Python Lambda functions are single line, anonymous functions. Lambda expressions in Python are useful for single line functions that do not need a name, such as filtering lists, converting string cases, or multiplying a number.
When you’re writing a program that will perform a similar task multiple times, it’s impractical to repeat your code as it would mean you’d have to update every instance of it if you needed to change how the task works. The larger your codebase is, the longer it will take to make these changes.
That’s where functions come in handy. In Python, there’s a special type of function called an anonymous function which allows you to declare quick functions: the lambda expression.
This guide will discuss how to use lambda functions in Python to automate repetitive processes. We’ll also provide two examples of a lambda function in action so you’ll have all the materials you need to write your own.
What is a Function?
In programming, a function is a block of code that performs a specific task.
One big reason why coders use functions is that they help reduce repetition in their code. If you bundle up a process into a function, you can call it anywhere in your program. You don’t need to repeat your code multiple times.
This also helps developers write more maintainable code. If you need to make a change to that process, all you need to do is modify your function. You don’t need to make changes to multiple lines of code which have just been copied and pasted in your program.
Here’s an example of a function in Python:
def say_hello(name): print("Hello there, " + name) say_hello("Arthur")
When we run this code, the following is returned: Hello there, Arthur.
While these functions are useful, if you want to do something simple like make every string in a list of strings lowercase, writing a function is quite verbose. You’d have to assign a name to a new function in order for your code to work. That’s where lambda functions come in.
What is a Lambda Function?
A lambda function is a type of function that is defined without a name. These functions are sometimes called anonymous functions, because they don’t need a name. Whereas our function from earlier had the name sayHello, and was defined using “def”, a lambda function does not need a name and is defined using “lambda”.
The main advantage of using a lambda function is that it can be written on one line. This makes lambda functions useful if you have a quick process you want to perform multiple times. Here are a few instances where a lambda function may be helpful:
- Multiplying a number
- Converting a string to upper or lowercase
- Filtering a list
Here’s the syntax for creating a lambda function in Python:
name = lambda arguments: expression
The number of arguments you can specify with a lambda function is not limited. Let’s walk through an example to illustrate how a lambda function works in Python. Suppose we want to multiply a number by seven. To do so, we could use the following function:
def multiply_by_seven(number): return number * 7 print(multiply_by_seven(2))
When we run this code, the following is returned: 14.
While this code does work, it’s not as efficient as it could be. We spend an entire line of code defining the name and arguments for our function and another line of code calculating and returning a value. To make our function more efficient, we could use a lambda function.
Here’s a lambda function that performs the same action as above:
multiply_by_seven = lambda n: n * 7 print(multiply_by_seven(2))
Our code returns: 14. As you can see, the output of our function is the same. The difference is that we’ve used a lambda function instead of a regular function. In this case:
- The lambda keyword indicates that we are declaring a lambda function
- n is the argument for our function.
- n * 7 is the single expression specified to our lambda function.
When Are Lambda Functions Used?
Lambda functions have a wide range of uses in Python. In our last example, we demonstrated how you could use a lambda function to multiply two numbers. But, there are a few more common use cases of which you should be aware.
Often, lambda functions are used alongside built-in functions such as the reduce()
function, the map()
function or the filter()
function. Lambda functions are sometimes used with list comprehensions to perform repetitive tasks, too.
Let’s say we own a bakery and we want to create a list of all the pies we sell based on a list of all the baked goods we sell. We could do so using this code:
baked_goods = ['Blondie', 'Chocolate Chip Cookie', 'Apple Pie', 'Pecan Pie', 'Blackberry Pie', 'Scone'] pies = filter(lambda pie: "Pie" in pie, baked_goods) print(list(pies))
Our code returns:
['Apple Pie', 'Pecan Pie', 'Blackberry Pie']
Let’s break down how this worked. On the first line of our code, we declared a list of baked goods. Then, we used the filter()
function to filter out all of the baked goods which contain the word “Pie”. The filter()
function allowed us to iterate through every item in the “baked_goods” list and filter out items that did not meet a particular criteria.
