Lists are a built-in data structure in Python 3. Python Lists can be used to organize and store data in an ordered way, such as names of suppliers for a manufacturing company, salary information for a company’s employees, a list of student grades, or anything else.
There are a number of built-in functions in Python that we can use to change or manipulate data that is stored in lists.
In this guide, we are going to break down some of the most useful built-in methods that can be used to work with lists. We’ll explore how to add and remove elements from a list, count the elements in a list, reverse a list, and more.
List Refresher
Lists are a data type in Python that are mutable, ordered arrangements of items. This means that lists can be changed, and store data in a specific order.
Each element within a list is called an item
, and lists are defined by comma-separated values enclosed within square brackets ([]
).
Here’s how to create a list in Python:
news_sites = ["New York Times", "Washington Post", "CNN", "BuzzFeed"]
In order to print out our list, we can use the print()
function:
print(news_sites)
Our program returns our original array as we defined it:
['New York Times', 'Washington Post', 'CNN', 'BuzzFeed']
In order to retrieve a specific element from a Python list, we must reference the index of the element. Each item in a list has an index number, starting with 0
, which allows us to retrieve the contents of an individual element. Here are the index values for the list we created above:
New York Times | Washington Post | CNN | BuzzFeed |
0 | 1 | 2 | 3 |
Now that we know the index numbers of our list elements, we can retrieve a specific value from our list. To do so, we can use the following code:
print(news_sites[0])
Our code returns: New York Times
. Similarly, if we referenced the list item with the index value of 2
, our code would return CNN
.
Each item in our list also has a negative index number. This allows us to count backward on a list and is useful if our list contains a large number of values and we want to retrieve a value toward the end of that list.
Here are the negative index values for our list above:
New York Times | Washington Post | CNN | BuzzFeed |
-4 | -3 | -2 | -1 |
If we wanted to get the item at the index position -1
, we could use this code:
print(news_sites[-1])
Our code returns: BuzzFeed
.
Now that we have covered the basics of Python lists, we can start to explore Python’s list methods.
Add Elements Using list.append()
The list.append()
method allows you to add an item to the end of a list. Here’s an example of a list that stores pizza flavors:
flavors = ["Ham and Pineapple", "Cheese", "Mexican", "Chicken", "Pepperoni"]
Our list consists of five values, starting with the index value 0
, and ending with the index value 4
. But what if we want to add a meat-lovers option to our list? We can use the append()
method to perform this action.
Here’s an example of append()
being used to add Meat-Lovers
to our list:
flavors.append("Meat-Lovers") print(flavors)
Our code returns our revised list with Meat-Lovers
at the end:
['Ham and Pineapple', 'Cheese', 'Mexican', 'Chicken', 'Pepperoni', 'Meat-Lovers']
Insert into List Using list.insert()
The append()
method allows you to add an item to the end of a list, but if you want to add an item at a specific index position, the insert()
method will insert an item at any place in a list.
Let’s say that our pizza chef has been cooking up an in-house special with Jalapeno peppers and is ready to add it to the menu. The chef wants this pizza to come at the top of our list of flavors, to encourage new customers to try out the pizza.
We can use the insert()
method to accomplish this goal. The insert()
method takes two arguments: the index position at which you would like to add the item and the item you want to add to your list. Here’s an example of insert()
being used to add Jalapeno Special
to the start of the flavors list:
flavors.insert(0, "Jalapeno Special") print(flavors)
Our code returns the following list:
['Jalapeno Special', 'Ham and Pineapple', 'Cheese', 'Mexican', 'Chicken', 'Pepperoni', 'Meat-Lovers']
As you can see, Jalapeno Special
was added to the start of our list, and was assigned the index number 0
. Every other item in our list moved up, and so they are given new index numbers corresponding to their new position in the list. Ham and Pineapple
, for example, now has the index value 1
, and so on.
Remove From List Using list.remove()
In order to remove an item from a Python list, we can use the list.remove()
method. This method removes the first item from a list whose value is equal to the value we specify.
