The isinstance()
function in Python returns true or false if a variable matches a specified data type. The syntax for isinstance()
in Python is isinstance(variable_to_check, data_type)
.
Checking the data type of a particular value is a common operation in programming. For example, you may want to check whether the value you stored in a variable is a string or a number since each of these data types works differently in code.
That’s where the isinstance()
function can be useful. isinstance()
is a built-in Python method that allows you to verify a particular value’s data type. For example, you can use isinstance()
to check if a value is a string or a list.
This tutorial will walk you through how to use the isinstance()
method in Python and will provide a few examples to help you better understand it.
Python isinstance Overview
When you code in Python, you use various types of data. These data types include strings, numbers, lists, tuples, and dictionaries. Every data type has its own set of rules that governs how that data is stored and manipulated. For example, when using Python, you can perform mathematical calculations on numbers but not on strings.
As a result, it’s important that you are working with the right data types in your program. You can use isinstance in Python to verify that the data you are working with is stored as the appropriate data type. When using Python, check data types as needed.
isinstance()
is a function built into Python that checks whether a value is stored as a particular data type. Unlike the type()
method, which is discussed later in this article, the isinstance()
method returns only True
or False
, depending on whether the value you are checking is stored as the data type or types you specify.
The syntax for isinstance()
is as follows:
isinstance(object, data_types)
The isinstance()
method takes in two parameters, both of which are required:
- object: the object whose data type you want to check.
- data_types: at least one of the possible data types of the object.
The isinstance()
method then compares the object against the data type(s) you specified. If you want to compare the object against two or more data types, you’ll need to specify a tuple as the data_types parameter.
In response, your code returns True
or False
. The code returns True
if the object belongs to the data type (or one of the data types) you specify, and False
if not.
Essentially, when you use isinstance in Python, you are presenting your code with a fill-in-the-blank statement and at least one answer choice. Your statement is: This object is a (data type)
. Specifying the data type parameter completes that statement. Two examples are:
12
is a number.books
is a number.
In response, your code returns True
or False
.
Python isinstance Examples
Let’s run through two examples to show how you can use the isinstance()
method in your code. Say that we have a value, and we want to verify that the value is a string. We could use isinstance()
to do this. Here’s the code we could use:
print(isinstance("String", str))
Our code performs a type check and then our function returns: True
.
Because String
is actually a string (str
is how you refer to the data type string
in Python) our program returns True
.
Now we’ll explore a more detailed example.
Let’s say that we are building a multiplication game for a second-grade class. Our game presents a user with a math problem, and then it checks the user’s answer to see whether they are correct. In order for our program to work, we need to make sure that the user has entered a number into our game.
To check if the answer we collected from the player is a number, we could use this code:
answer = 5 * 8
user_answer = input("What is 5 x 8?")
print(isinstance(user_answer, (int, float)))
Our code returns: False
.
Let’s break down our program. On the first line, we calculate the answer to the math problem that we presented to the player. In this case, that math problem was: What is 5 x 8?
, so we input answer = 5 * 8
for the first line of our code.
Then, we use the input()
method to retrieve an answer from the user.
On the final line of our code, we use isinstance()
to check whether our user’s answer (stored in user_answer
) is either an integer or a float, which are the two data types used to represent numbers in Python. We do this by creating a tuple that stores the data types against which we want to check our value. In our code, this tuple is: (int, float)
.
Our code returns False
, which tells us that the user’s answer is neither an integer (int) nor a float.
Now that we know that our object is not an instance of int or float, we can start to diagnose the problem. Our code returns False
because input()
gives us a string by default, even if a user enters a number. So, for our code to work, we need to convert the result of our input()
method into an integer. We can do so using the following code:
answer = 5 * 8
user_answer = int(input("What is 5 x 8?"))
print(isinstance(user_answer, (int, float)))
Now when we run our code, we get: True
.
The only difference between this example and the previous one is that, in this code, on the user_answer
line, we convert the result of our input()
method to an integer by using int()
. Therefore, the program registers the input as an integer rather than a string. So, when we check this value’s data type using the isinstance()
method, our program verifies that the value is either an integer or a float.
