JSON is a popular data format used for storing structured data.
When you’re building an application in Python, you may decide that you want to use JSON in your app. For instance, you may want to read data from a JSON file, or write data stored in the JSON format to a file.
In this guide, we’re going to break down the basics of the JSON data format, how to use the Python json module, and how to work with JSON in Python. By the end of reading this tutorial, you’ll be an expert at using JSON in your Python programs!
What is JSON?
JSON, which is short for JavaScript Object Notation, is a data format that allows you to store structured data.
Often, JSON is used to send data to and from a server in a web application. You may have seen, for instance, that many APIs such as the Fitbit API, or the Google Maps API return data in the JSON format
This is because JSON data is standardized, structured, and easy to read.
Here is an example of a JSON record in Python:
{ "name": "James Smith", "id": 202, "probation": False }
This record stores three keys, which are on the left side of the colons (:), and three values, which are stored on the right side of the colons. Each key is bound to a value.
The JSON data format should not be confused with a dictionary. While this may look just like a dictionary, JSON is a data format, whereas a dictionary is a data structure.
This means that, if you want to store your JSON data in a dictionary, you will need to convert it to a dictionary; if you want to store a dictionary as JSON, you’ll need to convert it to JSON.
Import json in Python
Before you start working with JSON objects in Python, you’ll need to import the Python json module. This module includes a number of functions that allow you to work with JSON data.
To import the JSON module, you can use this statement:
import json
Now that we’ve imported the JSON module into your code, we can start working with its functions.
Parse JSON in Python
One of the most important functions you’ll need to perform when it comes to working with JSON data is parsing JSON into a dictionary.
To parse JSON into a dictionary, you can use the json.loads()
method.
json.loads()
accepts one parameter: the JSON string you want to convert to a dictionary.
Suppose you have a JSON string that stores information about the projects a coder is assigned to on an engineering team. You want to convert that JSON string into a dictionary. To do so, you could use this code:
import json employee = "{'name': 'Linda Richardson', 'projects': ['Directory', 'Homepage']}" employee_dictionary = json.loads(employee) print(employee_dictionary) print(employee_dictionary["name"])
Our code returns:
{‘name’: ‘Linda Richardson’, ‘projects’: [‘Directory’, ‘Homepage’]}
Linda Richardson
Let’s break down our code. First, we import the json module into our code. Then, we declare a string called employee
which stores our employee record in a JSON structure.
Next, we use json.loads()
to convert our employee
string to JSON. Then, we print our new dictionary to the console. We also print the value of the name
key to the console.
As you can see, our dictionary looks the same as our JSON string. But, now our data is stored as a dictionary. We can tell because, when we print out the value of the “name” key, “Linda Richardson” is printed to the console.
Convert Dictionary to JSON String
When you’re working with a dictionary, you may want to convert it into a JSON string.
This is a common operation because, because you save a JSON value to a file, it needs to be formatted as a string. To convert a dictionary to JSON, we can use the json.dumps()
method.
Suppose we want to convert a dictionary with information about an employee to a JSON string. We could do so using this code:
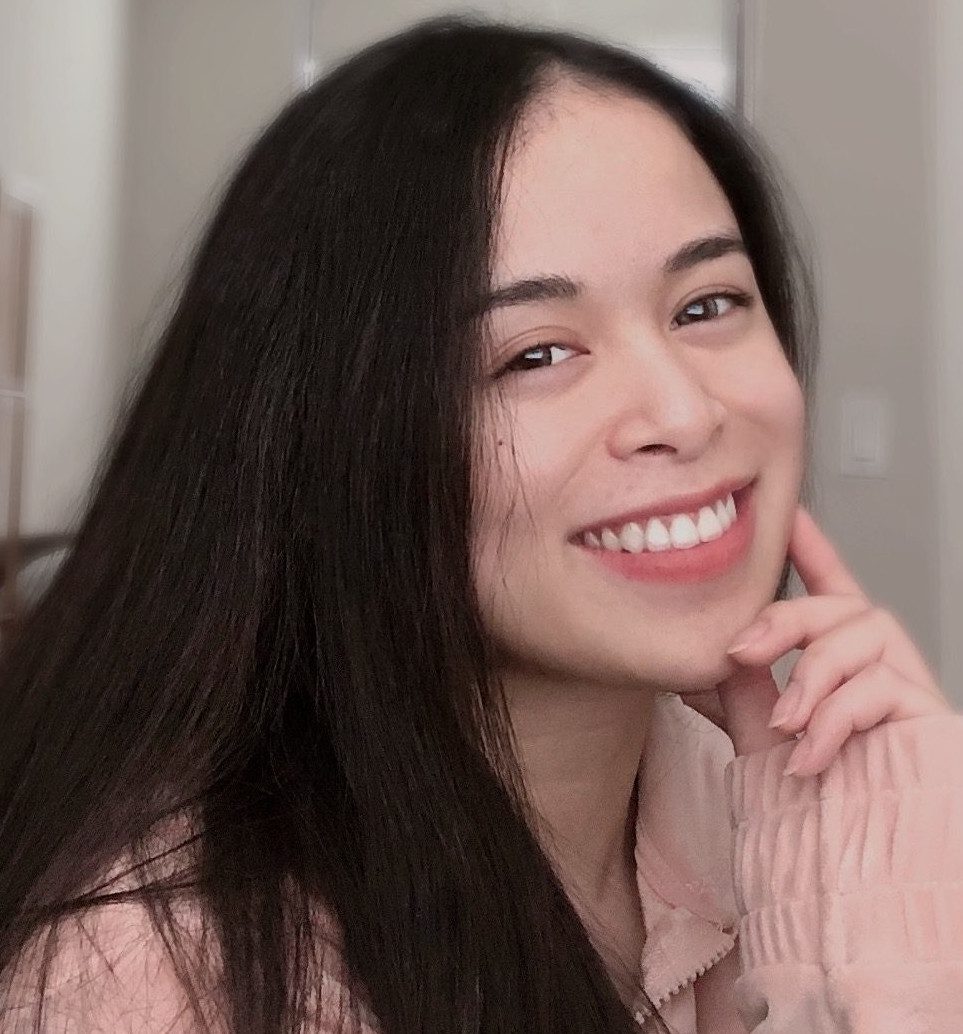
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
import json employee = { "name": "Linda Richardson", "id": 107, "probation": False, "department": "Sales" } employee_json = json.dumps(employee) print(employee_json)
Our code returns:
‘{“name”: “Linda Richardson”, “id”: 107, “probation”: false, “department”: “Sales”}’
