A variable is an important and ubiquitous concept in programming. Variables are containers for storing data. Variables can store many types of data, including usernames, email addresses, and items in a user’s online game inventory.
When programming in Python, you will encounter two types of variables: global and local. In this guide, we discuss the difference between these two types of variables, how they work, and how you can use them in your code.
Python Variables
In Python, variables essentially allow you to label and store data. When defining a variable, you will give it a name. You can then use that name later in your code to retrieve the data it represents. Variables can store strings, numbers, lists, or any other data type.
Suppose we are building a game and want to store a user’s name. Instead of having to type the name throughout our program, we can use a variable to store it.
Here’s a variable that stores the name of a user for a game:
name = "Carlton Hernandez"
We declared a variable called name
and assigned it the value “Carlton Hernandez”.
Now that we declared that variable, we can manipulate it in our code. For example, if we wanted to change our user’s name, we could do so using this code:
name = "Carlton Hernandez" name = "Carlton Lewis Hernandez"
On the first line of our code, we assigned the value “Carlton Hernandez” to the name
variable. Then, we assigned the value “Carlton Lewis Hernandez” to the name
variable. When you assign a new value to a variable, the program overwrites the most recent value, replacing it with the new one.
Python Global Variables
In Python, there are two main types of variables: local and global.
Global variables are variables declared outside of a function. Global variables have a global scope. This means that they can be accessed throughout an entire program, including within functions. Consider the following visual representation of this concept.
Global and Local Variables in Python
A global variable can be accessed throughout a program.
Here is an example of a global variable in Python:
name = "Carlton Hernandez"
Once we declare a global variable, we can use it throughout our code. For example, we can create a function that prints the value held by our global variable name
using the following code:
def printName(): print(name) printName()
Our code returns:
"Carlton Hernandez"
Here, we initialized a function called printName()
. This function, when invoked, prints the value of the name
variable to the console. This is what happened above when we invoked the function.
Python Local Variables
Local variables, on the other hand, are variables declared inside a function. These variables are known to have local scope
. This means they can be accessed only within the function in which they are declared. Consider again this visual representation we displayed earlier that depicts the concept of variables in Python:
Global and Local Variables
A local variable can only be accessed within a particular function.
The following is an example of a program that uses a local variable:
def printName(): name = "Georgia Anderson" print(name) printName()
Our code returns:
"Georgia Anderson"
In our code, we declared a function called printName()
. Within that function, we defined a variable called name
. Because we declared this variable within a function, it is a local variable.
At the end of our code, we called our function using printName()
. In response, the program executed the printName()
function.
The name
variable in this example is local to the printName()
function. Therefore, we cannot access that variable outside of our function. Here’s what happens when we try to print this local name
variable outside of the function in which it is declared:
def printName(): name = "Georgia Anderson" print(name) printName() print(name)
Our code returns:
NameError: name 'name' is not defined
We cannot access a local variable outside of the function in which we assigned that variable. When we try to access a local variable in our main program, the program will return an error.
Using Global and Local Variables
