An IndexError is nothing to worry about. It’s an error that is raised when you try to access an index that is outside of the size of a list. How do you solve this issue? Where can it be raised?
In this article, we’re going to answer those questions. We will discuss what IndexErrors are and how you can solve the “list assignment index out of range” error. We’ll walk through an example to help you see exactly what causes this error.
Without further ado, let’s begin!
The Problem: indexerror: list assignment index out of range
When you receive an error message, the first thing you should do is read it. An error message can tell you a lot about the nature of an error.
Our error message is: indexerror: list assignment index out of range.
IndexError tells us that there is a problem with how we are accessing an index. An index is a value inside an iterable object, such as a list or a string.
The message “list assignment index out of range” tells us that we are trying to assign an item to an index that does not exist.
In order to use indexing on a list, you need to initialize the list. If you try to assign an item into a list at an index position that does not exist, this error will be raised.
An Example Scenario
The list assignment error is commonly raised in for and while loops.
We’re going to write a program that adds all the cakes containing the word “Strawberry” into a new array. Let’s start by declaring two variables:
cakes = ["Strawberry Tart", "Chocolate Muffin", "Strawberry Cheesecake"] strawberry = []
The first variable stores our list of cakes. The second variable is an empty list that will store all of the strawberry cakes. Next, we’re going to write a loop that checks if each value in “cakes” contains the word “Strawberry”.
for c in range(0, len(cakes)): if "Strawberry" in cakes[c]: strawberry[c] = cakes[c] print(strawberry)
If a value contains “Strawberry”, it should be added to our new array. Otherwise, nothing will happen. Once our for loop has executed, the “strawberry” array should be printed to the console. Let’s run our code and see what happens:
Traceback (most recent call last): File "main.py", line 6, in <module> strawberry[c] = cakes[c] IndexError: list assignment index out of range
As we expected, an error has been raised. Now we get to solve it!
The Solution
Our error message tells us the line of code at which our program fails:
strawberry[c] = cakes[c]
The problem with this code is that we are trying to assign a value inside our “strawberry” list to a position that does not exist.
When we create our strawberry array, it has no values. This means that it has no index numbers. The following values do not exist:
strawberry[0] strawberry[1] …
We are trying to assign values to these positions in our for loop. Because these positions contain no values, an error is returned.
We can solve this problem in two ways.
Solution with append()
First, we can add an item to the “strawberry” array using append():
cakes = ["Strawberry Tart", "Chocolate Muffin", "Strawberry Cheesecake"] strawberry = [] for c in range(0, len(cakes)): if "Strawberry" in cakes[c]: strawberry.append(cakes[c]) print(strawberry)
The append()
method adds an item to an array and creates an index position for that item. Let’s run our code: [‘Strawberry Tart’, ‘Strawberry Cheesecake’].
Our code works!
Solution with Initializing an Array
Alternatively, we can initialize our array with some values when we declare it. This will create the index positions at which we can store values inside our “strawberry” array.
To initialize an array, you can use this code:
strawberry = [] * 10
This will create an array with 10 empty values. Our code now looks like this:
cakes = ["Strawberry Tart", "Chocolate Muffin", "Strawberry Cheesecake"] strawberry = [] * 10 for c in range(0, len(cakes)): if "Strawberry" in cakes[c]: strawberry.append(cakes[c]) print(strawberry)
Let’s try to run our code:
['Strawberry Tart', 'Strawberry Cheesecake']
Our code successfully returns an array with all the strawberry cakes.
This method is best to use when you know exactly how many values you’re going to store in an array.
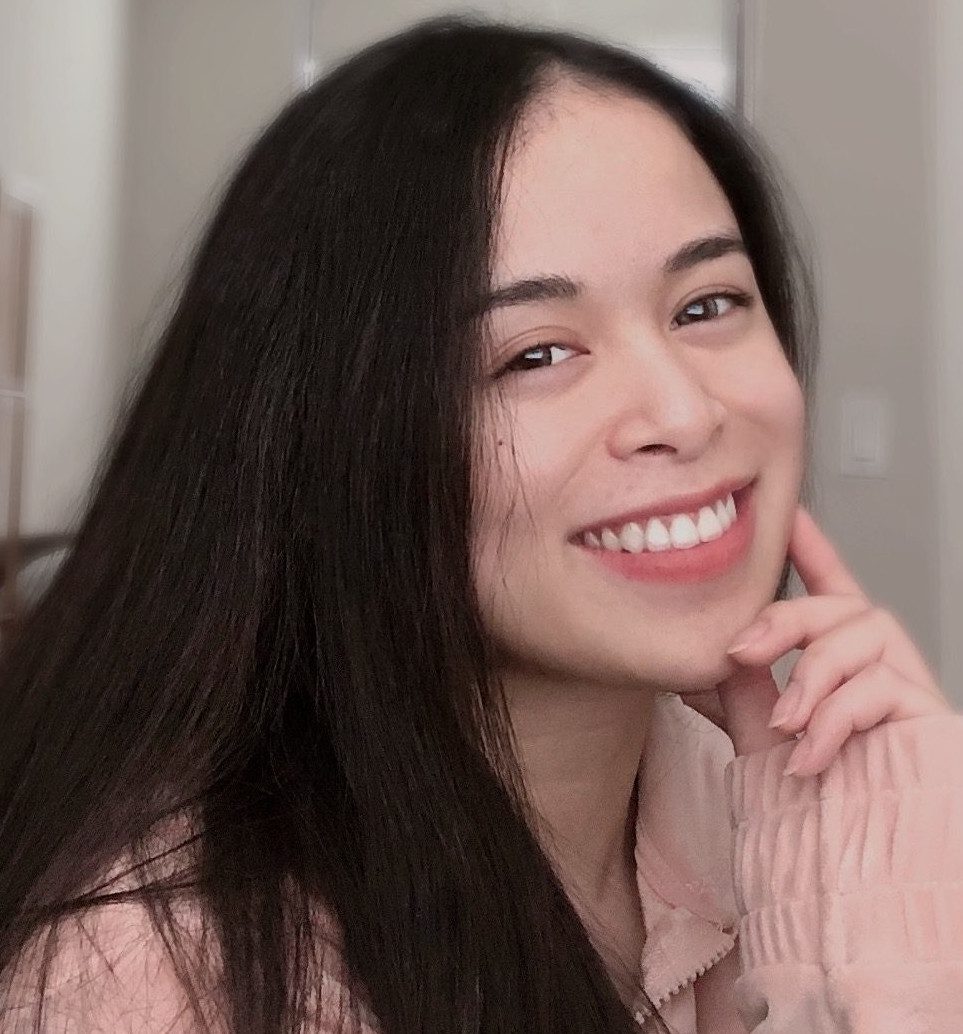
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Our above code is somewhat inefficient because we have initialized “strawberry” with 10 empty values. There are only a total of three cakes in our “cakes” array that could possibly contain “Strawberry”. In most cases, using the append() method is both more elegant and more efficient.
Conclusion
IndexErrors are raised when you try to use an item at an index value that does not exist. The “indexerror: list assignment index out of range” is raised when you try to assign an item to an index position that does not exist.
To solve this error, you can use append()
to add an item to a list. You can also initialize a list before you start inserting values to avoid this error.
Now you’re ready to start solving the list assignment error like a professional Python developer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.