While the output of our code may look just like our original dictionary, our dictionary is now stored as a string.
Formatting a JSON String
The json.dumps()
method comes with a few parameters that you can use to make it easier to read the final string created by the method.
The indent
parameter allows you to define the number of indents that appear in the final JSON string. Suppose you want to add four indents to each line on your JSON string. You could do so using the following json.dumps()
statement:
employee_json = json.dumps(employee, indent=4)
This statement, when combined with our last example, would return:
{ "name": "Linda Richardson", "id": 107, "probation": false, "department": "Sales" }
You can also change the separators of the data in the JSON string. The default values for the separators are “, ” and “: ”, which means that every object will be separated using a comma, and every key and value will be separated using a colon.
Suppose we want our keys and values to be separated using an equals sign (=), and we want to use an indent of 4. We could do so using this statement:
employee_json = json.dumps(employee, indent=4, separators=(", ", " = "))
Our code returns:
{ "name" = "Linda Richardson", "id" = 107, "probation" = false, "department" = "Sales" }
As you can see, every key and value in our JSON string is now separated using an equals sign.
Read a JSON File
To read a JSON file in Python, you can use json.load()
. This method accepts one parameter: the file object that you want to read in your program.
Suppose we have a file called employee.json that we want to load into our program. The contents of this file are as follows:
{"name": "Linda Richardson", "id": 107, "probation": false}
We could do so using this code:
{"name": "Linda Richardson", "id": 107, "probation": false} We could do so using this code: import json with open('employee.json') as final_file: employee = json.load(final_file) print(employee)
Our code returns:
{“name”: “Linda Richardson”, “id”: 107, “probation”: false}
In this code, we first import the json library. Then, we use a with
statement to read our employee.json
file. We use the json.load()
method to convert the contents of our file, stored in the file
variable, to a dictionary. Then, we print out the value of our dictionary.
Write JSON to a File
You can use the json.dump()
method to write JSON to a file in Python.
The json.dump()
method accepts two parameters: the dictionary you want to write to a file, and the file object that you want to write your dictionary.
Suppose we want to save our employee’s record as a JSON value in a file. The name of this file should be linda_employee.json
. We could do so using the following code:
import json employee = { "name": "Linda Richardson", "id": 107, "probation": False, "department": "Sales" } with open('linda_employee.json', 'w') as final_file: json.dump(employee, final_file)
Let’s break down our code. First, we import the json module into our program. Then, we declare a variable which stores information about an employee called Linda Richardson in a dictionary structure.
Next, we use a “with” statement with the “w” flag to prepare a file called linda_employee.json
to which we can write. We then use json.dump()
to convert our employee
dictionary to a JSON string and to save it to our final_file
object.
When this program is run, the contents of our employee
dictionary are written to the linda_employee.json
file. The final contents of this file are:
{"name": "Linda Richardson", "id": 107, "probation": false, "department": "Sales"}
Conclusion
The Python json module allows you to read and manipulate JSON data.
Using the json module, you can:
- Read JSON from a file
- Convert a dictionary to JSON
- Convert JSON to a dictionary
- Write a JSON string to a file
In this tutorial, we explored how to perform all these operations using the json module. Now you’re ready to start working with JSON data in Python like a professional!
Are you interested in learning how to code in Python? Download the free Career Karma app today to unlock top learning resources and to discover training programs that can help you master Python.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.