It is possible for a program to use the same variable name for both a local and a global variable. In such a scenario, the local variable will be read in local scope, and the global variable will be read in global scope.
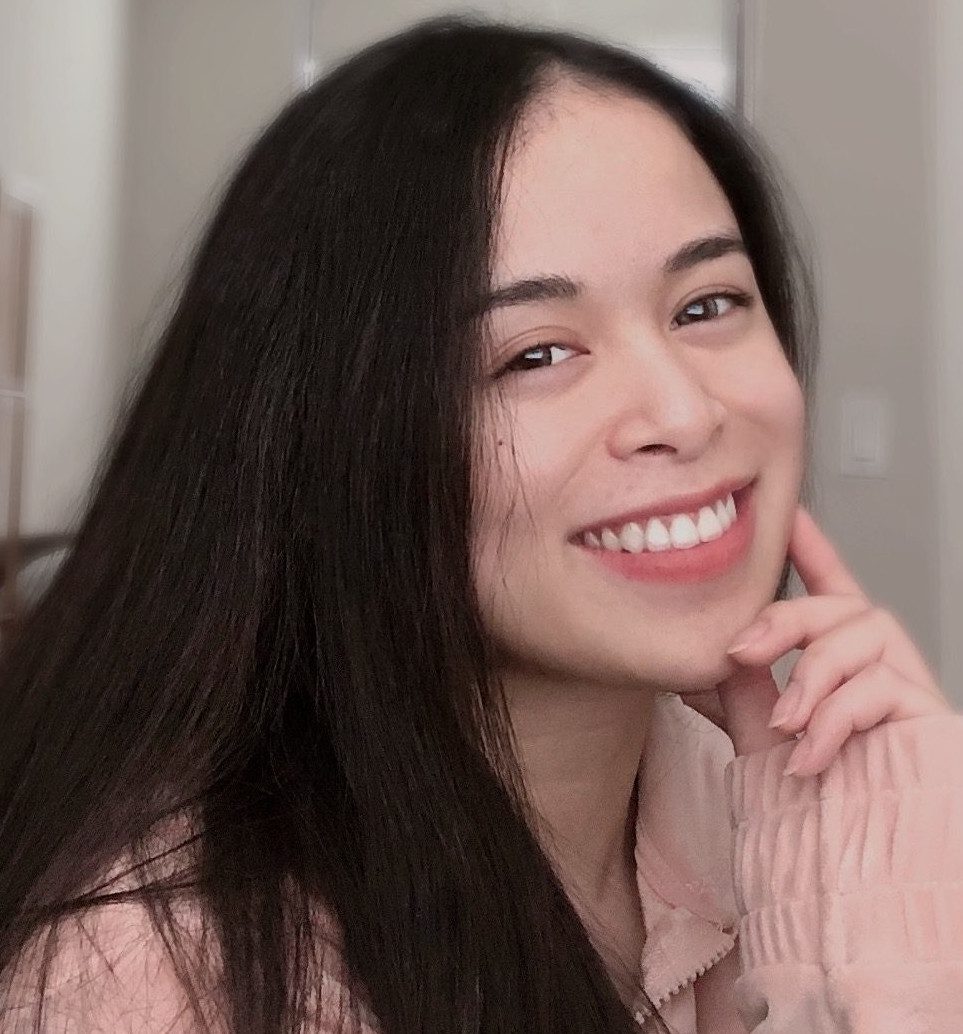
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here’s an example of a program that has local and global variables with the same name:
score = 5 def calculateScore(): score = 10 print("Final Score:", score) calculateScore() print("Initial Score:", score)
Our code returns:
Final Score: 10 Initial Score: 5
First, we assigned the global variable score
in our code. Then, in our calculateScore()
function, we created a local variable with the same name.
The value of our local score
variable in the calculateScore()
function is 10. So, when we call our calculateScore()
function, the message Final Score: 10
is printed to the console.
However, outside of our calculateScore()
function, the value of the score
variable is 5. This is because we set the value of the score
variable in global scope to 5. So, when we print out Initial Score:
, followed by the value of the score
variable, the program displays the value 5.
Assigning Global Variables within a Function
In the above sections, we learned that global variables are defined outside of a function (i.e., globally), and local variables are defined inside a function (i.e., locally). However, if you use the global
keyword to define a variable within a function (i.e., locally), and then run that function in the program (globally), that variable will become a global variable.
Consider the following example:
def setName(): global name name = "Bianca Peterson" setName() print(name)
Our code returns:
"Bianca Peterson"
In our code, we assigned the value of the name
variable locally, inside the setName()
function. However, because we used the global keyword before defining this variable, we prepare to give the name
variable global scope. However, the name
variable will become a global variable only after we run this function in the program.
After the program runs the setName()
function, any time we use the name
variable in our program, as we did when we ran the print()
function, the program will use the value we declared in the local function. This is because the name
variable is now a global variable.
Remember how earlier we said that can’t directly change a global variable inside a function? That is true. However, you can use the global keyword to change a global variable inside a function indirectly.
Here’s an example of a program that uses the global keyword in a function to change the value of a global variable:
name = "Bianca Peterson" def setName(): global name name = "Bianca Lucinda Peterson" setName() print(name)
Our code returns:
"Bianca Lucinda Peterson"
Let’s break down this code. First, we declared a variable called name
and assigned it the value “Bianca Peterson”. This is a global variable. Then, we declared a function called setName()
. When called, the setName()
function changes the value of the name
variable.
We then called the setName()
function, which changed the value of name
to “Bianca Lucinda Peterson”. Finally, we printed the value of name
to the console. The value of the global variable name
is now “Bianca Lucinda Peterson”.
Generally speaking, the global keyword should be used sparingly. Using the global keyword too often can make it difficult to understand the scope of variables in a program.
Conclusion
Global variables are variables declared outside a function. Local variables are variables declared inside a function.
While global variables cannot be directly changed in a function, you can use the global keyword to create a function that will change the value of a global variable. In this case, the global variable’s value will not actually change until you run that function.
This tutorial discussed, with reference to examples, the basics of local and global variables, and how they work. Now you’re ready to start using local and global variables in your Python code like a professional programmer!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.