An if else Python statement evaluates whether an expression is true or false. If a condition is true, the “if” statement executes. Otherwise, the “else” statement executes. Python if else statements help coders control the flow of their programs.
When you’re writing a program, you may want a block of code to run only when a certain condition is met. That’s where conditional statements come in. Conditional statements allow you to control the flow of your program more effectively.
In Python, the if and if…else statements are used to perform conditional operations. This tutorial will discuss, with reference to examples, the basics of the if, if…else, and elif statements in Python. We’ll also discuss how to use nested if statements.
Python if Statement
A Python if statement evaluates whether a condition is equal to true or false. The statement will execute a block of code if a specified condition is equal to true. Otherwise, the block of code within the if statement is not executed.
Let’s write a program that prints the price of a sandwich order. The price of a sandwich order should only be displayed if the customer has ordered a ham roll. Our sandwich order is a Python string. Here’s the code for our program:
sandwich_order = "Ham Roll" if sandwich_order == "Ham Roll": print("Price: $1.75")
Our code returns: Price: $1.75
We have declared a variable called sandwich_order. This variable has been assigned the value Ham Roll.
We use an if statement to check whether sandwich_order is equal to Ham Roll. If our condition is true, our print() statement is be executed. If our condition is false, nothing will happen.
Our sandwich_order variable is equal to Ham Roll. This means that our if statement is executed. Now, let’s see what happens when we change our sandwich order to Cheese Roll:
sandwich_order = "Cheese Roll" if sandwich_order == "Ham Roll": print("Price: $1.75")
Our code returns nothing. The print() statement in our code is not given the chance to execute. This is because our sandwich order is not equal to Ham Roll.
if else Python Statement
An if…else Python statement checks whether a condition is true. If a condition is true, the if statement executes. Otherwise, the else statement executes.
So far, we have used an if statement to test for whether a particular condition is met. But, what if we want to do something if a condition is not met?
Suppose we are building an app that checks whether a customer at a local restaurant has run up a tab. If the customer has run up a tab over $20, they need to pay it off before they can order more food. Nothing should happen if the customer does not have a tab accrued over $20.
To accomplish this task, we could use the following code:
tab = 29.95 if tab > 20: print("This user has a tab over $20 that needs to be paid.") else: print("This user's tab is below $20 that does not require immediate payment.")
Our code returns: This user has a tab over $20 that needs to be paid.
Let’s walk through how our code works. First, we declare a Python variable called tab. This variable tracks a customer’s tab. We use an if statement to check whether the customer’s tab is greater than 20.
If a customer’s tab is worth more than $20, the print() statement after our if statement is executed. Otherwise, the print() statement after our Python if…else clause is executed.
Because our customer’s tab is over $20, the Python interpreter executes our if statement. This instructs our program to print a message to the console. The message tells us that the customer must pay their tab.
Let’s set the customer’s tab to $0 and see what happens:
This user's tab is below $20 which does not require immediate payment.
Our code returns a different output. The customer’s tab is not over $20. This means the contents of our else statement are executed instead of our if statement.
Python elif Statement
A Python elif statement checks for another condition if all preceding conditions are not met. They appear after a Python if statement and before an else statement. You can use as many elif statements as you want.
In our above example, we created a conditional statement with two possible outcomes. If the user’s tab was over $20, a message was printed to the console. If a user’s tab was under $20, a different message was printed to the console.
In some cases, we may want to evaluate multiple conditions and create outcomes for each of those conditions. That’s where the elif condition comes in.
elif Python Statement Example
Let’s return to our sandwich example from earlier. Suppose we want to have four potential outputs from our program, depending on the sandwich filling a customer chooses. These are:
- Ham Roll: $1.75
- Cheese Roll: $1.80
- Bacon Roll: $2.10
- Other Filled Roll: $2.00
We could use the following code to calculate the cost of the customer’s order:
sandwich_order = "Bacon Roll" if sandwich_order == "Ham Roll": print("Price: $1.75") elif sandwich_order == "Cheese Roll": print("Price: $1.80") elif sandwich_order == "Bacon Roll": print("Price: $2.10") else: print("Price: $2.00")
Our code returns: Price: $2.10
.
