The Python map() function executes a function on each item in a collection, such as a list or a set. The map() function accepts a function and an object to apply that the function will operate on as arguments.
When you’re working with a list of items in Python, you may want to perform a specific function on each of those items.
That’s where the Python built-in function map() comes in. The Python map function executes a function on all elements within an iterable object, such as a list, and returns map objects.
In this tutorial, using a series of examples, we discuss how to use the map() function in Python.
Python Iterable Objects
Iterable objects are items that contain a countable number of values and that can be traversed. Lists, dictionaries, tuples, and sets are all iterable objects in Python as they can contain multiple values and can be traversed.
Let’s say that you have a list of student names that you want to store. Instead of storing these names in multiple Python variables, you can declare an array to store the values:
students = ["Lucy", "Bill", "Graham", "Tommy", "Leslie"]
Our students
variable contains a list, which is an iterable object. This means that we can traverse through the items in the list.
Python Map Function Syntax
The map() function passes each element in a list and executes a function on each element. map() is built-in to Python. This means that you do not need to import any libraries to use the map() method.
Python map() is a higher-order function that can be used to apply a specific function to multiple elements in an iterable object. The syntax for the Python map() function is as follows:
map(function, iterable)
The first argument the map() function takes is the function. This is the function that will be executed on every item in the iterable. The iterable is the object that will be mapped, such as a list, tuple, dictionary, or set.
How to Use the Map Function in Python
Say you are an administrator at a school that has been tasked with creating a full student roster.
We decide to print out a list of student names with each student’s class grade listed next to their name. This will prevent confusion if two students in two different grades have the same name.
This is a perfect application of the Python map() function. We have an iterable upon which we want to execute a function. The function will merge a student’s name with the grade they are in. Here’s a program that could be used to merge the names of students with their grade level:
def mergeNamesAndGrades(name): return name + " First Grade" students = ["Lucy", "Bill", "Graham", "Tommy", "Leslie"] student_roster = map(mergeNamesAndGrades, students)
On the first two lines, we declare a Python function called mergeNamesAndGrades. This function combines each student’s name with First Grade.
Then, on the next line, we define the list of students in our school. THere are five students in our list.
The map() function is applied to the contents of the student_roster Python variable. The map() function takes in two arguments: the function (in this case, mergeNamesAndGrades) and the iterable object (students).
The map() method applies the mergeNamesAndGrades() function to each student in our list.
Converting a Python Map to a List
However, our program is not yet complete. The map() function returns a mapped object, not our complete list. If we print out our student_roster variable right now, our code will return a mapped object like this:
<map object at 0x7f2c74103890>
This output was generated because the map() function returns its own custom object, rather than a list. So, if we want to print out the list of student names, we need to convert our student_roster variable to a list. Here’s the code we can use to perform this action:
print(list(student_roster))
Our code returns the following Python list:
['Lucy First Grade', 'Bill First Grade', 'Graham First Grade', 'Tommy First Grade', 'Leslie First Grade']
Let’s look at another example. Say that we want to convert every student’s name and grade into upper case for our student roster. We could use the following code to change the cases of the student names and grades:
def changeCase(name): return name.upper() student_roster = ['Lucy First Grade', 'Bill First Grade', 'Graham First Grade', 'Tommy First Grade', 'Leslie First Grade'] final_student_roster = map(changeCase, student_roster) print(list(final_student_roster))
Our code returns a list over which we can iterate:
['LUCY FIRST GRADE', 'BILL FIRST GRADE', 'GRAHAM FIRST GRADE', 'TOMMY FIRST GRADE', 'LESLIE FIRST GRADE']
In this example, we defined a function called changeCase. This function changed each student name to uppercase. We used the Python upper() function to convert the case of each name.
Our program used the map() function to call changeCase() on each object in our iterable student_roster. Finally, our program returned the revised list of student names in capital letters.
Python Map Function with Lambda
The Python map() function can be used with the lambda function to make our code more efficient. In the above examples, we declared a new function to change our iterable in a certain way.
But if we only want to perform an action on an iterable object once, it’s not necessary to declare a new function. Instead, we can use the Python lambda function, which is a small, anonymous function.
Lambda functions can be used with map() for small functions where we don’t want to define a new function.
