The Python input() and raw_input() functions are used to collect user input. input() has replaced raw_input() in Python 3 and onward. Both functions return a user input as a string.
Processing user input is a crucial part of programming.
For example, you may want to ask a user their age so you can determine whether they should be allowed to use your site. Or you may want to ask a user to input their name so you can determine its length. Whatever data you need from a user, you’ll need to find a way to get it in some fashion.
That’s where the Python input() function comes in. Input(), a built-in function in Python, allows coders to receive information through the keyboard, which they can process in a Python program. In this tutorial, we are going to break down the basics of Python input().
Python User Input: A Guide
Accepting user input lets you retrieve values from the user while your program is running. This means that you do not need to depend on pre-existing values or the contents of a file in order to run your program.
Python 3.x uses a method called input() to retrieve user input. In Python 2.x, you must use the raw_input() method.
When we call an input function, our program will pause. The program resumes after the user enters the text through the Python shell or command line. For example, we may ask a user for their email address.
Our program will pause until a user inserts text into the program and presses the enter key. The user must press the enter key. This key tells Python the user has finished inserting text on the shell.
Python input() in Version 3
The input() function allows a user to insert a value into a program. input() returns a string value. You can convert the contents of an input using any data type. For instance, you can convert the value a user inserts to a floating-point number. input() is supported in Python 3.x.
The input() function works in the same way as the raw_input() function. input() uses the following syntax:
input(message)
The message is the string that you want to display when a user is prompted to input text. This message should tell a user that they are expected to perform an action. Otherwise, a user get confused at why the program is not continuing.
Let’s ask the user to insert their email address into the shell:
email = input("Enter your email address: ") print("To confirm, is your email address:", email)
We have used the input() method to ask a user to enter their email address. The message we enclosed in brackets is displayed to the console on the same line a user is expected to input text.
We store the value the user inserts in a variable. This lets us refer back to the user’s input later in our program.
Here is the result of our code:
Enter your email address: python3@test.com To confirm, is your email address: python3@test.com
Our user was first asked to insert their email. Then, our program printed out a message which used the value the user inserted. This means that the value the user inserted was successfully stored in our program.
Python raw_input() in Version 2
raw_input() accepts user input. The raw_input() method is the Python 2.x equivalent of Python 3’s input().
The raw_input() function will prompt a user to enter text into the program. That data is collected the user presses the return key.
raw_input() takes one parameter: the message a user receives when they are prompted for input. This is the same as the input() method we discussed earlier.
Here is an example of the Python raw_input() function in action which uses the input string Enter your email address:
email = raw_input("Enter your email address: ") print "To confirm, is your email address:", email
Our program asks us to enter our email address. After we enter a value, we hit enter. This lets us submit the email address into our program. The email address is saved in the email variable for later.
When we run our code and give it our email address, our prompt will be printed like this:
Enter your email address: test@test.com To confirm, is your email address: test@test.com
Our program successfully stores the input value we have retrieved from the user.
If you try to use raw_input() in Python 3, you’ll encounter the “NameError: name ‘raw_input’ is not defined” error. Click here to read our guide on how to fix this error.
Input Types
By default, the input() and raw_input() functions will convert the data a user enters into a string. If you want to work with a string, this is not a problem. But if you’re going to work with an integer or another data type, you’ll need to change it manually.
To do this, you should use the built-in Python data functions to change the type of data you have. Here’s an example of a program that will prompt a user for their age, add one year onto their age, and returns the value:
user_age = input("Enter your age: ") final_user_age = int(user_age) + 1 print("On your next Birthday, you'll be:", final_user_age)
We convert the user’s age to an integer using the int() function. Then, we add 1 to that age. On the final line of our code, we allow our value to print on the screen, alongside a short message. Here’s our code in action:
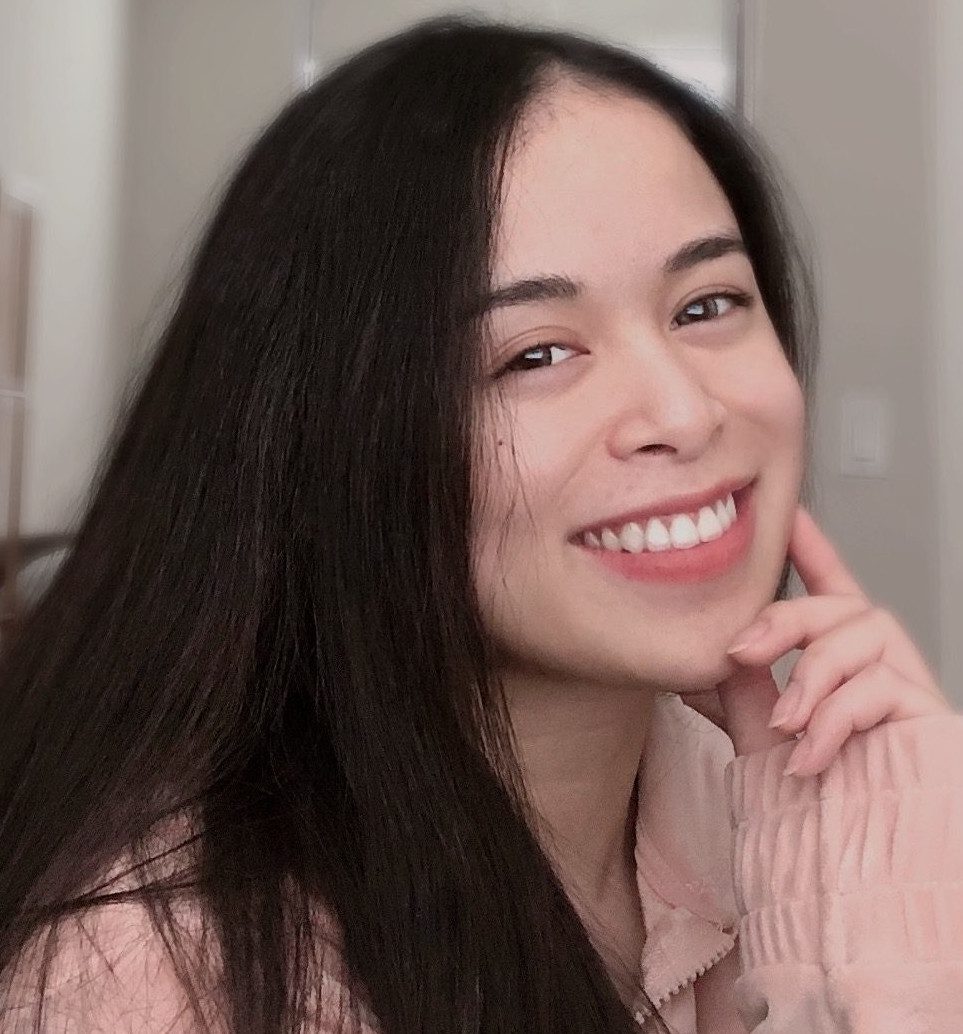
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Enter your age: 19 On your next Birthday, you'll be: 20
Alternatively, we could wrap our input() statement with the int() function. This will convert our value to a number immediately after the value has been retrieved. Here’s an example:
user_age = int(input("Enter your age: "))
We have converted the age value into an integer.
Conclusion
The input() and raw_input() functions have a wide array of uses in Python. If you’re looking to get data from a user, you’ll need to use these functions at some point.
In this tutorial, we have broken down how the input() and raw_input() functions work in Python. Now you’re an expert on collecting user input in Python! To learn more about programming in Python, read our How to Code in Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.