When you’re working with conditional statements, you may want to make more than one comparison in the statement. For instance, you may want to check if two statements evaluate to True, or if one of two statements evaluate to False.
That’s where the Python logical operators come in. Logical operators are a special type of operator that allows you to make more than one logical comparison in a conditional statement.
This tutorial will discuss, with examples, the basics of operators, and how to use the three logical operators offered in Python.
Python Operators
An operator is a symbol that indicates a special operation in Python. For instance, the minus sign (-) operator indicates a subtraction operation.
In Python, there are three different types of operators. These are:
- Arithmetic operators: These allow you to perform mathematical operations in a program.
- Comparison operators: These allow you to compare values, and returns True or returns False.
- Logical operators: These allow you to combine conditional statements.
The second two operators—comparison and logical—allow programmers to better control the flow of a program. For instance, you can use a comparison operator to check if a condition is True, and if it is, run a certain block of code in your program.
Comparison and logical operators are often used with an if
statement. For instance, suppose we wanted to check whether a user of an online shopping site was aged 16 or over. We could do so using this code:
age = 17 if age >= 16: print("User is 16 or over!") else: print("User is under 16!")
Our code returns: User is 16 or over!
In this program, we used a comparison operator to compare whether the user’s age, which is equal to 17 in this case, was greater than or equal to 16. Because 17 is greater than 16, this statement evaluated to True, and the message User is 16 or over!
was printed to the console.
But what if we want to run multiple comparisons in an if
statement? That’s where logical operators come in.
Python Logical Operators
Python offers three logical operators that allow you to compare values.
These logical operators evaluate expressions to Boolean values, and return either True or False depending on the outcome of the operator. The three logical operators offered by Python are as follows:
Name | Description | Example |
and | True if both expressions are true | a and b |
or | True if at least one expression is true | a or b |
not | True only if an expression is false | not a |
Logical operators are usually used to evaluate whether or not two or more expressions evaluate a certain way.
Let’s explore a few examples to illustrate how these logical operators work. We’ll return to our online shopping example from earlier.
Python and Operator
The and operator evaluates to True if all expressions specified evaluate to True.
Suppose we are building an online shopping site. Our site should check to make sure a user is over 16, and it should also make sure a user has an account in good standing. To do so, we could use this code:
age = 17 good_standing = True if (age >= 16) and (good_standing == True): print("This user's account can make a purchase.") else: print("This user's account cannot make a purchase.")
Our code returns: This user’s account can make a purchase.
In our code, we have used an and
statement to evaluate whether a user is aged 16 or over, and to evaluate whether a user’s account is in good standing. In this case, age >= 16
is evaluated, then good_standing == True
is evaluated. Since both these statements evaluate to True, the contents of our if
statement are executed.
If either of these conditions evaluated to False—if a user was under 16, or had an account that was not in good standing—then the contents of our else
statement would have been executed.
Python or Operator
The or operator evaluates to True if at least one expression evaluates to True.
Suppose we want to give all shoppers a 5% discount who are subscribed to our loyalty plan, and give a discount to all people aged 65 or over who are making a purchase. To do so, we could use the following program:
loyalty_plan = False age = 67 if (loyalty_plan == True) or (age >= 65): discount = 5 else: discount = 0 print("Shopper discount: ", discount)
Our code returns: Shopper discount: 5.
In this case, our code evaluates whether loyalty_plan
is equal to True, and it also evaluates whether age
is equal to or greater than 65. In this case, loyalty_plan is not equal to True, so that statement evaluates to False. But, age is greater than 65, and so that statement evaluates to True.
Because we specified an or
statement in our code and one of our conditions evaluated to True, the contents of our if
statement were run. However, if our user was not on our loyalty plan and was under the age of 65, the contents of our else
statement would be run.
In this case, our user was granted a discount of 5%. Then, the message Shopper discount:
, followed by the size of the user’s discount, was printed to the console.
Python not Operator
The not operator evaluates to True only if an expression evaluates to False.
