per gallon that each vendor quoted to us.
We want to find the cheapest supplier in our list, which we can do using the following program:
supplier_quotes = [3.28, 3.27, 3.29, 3.30, 3.32, 3.26] print("The cheapest price is $", min(supplier_quotes), " per gallon of milk.")
Our code returns the smallest item in our iterable:
The cheapest price is $3.26 per gallon of milk.
On the first line of our code, we define a list of vendor quotes. Then, we use the min() method to find the smallest value, and we print out a message with the result of that method. In this case, the cheapest price available was $3.26 per gallon of milk.
Python Max Function
The Python max() function returns the largest value in an iterable, such as a list. If a list contains strings, the last item alphabetically is returned by max().
Let’s look at the max() function Python syntax:
max(value1, value2...)
You can see that you can specify individual values from which the highest value will be selected.
We can also use this syntax where we pass an iterable as an argument:
max(list)
The second example is the most common use of max(). This code reads through the contents of the specified iterable, such as a list, and returns the largest value in that iterable.
Passing an empty value as an argument to the max() function will result in the same ValueError we discussed earlier.
max() Python Example
Say that we are a premium coffee house and we are looking to pay a premium price for quality milk. We could find the largest quote that we received by using the following code:
supplier_quotes = [3.28, 3.27, 3.29, 3.30, 3.32, 3.26] print("The most expensive price is $", max(supplier_quotes))
Our code returns the largest item in our list:
The most expensive price is $3.32
As you can see, our code is almost identical to the code in the min() example above. Instead of using min(), we used max().
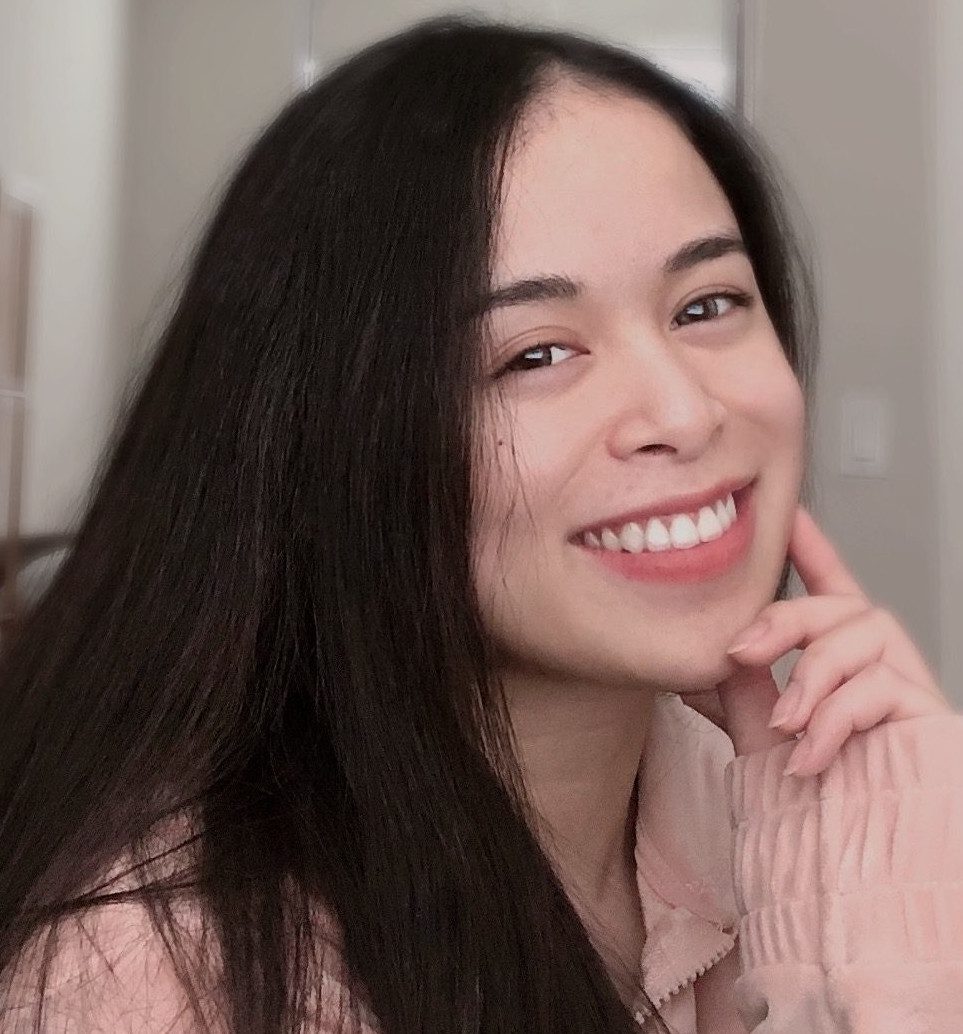
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
The max() method searched through our Python array and computed the maximum value in our list. Then, our program printed out a message to the console with that value.
Python Min and Max with Strings
In the examples above, we used the min() and max() methods to find the smallest and largest values in a list.
The min() and max() methods can also be used to find the smallest and largest characters in a string. In this case, smallest and largest refer to the position of the character in the alphabet.
The smallest possible character is the capital letter A, since all capital letters come first in Python. Our largest character is the lowercase letter z. (To learn more about Python’s ordering system, check out our tutorial on the Python ord() method.)
Let’s say that we have a string that contains the grades for every student in a fifth-grade math class. We want to know what the lowest grade was. To calculate the lowest grade, we could use max() function. Here’s an example program that accomplishes this task:
grades = "AABABBACBAABCD" print("The lowest grade in the class was", max(grades))
Our code returns:
The lowest grade in the class was D
Our code searches through a Python string to find the smallest value. In this case, the lowest grade was D, which our max() function identified and returned to our code.
When you’re working strings and the min() and max() methods, you can specify multiple parameters. This allows you to find the smallest and largest string out of multiple values.
Say that you have three student names and you want to find out which one comes last alphabetically. You could do so using the following code:
name_one = "Garry" name_two = "Lenny" name_three = "Jerry" print("The name that appears last in the alphabet is", max(name_one, name_two, name_three))
Our code returns:
The name that appears last in the alphabet is Lenny
The max() method took in three parameters: name_one, name_two, and name_three. The max() method then calculated which of these names comes last alphabetically and returned that name to the program.
Finally, our code printed out a message that stated the name that appears last in the alphabet.
Conclusion
Often, when you’re working with lists or strings, you’ll want to find the lowest or highest value that appears in that list or string. The Python min() and max() methods can be used to do so.
This tutorial discussed how to use the min() and max() methods with Python lists and strings. You’re now ready to start working with min() and max() on lists and strings like a pro!
To learn more about Python programming, read our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.