The Python insert() method adds an item to a list. This method accepts two arguments: the position at which you want to add the item, and the item you want to add.
Suppose you have a list of student names, and a new student joins your class. To keep your list up to date, you would want to add the new student’s name to your list of existing class members.
That’s where the Python insert() method comes in. The insert() method allows you to add an element into a list at a given index position.
This tutorial will discuss how to use the Python insert() method to add an element to a list at a specific position. We’ll walk through an example to help you get started with this method.
Python Lists: A Refresher
A list is a type of data that can store one or more items in an order. For instance, a list could be used to store the names of all books in a library. Or a list could store the phone numbers of everyone who has made a reservation at a restaurant.
Here is an example of a list in Python:
birds = ["Robin", "Collared Dove", "Great Tit", "Goldfinch", "Chaffinch"]
Each item in our list has its own index number, starting from 0, which we can use to access items individually in our list. So, Robin has the index number 0, Collared Dove has the index number 1, and so on.
Python insert() Method
The Python insert() method adds an element to a specific position in a list. insert() accepts the position at which you want to add the new item and the new item you want to add as arguments.
The syntax for the insert() method is as follows:
list_name.insert(index, element)
We can see this method accepts two parameters. The parameters for the insert() method are:
- index: The position where an element should be inserted.
- element: The item you want to add to your list.
This method is similar to the Python append() method, which also adds an item to a list. However, while append() adds an item to the end of a list, insert() adds an item to a list at a specific position.
insert() Python Example
Let’s suppose that our list from earlier stores an account of all the birds we have seen in our garden this year. After a birdwatching session, we have spotted a new bird: the Starling. We want to add this bird to our list, at the very start.
To do so, we could use the following code:
birds = ["Robin", "Collared Dove", "Great Tit", "Goldfinch", "Chaffinch"] birds.insert(0, "Starling") print(birds)
Our code returns:
[‘Starling’, ‘Robin’, ‘Collared Dove’, ‘Great Tit’, ‘Goldfinch’, ‘Chaffinch’]
First, we declare a Python array called birds. This array stores a list of the birds we have spotted.
We use the insert() method to add the Starling to our list of birds. Because we want to add our bird to the start of our list, we specify the index parameter 0. This adds the value Starling to the start of our list.
We print out the updated contents of our list using the print() function.
Add a List to a List
In the above example, we added a string to our list. But, we could use the insert() list method to add any type of data instead.
Suppose we have a list that stores two lists. One of the lists stores the birds we have seen, and the other list stores the birds we want to see.
We want to add a new list which lists the birds we think we have seen. This list should store the birds where we have had no confirmation from someone else that we have actually seen a bird. But, we might have seen them.
To add our list of unconfirmed sights to a list, we could use this code:
birds = [ ["Robin", "Collared Dove", "Great Tit", "Goldfinch", "Chaffinch"], ["Dunnock", "Long-tailed Tit", "Greenfinch"] ] list_to_add = ["Song Thrush", "Grey Tit"] birds.insert(1, list_to_add) print(birds)
Our code returns:
[[‘Robin’, ‘Collared Dove’, ‘Great Tit’, ‘Goldfinch’, ‘Chaffinch’], [‘Song Thrush’, ‘Grey Tit’], [‘Dunnock’, ‘Long-tailed Tit’, ‘Greenfinch’]]
First, we declare a Python variable called birds which stores a list. This list contains two lists. The first list stores a list of birds that we have seen, and the other stores the list of birds we want to see.
We have declared another list that stores the names of all the birds we think we have seen, but we are not sure about. Next, we use the insert() method to add this list to the index position 1 in our birds list.
The contents of the variable list_to_add were added at index position 1. This means the new list came after index position 0. So, the new list is the second list in our list of lists.
Similarly, you can use the insert() method to add numbers, booleans, Python tuples, and other data types to a list.
Conclusion
The Python insert() method adds item to a list at a specific position in a list. insert() accepts two arguments. These are the position of the new item and the value of the new item you want to add to your list.
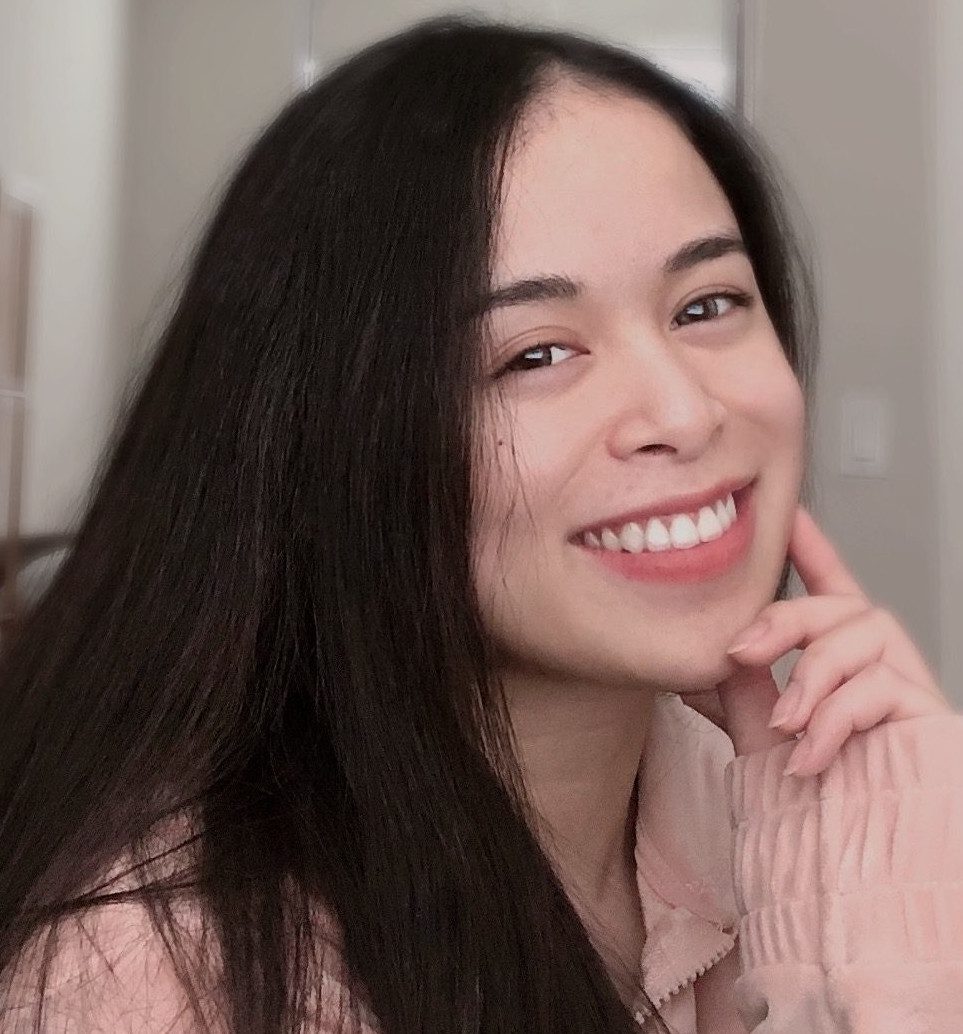
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Like the append() method, insert() adds an item to a list. But, you cannot specify the position of the new item using the append() method.
This tutorial discussed, with reference to examples, the basics of Python lists and how to use the insert(). Now you’re ready to start using the insert() method like a Python pro!
Do you want to learn more about coding in Python? Check out our How to Learn Python tutorial. This guide contains a list of top courses, learning resources, and books you can use to build your Python knowledge.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.