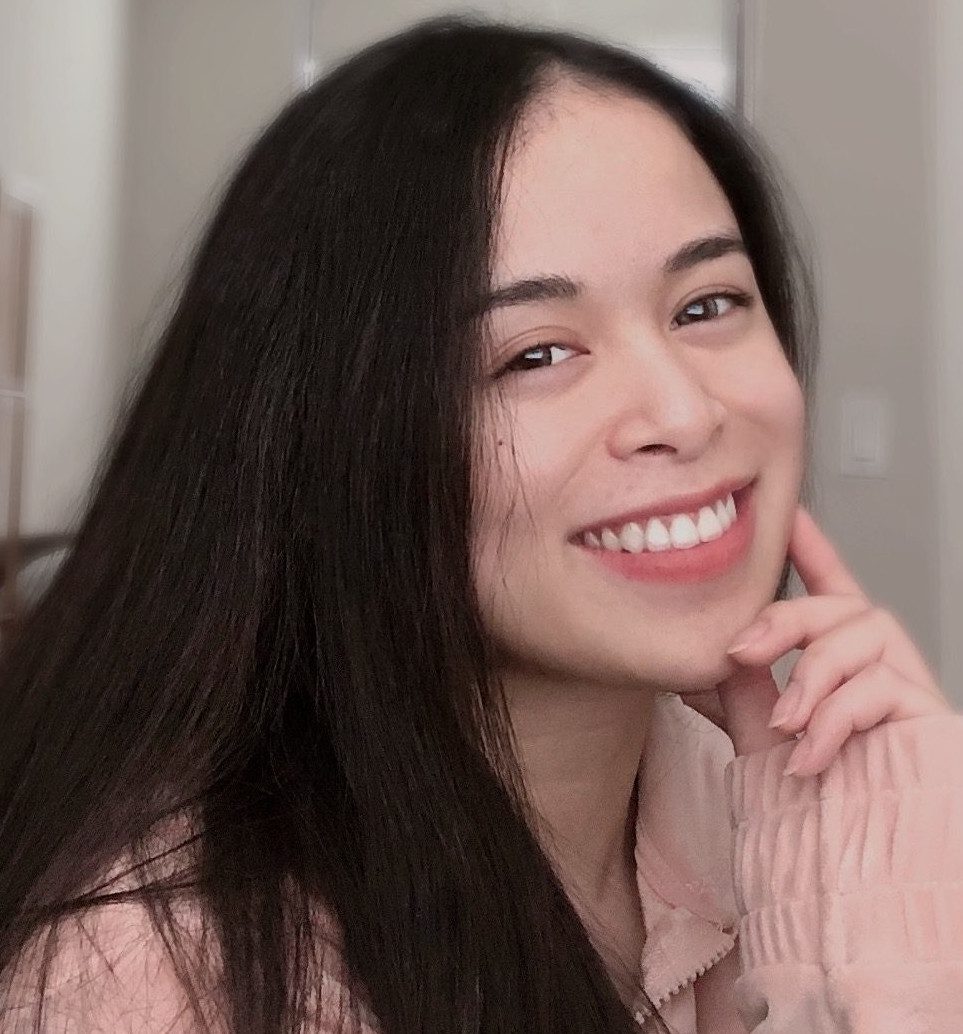
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Suppose our discounts are only to be used one time, and we only want to offer a discount only to customers who have not already made a purchase using their discount. We could do so using this code:
used_discount = True if not(used_discount == True): print("This user has not used their discount.") else: print("This user has used their discount.")
Our code returns: This user has not used their discount.
In our code, we used a not statement to evaluate whether the statement used_discount == True
evaluates to False. In this case, because the statement evaluates to True, the statement not
evaluates to False. This results in the code in our else
block being executed.
If our user did not use their discount, used_discount == True
would have evaluated to False, so our not
statement would evaluate to True, and the contents of our if
statement would have been executed.
Conclusion
Logical operators allow you to control the flow of your program.
The and logical operator allows you to check if two expressions are True, the or logical operator allows you to check if one of multiple expressions are True, and the not operator allows you to check if an expression is False.
With the examples provided in this article, you’re equipped with everything you need to start using logical operators in your Python code like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.