IndexErrors are one of the most common types of runtime errors in Python. They’re raised when you try to access an index value inside a Python list that does not exist. In most cases, index errors are easy to resolve. You just need to do a little bit of debugging.
In this tutorial, we’re going to talk about the “indexerror: list index out of range” error. We’ll discuss how it works and walk through an example scenario where this error is present so that we can solve it.
The Problem: indexerror: list index out of range
As always, the best place to start is to read and break down our error message:
indexerror: list index out of range
This error message tells us that we’re trying to access a value inside an array that does not have an index position.
In Python, index numbers start from 0. Here’s a typical Python array:
programming_languages = ["Java", "Python", "C++"]
This array contains three values. The first list element, Java, has the index value 0. Each subsequent value has an index number 1 greater than the last. For instance, Python’s index value is 1.
If we try to access an item at the index position 3, an error will be returned. The last item in our array has the index value 2.
Example Scenarios (and Solutions)
There are two common scenarios in which the “list index out of range” error is raised:
- When you try to iterate through a list and forget that lists are indexed from zero.
- When you forget to use range() to iterate over a list.
Let’s walk through both of these scenarios.
Lists Are Indexed From Zero
The following program prints out all the values in a list called “programming_languages” to the Python shell:
programming_languages = ["Java", "Python", "C++"] count = 0 while count <= len(programming_languages): print(programming_languages[count]) count += 1
First, we have declared two variables. The variable “programming_languages” stores the list of languages that we want to print to the console. The variable “count” is used to track how many values we have printed out to the console.
Next, we have declared a while loop. This loop prints out the value from the “programming_languages” at the index position stored in “count”. Then, it adds 1 to the “count” variable. This loop continues until the value of “count” is no longer less than or equal to the length of the “programming_languages” list.
Let’s try to run our code:
Java Python C++ Traceback (most recent call last): File "main.py", line 5, in <module> print(programming_languages[count]) IndexError: list index out of range
All the values in our list are printed to the console but an error is raised. The problem is that our loop keeps going until the value of “count” is no longer less than or equal to the length of “programming_languages”. This means that its last iteration will check for:
programming_languages[3]
This value does not exist. This causes an IndexError. To solve this problem, we can change our operator from <= to <. This will ensure that our list only iterates until the value of “count” is no longer less than the length of “programming_languages”. Let’s make this revision:
programming_languages = ["Java", "Python", "C++"] count = 0 while count < len(programming_languages): print(programming_languages[count]) count += 1
Our code returns:
Java Python C++
We’ve successfully solved the error! Our loop is no longer trying to print out programming_languages[3]. It stops when the value of “count” is equal to 3 because 3 is not less than the length of “programming_languages”.
Forget to Use range()
When you’re iterating over a list of numbers, it’s easy to forget to include a range() statement. If you are accessing items in this list, an error may be raised.
Consider the following code:
ages = [9, 10, 9] for age in ages: print(ages[age])
This code should print out all the values inside the “ages” array. This array contains the ages of students in a middle school class. Let’s run our program and see what happens:
Traceback (most recent call last): File "main.py", line 4, in <module> print(ages[age]) IndexError: list index out of range
An error is raised. Let’s add a print statement inside our loop to see the value of “age” in each iteration to see what has happened:
ages = [9, 10, 9] for age in ages: print(age) print(ages[age])
Our code returns:
9 Traceback (most recent call last): File "main.py", line 5, in <module> print(ages[age]) IndexError: list index out of range
The first age, 9, is printed to the console. However, the value of “age” is an actual value from “ages”. It’s not an index number. On the “print(ages[age])” line of code, we’re trying to access an age by its index number.
When we run our code, it checks for: ages[9]. The value of “age” is 9 in the first iteration. There is no item in our “ages” list with this value.
To solve this problem, we can use a range() statement to iterate through our list of ages:
ages = [9, 10, 9] for age in range(0, len(ages)): print(ages[age])
Let’s run our code again:
9 10 9
All of the values from the “ages” array are printed to the console. The range() statement creates a list of numbers in a particular range. In this case, the range [0, 1, 2] is created. These numbers can then be used to access values in “ages” by their index number.