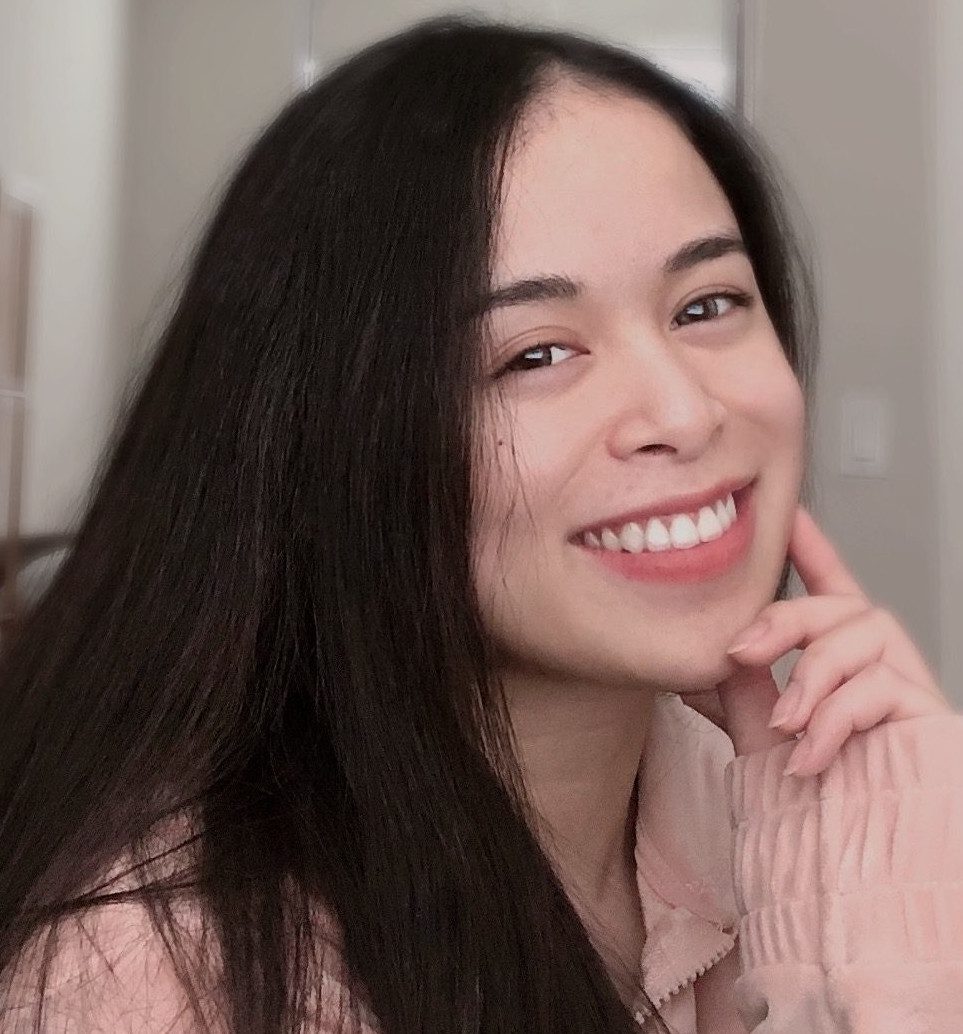
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Because lambda functions are more concise than regular functions, you should try to use them when you can. Using lambda functions for simple functions will help improve the readability of your code.
Here’s an example of lambda being used to convert the case of a list of student names to uppercase, like we did above:
student_roster = ['Lucy First Grade', 'Bill First Grade', 'Graham First Grade', 'Tommy First Grade', 'Leslie First Grade'] final_student_roster = map(lambda s: s.upper(), student_roster) print(list(final_student_roster))
We did not declare a new function to convert the cases of our student names to uppercase. Instead, we used lambda s: s.upper(), which is a small, anonymous function that will convert the cases of our student names.
Our code returns the following:
['LUCY FIRST GRADE', 'BILL FIRST GRADE', 'GRAHAM FIRST GRADE', 'TOMMY FIRST GRADE', 'LESLIE FIRST GRADE']
Conclusion
The Python map() function can be used to apply a specific function to all elements within an iterable object. For example, you can use map() to convert the cases of a list of strings to uppercase. Or, you could use the map() function multiply a list of numbers by a certain amount.
In this tutorial, we discussed how you can use map() in Python to apply a function to all elements within an iterable object. We also discussed how you can use Python’s lambda function with map() to create more efficient code.
You’re now equipped with the Python knowledge you need to start using map() like a pro.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.