The Python main() method contains the methods and code you want to execute in a main program. It is not necessary because Python runs code line-by-line but the main() method helps make code more readable.
How to Use Python Main Functions
“I’ve seen a few tutorials that use main() in Python. What does it mean?” That’s a great question. The main() function may seem trivial when it’s not actually necessary.
Coding is all about being concise; you’re probably curious why developers want to add in more lines of code into a program. The main() function may add an extra few lines of code into your Python program, but it does serve an important purpose.
In this guide, we’re going to talk about what the main() function is and why it is useful.
What is the Python main() Function?
The Python main() function lets you introduce logic into your Python programs. A main() function is executed as soon as a program is run. It defines the order in which particular functions should be executed.
The syntax for the main() function is:
def main(): yourmaincode...
The main() function appears at the bottom of a program, after all modules have been imported and functions have been declared.You can call a Python function at the bottom of your program and it will run. But, it can be quite confusing that the code at the bottom of a program is the main program. In other programming languages like C++ and Java, all the main code for a program is contained within a main function.
A main() function a good way of breaking down all the code in your programs. People who are new to Python but who know other programming languages like C++ will thank you for adding in a main() function.
Main Function Python: Walkthrough
There are two parts of a Python main function. The first part is the actual main() function which stores the code for our main program. Let’s call our cookies() function and print out the statement: “I like cookies.” from within a main() function:
def cookies(): print("Cookies are delicious!") def main(): print("I like cookies.") cookies()
Finally, let’s call our function at the bottom of our program:
main()
We can now run our code:
Cookies are delicious! I like cookies!
It is clear exactly what is happening in our program:
- Cookies() is a function that prints “Cookies are delicious.”
- When our program is run, our two functions, cookies() and main(), are defined.
- Then we call the main() function.
- “I like cookies.” is printed to the console.
- The cookies() function is then called, printing “Cookies are delicious!” to the console.
Our code is not only cleaner, but it’s also more logical. If you come from another language like Java, you’ll know how useful this is.
What is Python __name__?
The Python __name__ statement refers to the name of a script. If you run a program as a module, it will take on the name of the module. Otherwise, __name__ will have the value __main__, which refers to the program you are running.
Before we talk about the __name__ and __main__ statements that are often used together with a main function, we’ve got to talk about __name__. __name__ stores the name of a program.
When you run a file directly, __name__ is equal to __main__. Consider this file called print_name.py:
print(__name__)
We can run our code like this:
python print_name.py
Our code returns: __main__.
Suppose we were to import this as a module into a file called main.py. Let’s create a file called main.py and import the print_name module:
import print_name
Let’s run name.py:
python name.py
Our code returns:
print_name
The code within our print_name.py file is executed because we imported it as a module in our main program. The print_name.py file prints __name__ to the console. Because we imported print_name as a module, the value of __name__ is print_name.
What is if __name__ == __main__?
The Python if __name__ == __main__ statements execute the code they contain if you run a file directly. This statement prevents some code from executing if a program is called as a module.
You’ve probably seen something like this in a Python program with a main function:
if __name__ == "__main__":
What does this mean? That’s another good question. In Python, any variable that starts and ends with __ is a special variable. These are reserved values that have specific purposes in a Python program.
__main__ refers to the scope where your code will execute. When you run a Python file directly, the value of “__name__” is set to “__main__”. However, when you run a file as a module, the value of “__name__” is not “__main__”; it is set to the name of the module.
This means that the line of code above will evaluate to True when our program is run. But, this line will only evaluate to True if we run our program directly from the command line instead of from a Python module.