After a week of trialing the Jalapeno Special
, it has been made clear that the pizza kitchen’s customers are not in favor of the flavor. So the chef would like to remove it from the menu while he tinkers with the recipe. We could use the following code to remove Jalapeno Special
from our list:
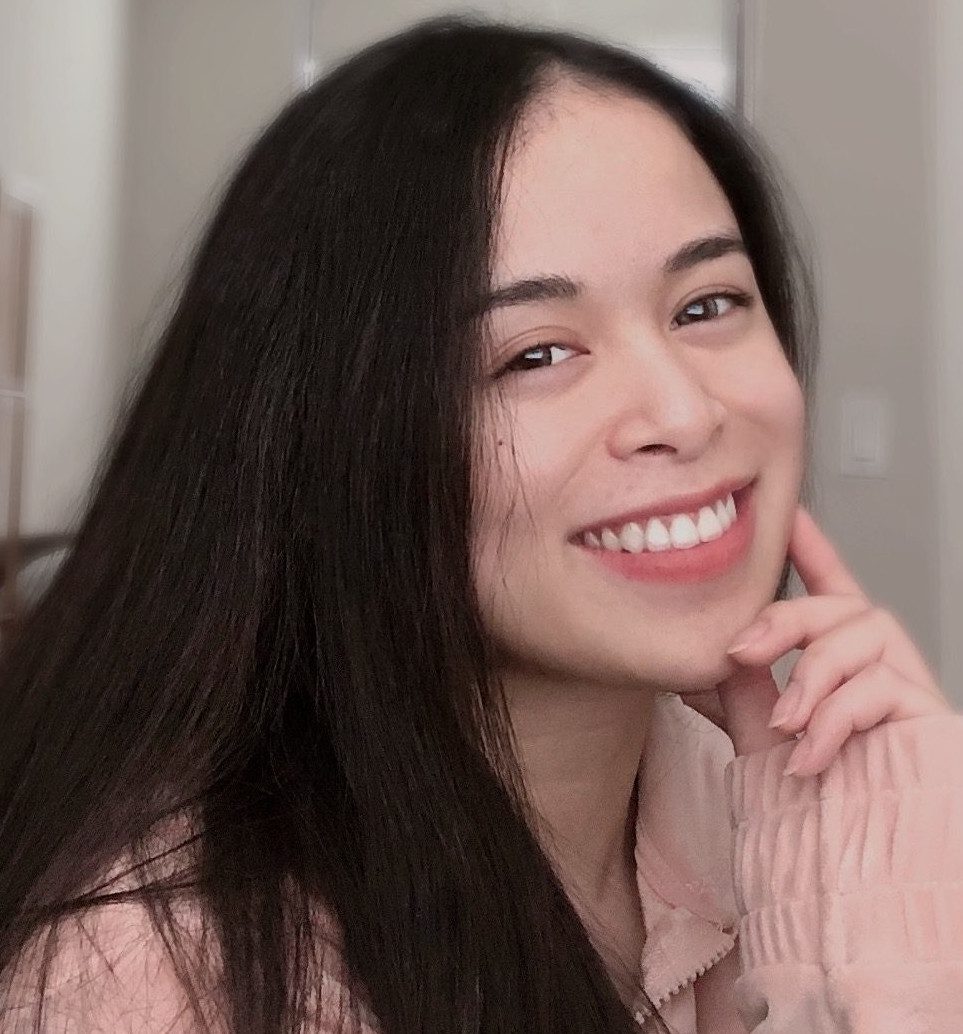
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
flavors.remove("Jalapeno Special") print(flavors)
Our code returns a revised list without Jalapeno Special
:
['Ham and Pineapple', 'Cheese', 'Mexican', 'Chicken', 'Pepperoni', 'Meat-Lovers']
There are a few things you should know about the remove()
method. Firstly, if you try to remove an item that does not exist within a list, an error not in list.
will appear. Secondly, the remove()
method only removes the first instance of a list item. If you had two Jalapeno Specials
in your list, you would have to run the remove()
method twice to remove them both.
Remove from List Using list.pop()
The list.pop()
method can be used to remove and return an item from a list.
The pop()
method takes one optional argument: the index value of the element we want to remove from our list. If we don’t specify an index, pop()
will return and remove the last item in our list.
Our pizza kitchen has decided that the Meat-Lovers
pizza should be moved to the special menu, which includes the chef’s new range of experimental pizzas. So the chef wants to remove Meat-Lovers
from the list of flavors for pizzas.
Here’s the code we would use to remove Meat-Lovers
(which has the index value 5
, from our list):
flavors = ["Ham and Pineapple", "Cheese", "Mexican", "Chicken", "Pepperoni", "Meat-Lovers"] print(flavors.pop(5)) print(flavors)
Our code returns:
Meat-Lovers ['Ham and Pineapple', 'Cheese', 'Mexican', 'Chicken', 'Pepperoni']
As you can see, the pop()
function removed the value we specified and returned the value of the list item we removed, which in this case was Meat-Lovers
. Then, we printed out the new list, which no longer includes Meat-Lovers
.