Our code has four possible outcomes:
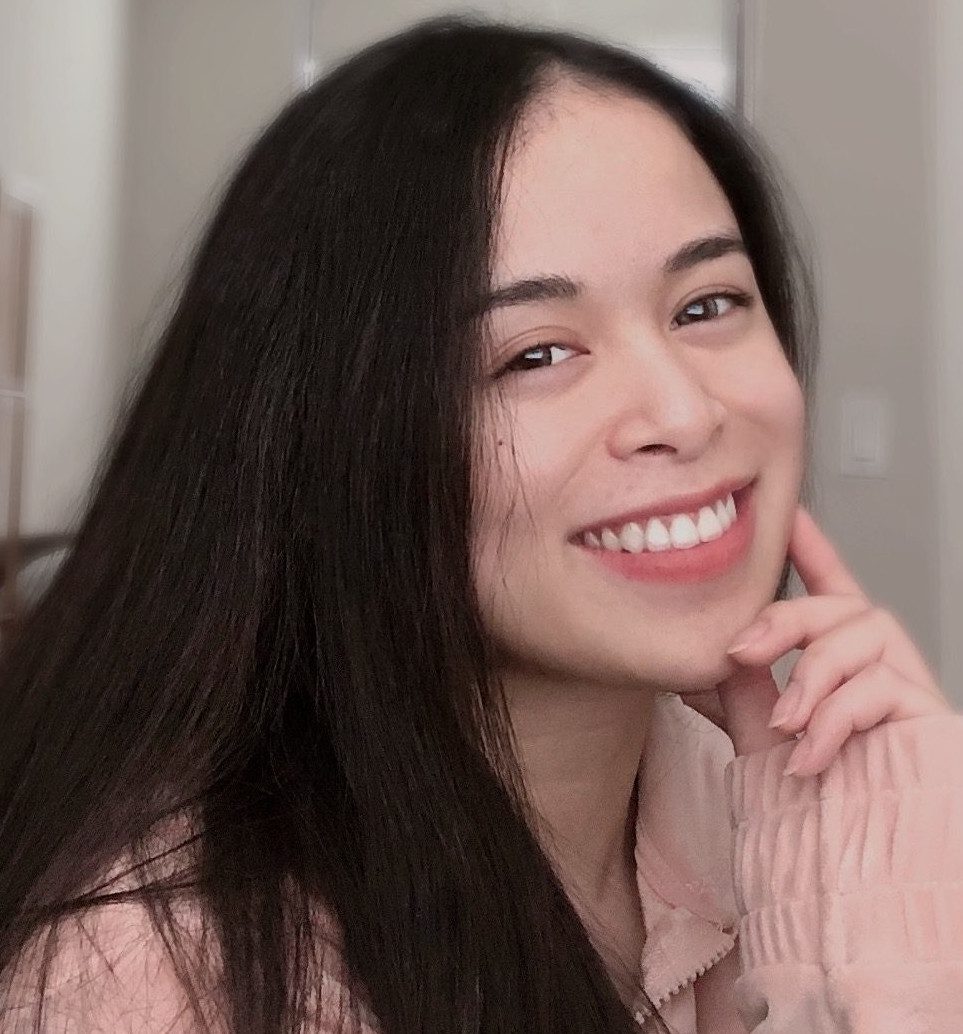
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
- If a customer orders a ham roll, the contents of the “if” statement are executed. This prints “Price: $1.75” to the console.
- If a customer orders a cheese roll, the contents of the first “elif” statement are executed. This prints “Price: $1.80” to the console.
- If a customer orders a bacon roll, the contents of the second “elif” statement are run. This prints “Price: $2.10” to the console.
- We display “Price: $2.10” on the console if a customer orders a roll with a different filling.
We used an if statement to test for a specific condition. Our two elif blocks to test for alternative conditions. The else statement returns a value in case no conditions are met.
We could add in more elif statements to our above code if we wanted. If we introduced a new Tuna Roll to our sandwich menu, we could add in a new elif statement. This new statement could print the price of the new menu item to the console.
Python Nested if Statements
A nested if statement is an if statement inside another if statement. Nested if statements let you check if a condition is met after another condition has already been met.
Let’s return to our sandwich example from earlier. Suppose we want to check whether a customer has ordered a roll that is on our menu. We want to do this before we check the prices of the customer’s order.
Python Nested if Statement Example
A message should be printed to the screen with our default price for non-menu items if a customer has ordered another custom sandwich. Custom sandwiches are sandwiches that are not on our menu (such as a buttered roll, or a jam roll).
However, if a customer has ordered a sandwich that is on our menu, we should then check to see the price of that sandwich. We could do so using this code:
sandwich_order = "Other Filled Roll" if sandwich_order != "Other Filled Roll": if sandwich_order == "Ham Roll": print("Price: $1.75") if sandwich_order == "Cheese Roll": print("Price: $1.80") elif sandwich_order == "Bacon Roll": print("Price: $2.10") else: print("Price: $2.00")
Our code returns: Price: $2.00
.
First, our program evaluates whether our sandwich order is not equal to Other Filled Roll. Our program will compare the sandwich we have ordered with the list of sandwiches on our menu. This happens if we have ordered a sandwich on the menu.
If we have ordered a filled roll that is not on our menu, the contents of the else statement in our code are executed.
In this example, we have ordered a filled roll that is not on our menu. This means that the statement if sandwich_order != Other Filled Roll evaluates to False, so the code in our if statement is executed.
Now, suppose we ordered a ham roll instead. This would cause our first if statement to evaluate to true. This is because Ham Roll is not equal to Other Filled Roll. Our order will be compared to the list of sandwich prices we have specified.
View the Repl.it from this tutorial:
Conclusion
The if else statement lets you control the flow of your programs. First, Python evaluates if a condition is true. If a condition is not true and an elif statement exists, another condition is evaluated.
If no conditions are met and an else statement is specified, the contents of an else statement are run. By using a conditional statement, you can instruct a program to only execute a block of code when a condition is met.
Now you’re ready to start using these statements in your own code, like a Python expert! To learn more about coding in Python, read our complete guide on How to Code in Python.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.