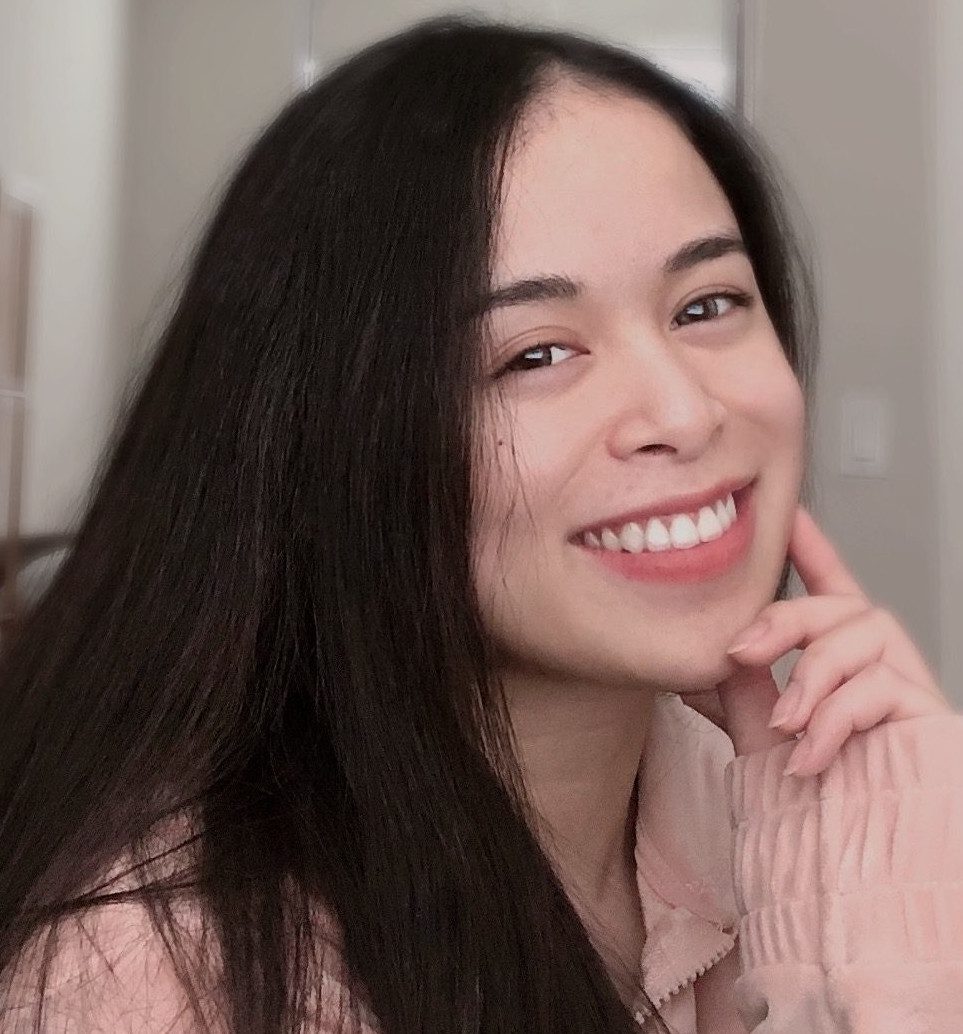
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
If we reference our file as a module, the contents of the Python “if” conditional statement will not be executed. Let’s demonstrate how this works with an example.
Python if __name__ == __main__ Example
Let’s create a new Python script called username.py. In this file, we’re going to ask a user to select a username and check if it is more than five characters long. If the username is not longer than five characters, the user will be asked to choose a new username.
We’ll start by defining a global variable to store our user’s username:
username = ""
We’re going to define two functions. The first function will ask a user to select a username and check if it is more than five characters long. The second function will print out that username to the Python shell:
def choose_username(): global username username = input("Choose a username: ") if len(username) > 5: print("Your username has been selected.") else: print("Please choose a username greater than five characters long.") choose_username() def print_username(): print(username)
We’ve used the Python “global” keyword so that the contents assigned to the “username” variable inside the choose_username() method will be accessible globally. Now that we’ve defined our functions, we’re going to create a main function which calls these functions:
def main(): choose_username() print_username()
We’re then going to add in the if __name__ = ‘__main__’ statement. This means that if we run our source file directly, the Python interpreter executes our two functions. If we run it as a module, the contents of our main() method will not execute.
if __name__ == "__main__": main()
Let’s run our code:
python username.py
Our code returns:
Choose a username: CareerKarma Your username has been selected. Career Karma
Our code runs the choose_username() function, and then executes the print_username() function. If we select a username that is fewer than four characters, we’ll see a response like this:
Choose a username: CK Please choose a username greater than five characters long. Choose a username:
We’re prompted to choose another username.
If we were to import this code as a module, our main() function would not run.
Conclusion
The main() function is used to separate blocks of code within a Python program. While it’s not necessary to write a main() function in Python, writing one is a good way to improve the readability of your code.
Writing a main() function is mandatory in languages like Java, on the other hand. A main() function it makes it clear in what order lines of code run within a program. Including a main() function in your program is a good practice for all programmers for this reason.
Now you’re ready to start writing and working with main methods in Python like a professional! For more Python learning resources, check out our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.