Combine Multiple Lists Using list.extend()
The list.extend()
method can be used to combine multiple lists in Python. The extend()
method takes one argument: the list object you want to add to an existing list.
Our pizza chef has decided that the special menu should be merged with the standard menu because customers have reported being confused with the two separate menus. In order to merge both our menus, we would use the following code:
flavors = ["Ham and Pineapple", "Cheese", "Mexican", "Chicken", "Pepperoni"] special_menu = ["Meat-Lovers", "BBQ Chicken", "Sausage and Pepperoni", "Vegetarian Special", "Greek"] flavors.extend(special_menu) print(flavors)
Our program returns the following:
['Ham and Pineapple', 'Cheese', 'Mexican', 'Chicken', 'Pepperoni', 'Meat-Lovers', 'BBQ Chicken', 'Sausage and Pepperoni', 'Vegetarian Special', 'Greek']
As you can see, our flavors
list now includes items from both our old flavors list, and our special_menu
list.
Copy a List Using list.copy()
The list.copy()
method allows you to create a copy of a list. This can be useful if you want to manipulate a list but keep the original list intact, in case you need to reference it again.
Our pizza chef has decided to experiment with the menu even further, adding two new pizzas to the menu. However, he wants to keep a list of the original menu for reference.
We could use the following code to create a copy of the menu, which he can add his new pizzas to while preserving the original list:
new_menu = menu.copy() print(menu)
Our code returns:
['Ham and Pineapple', 'Cheese', 'Mexican', 'Chicken', 'Pepperoni', 'Meat-Lovers', 'BBQ Chicken', 'Sausage and Pepperoni', 'Vegetarian Special', 'Greek']
Now, we have two lists with the same values: flavors
and new_menu
. So, the chef can alter the new_menu
, while still keeping the flavors
list intact.
Sort a List Using list.sort()
The list.sort()
method can be used to sort items in a list.
Our chef has decided that our list should be sorted in alphabetical order, to make it easier for people to find the pizza they are looking for. The following code uses sort()
to arrange our list in alphabetical order:
flavors.sort() print(flavors)
Our code returns:
['BBQ Chicken', 'Cheese', 'Chicken', 'Greek', 'Ham and Pineapple', 'Meat-Lovers', 'Mexican', 'Pepperoni', 'Sausage and Pepperoni', 'Vegetarian Special']
You can also use the sort()
function on a list of numbers, too, which will be arranged in ascending order.
Reverse a List Using list.reverse()
The list.reverse()
method can be used to reverse the order of items in a list.
This function does not sort a list in descending order but instead flips the order of every item in our list. So the last item in our list becomes the first item in our new list, and the second-last item becomes the second item, and so on.
Our pizza chef has decided that he wants the list of pizzas to be arranged in descending order, because he has noticed a growth in the number of sales from the bottom of the menu. Here’s the code we would use to reverse our list:
flavors = ['BBQ Chicken', 'Cheese', 'Chicken', 'Greek', 'Ham and Pineapple', 'Meat-Lovers', 'Mexican', 'Pepperoni', 'Sausage and Pepperoni', 'Vegetarian Special'] flavors.reverse() print(flavors)
Our code returns the following:
['Vegetarian Special', 'Sausage and Pepperoni', 'Pepperoni', 'Mexican', 'Meat-Lovers', 'Ham and Pineapple', 'Greek', 'Chicken', 'Cheese', 'BBQ Chicken']
As you can see, Vegetarian Special
was the last item in our original list, and now it is the first—our list has been reversed.
Clear a List Using list.clear()
The list.clear()
method allows you to remove all values within a list.
The new month has come upon the pizza kitchen, and so the chef wants to clear the list of sales for January and start a new list for February. Here’s the code that could be used to clear our sales
list to make room for February:
sales.clear() print(sales)
Our code returns a list with no values: []
.
Conclusion
Lists are mutable, ordered arrangements of items that can be used to store similar data. In this tutorial, we have broken down some of the most common Python list methods, which can be used to manipulate our lists and gather insights about the data in our list.
We covered how to use append()
, insert()
, remove()
, pop()
, extend()
, copy()
, sort()
, reverse()
, and clear()
list methods in this article, and explored examples of each method in action.
Now you’re ready to work with Python list methods like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.