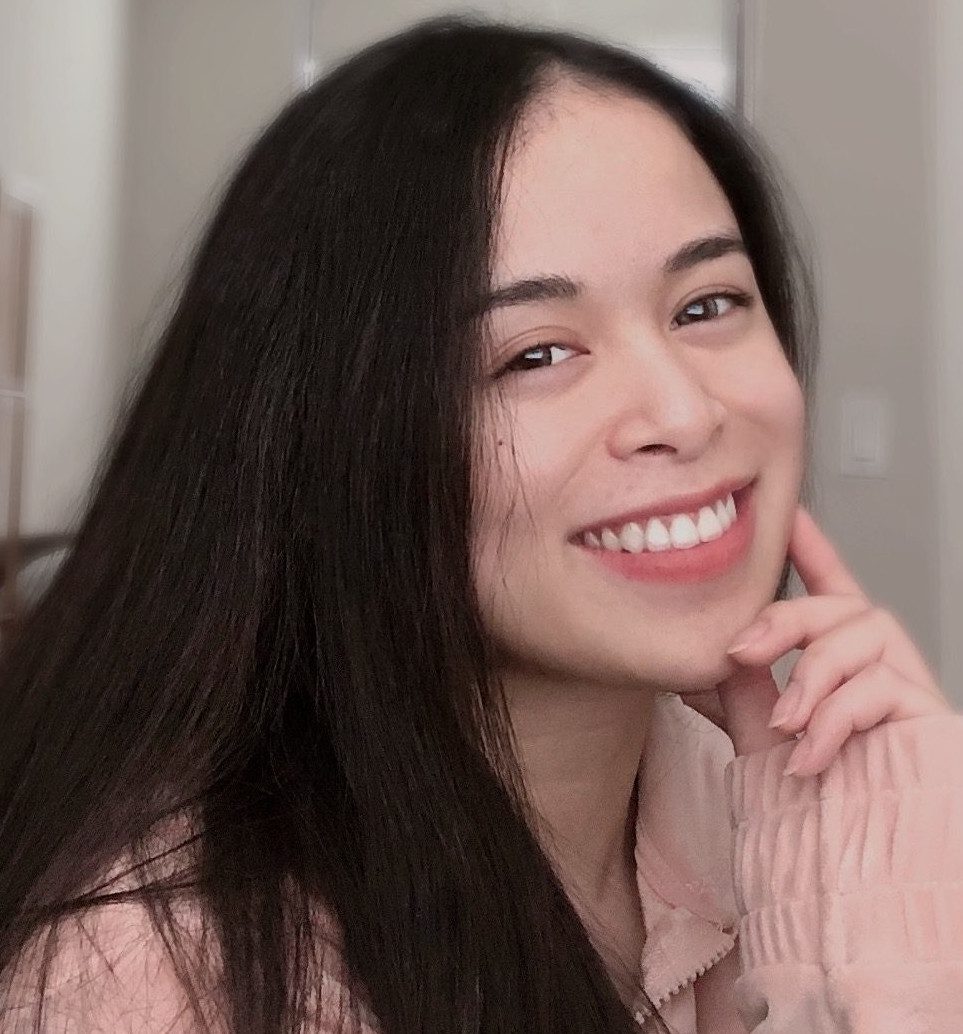
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Alternatively, we could use a “for…in” loop without using indexing:
ages = [9, 10, 9] for age in ages: print(age)
This code returns:
9 10 9
Our code does not try to access any values by index from the “ages” array. Instead, our loop iterates through each value in the “ages” array and prints it to the console.
Conclusion
IndexErrors happen all the time. To solve the “indexerror: list index out of range” error, you should make sure that you’re not trying to access a non-existent item in a list.
If you are using a loop to access an item, make sure that loop accounts for the fact that lists are indexed from zero. If that does not solve the problem, check to make sure that you are using range() to access each item by its index value.
Now you’re ready to solve the “indexerror: list index out of range” error like a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.