We did this by defining a lambda function which checks if each string contains “Pie”, and only returns the string if that string contains “Pie”. In this case, our lambda function is:
lambda pie: "Pie" in pie
You can see from this example that Python lambda functions do not need a name. They can exist in-line, unlike traditional functions. If an item meets our criteria, our Lambda expression returns true; otherwise, no value is returned.
Finally, we convert the variable “pies” to a list and print it to the console. We do this because filter()
returns a custom filter object, so in order to see our pies, we need to convert it using list()
.
Conclusion
Lambda functions, also known as anonymous functions, allow you to define a function without a name. They are useful if you need to perform an action multiple times in your code.
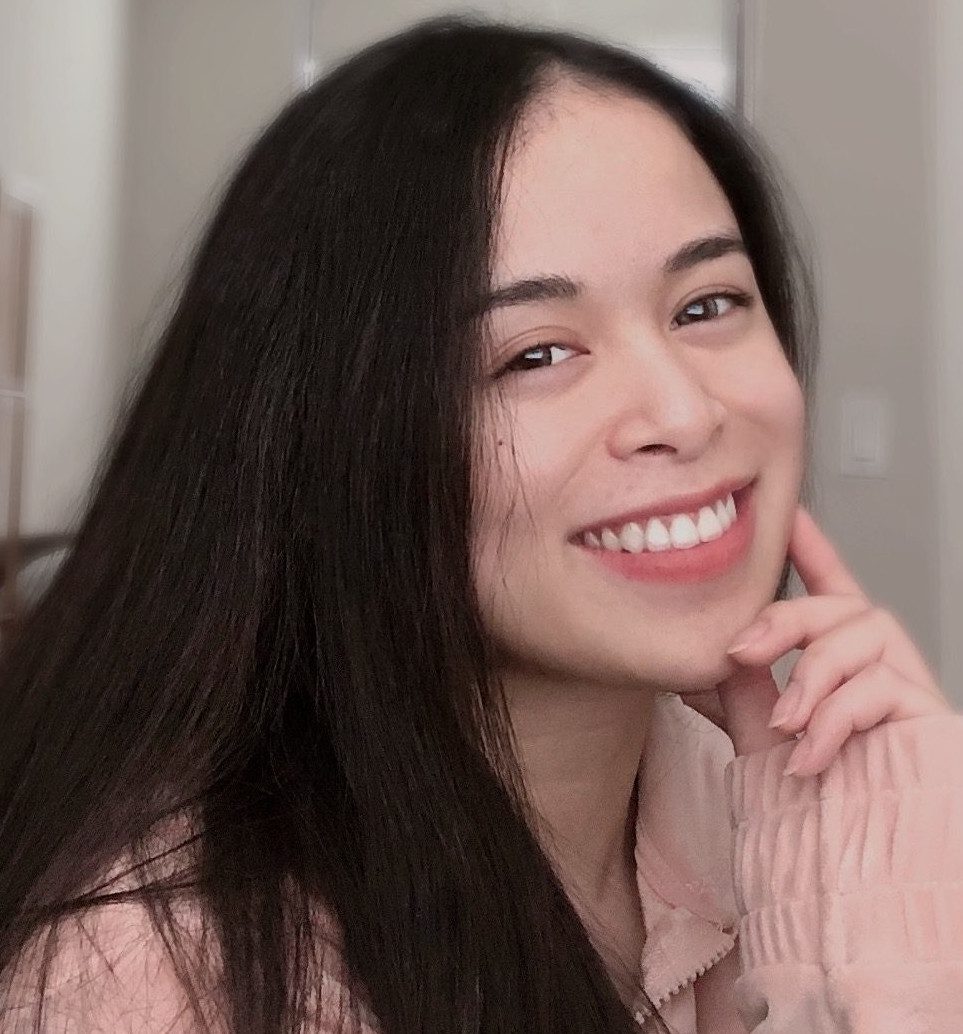
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
For instance, if you need to multiply a number by another number, you may use a lambda function; or you could use a lambda function to filter out items in a list.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.