A Python list comprehension is a way of creating a list based on an existing list. List comprehensions are commonly used to filter items out of a list or to change the values in an existing list. List comprehensions are enclosed within square brackets.
When you’re working with lists, you may want to create a list based on the contents of an existing sequence. For example, you may want to create a list based on a sequence of characters. Or you may want to a list that multiplies the contents of another list by two.
That’s where list comprehensions come in. This tutorial will explore, with examples, the basics of Python lists, and how to use list comprehensions.
Python Lists: A Refresher
The list data structure allows you to store collections of items in Python. Lists are commonly used when you want to work with multiple values that are related in some way.
For instance, you could use a list to store all the flavors of ice cream sold in an ice cream store. Or you could use a list to store a list of the phone numbers of members at a wine club.
Here’s an example of a list in Python:
pizzas = ['Chicken', 'Margherita', 'Chicken and Bacon', 'Vegan Special', 'Spinach and Brie', 'BBQ Chicken']
Now that we’ve revised the basics of lists, we can start talking about how to use list comprehensions.
Python List Comprehension
A Python list comprehension creates a new list from the contents of another list. You can use a list comprehension to duplicate a list or to modify the contents of an existing list into a new list. Or you can transfer the contents of another iterable into a list with a list comprehension.
You can specify filters so that your new list only includes certain values. For instance, you could create a new list from a list of numbers. Your new list may only include numbers greater than 250.
The syntax for a list comprehension in Python is as follows:
[expression for item in list]
This syntax is similar to a Python for statement. But, the statement is on one line. To distinguish between a for statement, a list comprehension is enclosed within square brackets.
There are three parts to the syntax above:
- expression: The end value that goes into the new list.
- item: The individual item in the list over which you iterate.
- list: The list or iterable object through which the list comprehension traverses (acceptable data types include tuples, strings, and lists).
You can use a list comprehension with a Python if…else statement inside:
[expression for item in list if condition else none]
This comprehension adds items to the list only if they meet the specified condition.
Using list comprehensions lets you to create a new list from an existing one without defining full for statements. for statements take up at least two lines of code whereas you can write a list comprehension on one line.
Some people say that list comprehensions are more Pythonic code. This is because they are more efficient than using a short for statement.
Python List Comprehension Example
Suppose we want to make a list of all the chicken pizzas we sell at our store. We’re going to move all chicken pizzas into a Chicken category on our menu. To do so, we could filter our pizzas using for statements. Or, we could filter our list using a list comprehension.
We could use a list comprehension to generate a new list of pizzas whose names contain Chicken based on our existing list of pizzas. We could do so using this code:
pizzas = ['Chicken', 'Margherita', 'Chicken and Bacon', 'Vegan Special', 'Spinach and Brie', 'BBQ Chicken'] chicken_pizzas = [p for p in pizzas if "Chicken" in p] print(chicken_pizzas)
Our comprehension returns the following output list:
[‘Chicken’, ‘Chicken and Bacon’, ‘BBQ Chicken’]
In our code, we first define a list of pizzas on our menu. Our pizzas are stored in the Python variable called pizzas. Then, we use a list comprehension to create a new list of pizzas whose names contain Chicken.
Our list comprehension consists of the following parts:
- p: This is the value to be added to our list.
- for p in pizzas: This loops through every pizza in our “pizzas” list.
- if “Chicken” in p: This checks to see if each pizza contains “Chicken”. If this evaluates to True, the value stored in “p” is added to our list.
Our list comprehension only took up one line of code. Whereas in our next example, we need to use three lines of code with a for statement to make it work. Our next example shows the extent to which list comprehensions help you write cleaner code.
Without a List Comprehension
Let’s return to the chicken pizza example. Without using a list comprehension, if we wanted to generate a list of chicken pizzas on our menu, we would use this code:
pizzas = ['Chicken', 'Margherita', 'Chicken and Bacon', 'Vegan Special', 'Spinach and Brie', 'BBQ Chicken'] chicken_pizzas = [] for pizza in pizzas: if "Chicken" in pizza: chicken_pizzas.append(pizza) print(chicken_pizzas)
Our code returns:
[‘Chicken’, ‘Chicken and Bacon’, ‘BBQ Chicken’]
First, we define two lists. One list stores a list of pizzas sold at our store. The other list stores a list of all the chicken pizzas we sell. The list of chicken pizzas we sell is initially empty.
We use a “for” statement to go through each pizza in our list of pizzas. Then, we check to see if each pizza contains the word Chicken. If a pizza contains the word Chicken, we add that pizza to our list of chicken pizzas. In this case, three pizzas contain Chicken, and all three of those pizzas are added to our chicken_pizzas list.
As you can see, to check if a pizza contains Chicken and to add it to our list, we use three lines of code. There is a more efficient way to write this code: using list comprehensions.