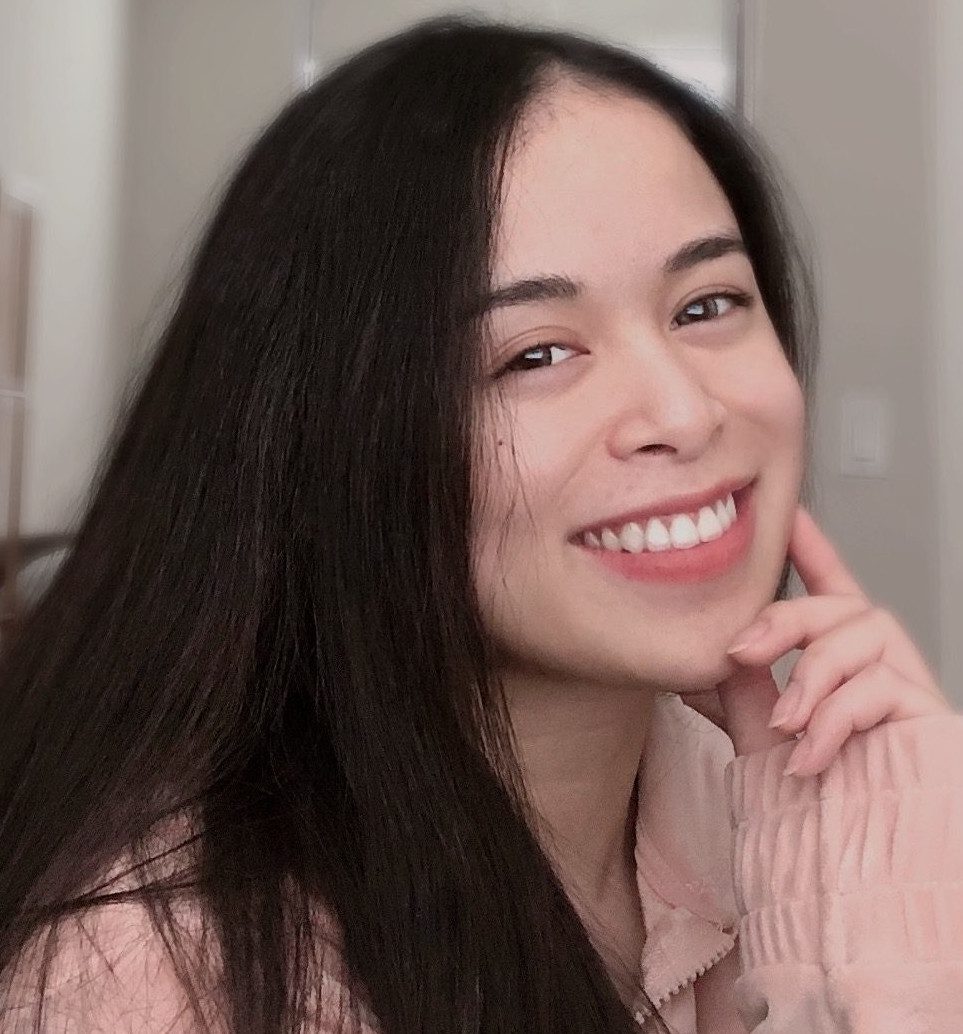
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
In the above example, we checked whether an object held a particular built-in data type. The isinstance()
method can also be used to compare an object with a specific class.
type vs. isinstance
If you’ve ever needed to check the type of a value in Python, you may have come across the type()
method. type()
is a built-in Python function that can be used to find out the type of a variable or value. Here’s the syntax for the Python type method:
type(data)
For example, let’s say you have a number and you want to check to see what data type it is coded as. You could do so using the following code:
number = 8
print(type(number))
Our code returns:
<class 'int'>
As you can see, our program returns the type of our data. It tells us that this data is stored as an integer.
There is one big difference between the type()
and isinstance()
functions that makes one more appropriate to use than another in certain circumstances. If you want to see a value’s data type, type()
may be more appropriate. But, if you want to verify that a value is stored as a certain data type or types—such as a string or a float—you should use isinstance()
.
Conclusion
In Python, you can use the isinstance()
function to verify whether a value holds a particular data type. For example, if you want to verify that a list of values is stored as a list, or if a number is stored as a float, you can use isinstance()
.
This Python tutorial demonstrated how to use isinstance()
to verify whether a value holds a certain data type. Now you have the information you need to use isinstance()
like a Python master.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.