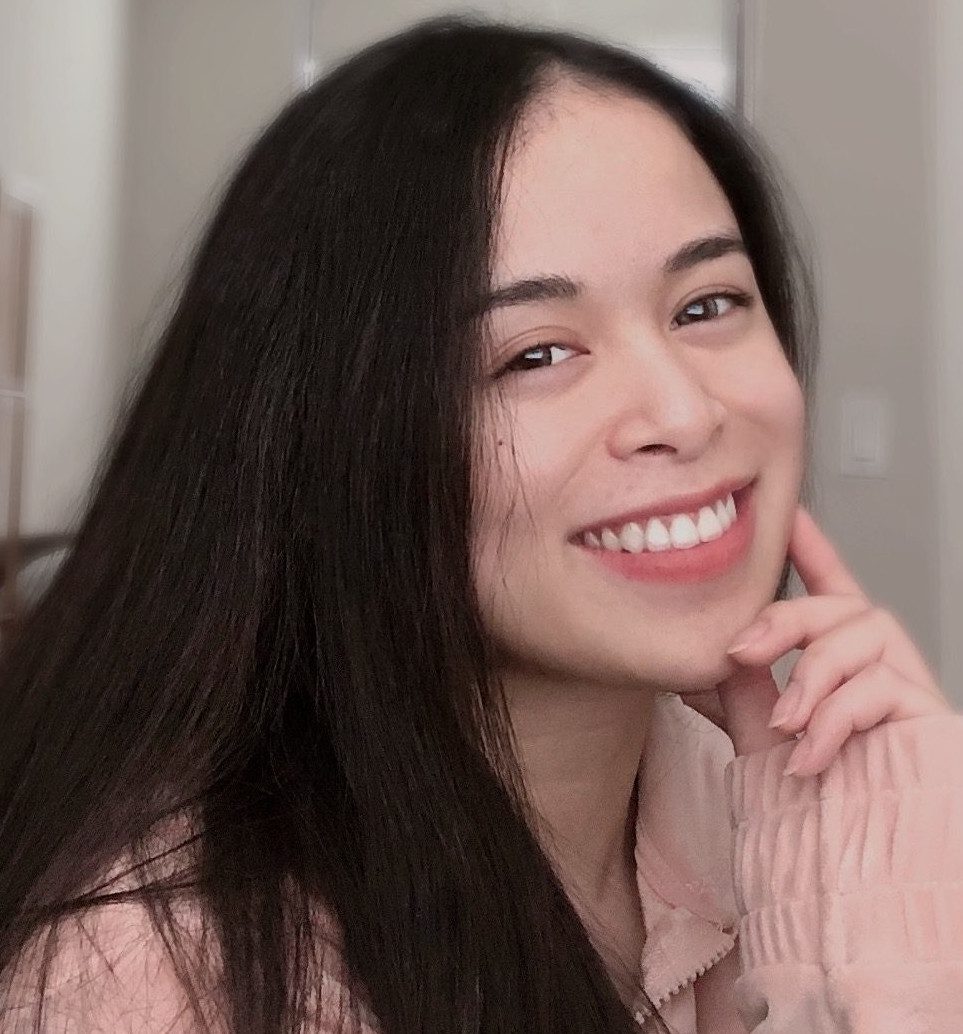
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
List Comprehensions Using if…else
You can also use an if…else statement with a list comprehension.
Earlier, we used an if statement to add a pizza to our list of chicken pizzas. We only added a pizza if the name of the pizza included the term Chicken. But, suppose we are making a list of which pizzas are vegetarian.
We want to add “Meat” to a list if a pizza name contains Chicken and Vegetarian if a pizza name does not contain Chicken.
This can be done using a list comprehension:
pizzas = ['Chicken', 'Margherita', 'Chicken and Bacon', 'Vegan Special', 'Spinach and Brie', 'BBQ Chicken'] is_veggie = ["Meat" if "Chicken" in p else "Vegetarian" for p in pizzas] print(is_veggie)
Our code returns:
[‘Meat’, ‘Vegetarian’, ‘Meat’, ‘Vegetarian’, ‘Vegetarian’, ‘Meat’]
Let’s break down our code. First, we declare a list of pizzas called pizzas. Then, we use a list comprehension to assess whether a pizza name contains Chicken or not.
If a pizza name contains Chicken, the value Meat is added to our is_veggie list. Otherwise, the value Vegetarian is added.
As you can see, the first value in our is_veggie list is Meat, because its corresponding value in the pizzas list is Chicken. But, our next value is Vegetarian, because its corresponding value in the pizzas list is Margherita, which does not contain Chicken.
Conclusion
List comprehensions allow you to create a new list based on an existing list. Using list comprehensions, you can create a copy of a list, or create a new list that filters out values from an old list.
This tutorial discussed, with reference to examples, the basics of Python lists and how to use the list comprehension technique to create new lists.
Do you want to learn more about coding in Python? Check out our How to Learn Python guide. This guide contains a list of learning resources to help you continue your journey toward mastering Python.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.