A Python function is a group of code. To run the code in a function, you must call the function. A function can be called from anywhere after the function is defined. Functions can return a value using a return statement.
Functions are a common feature among all programming languages. They allow developers to write blocks of code that perform specific tasks. A function can be executed as many times as a developer wants throughout their code.
Functions allow developers to reduce repetition in their code because they can execute the same block of code multiple times over in one program.
This tutorial will discuss, with examples, the basics of Python functions, how to create and call a function, and how to work with arguments. By the end of reading this tutorial, you’ll be an expert at writing functions in Python.
What is a Python Function?
A function is a block of code that only runs when it is called. Python functions return a value using a return statement, if one is specified. A function can be called anywhere after the function has been declared.
By itself, a function does nothing. But, when you need to use a function, you can call it, and the code within the function will be executed.
In Python, there are two types of functions: user-defined and built-in. Built-in functions are functions like:
- print(), which prints a statement to the console
- Python len(), which calculates the length of a list
- Python str(), which converts a value to a string
User-defined functions are reusable blocks of code written by you, the developer. These blocks of code allow you to organize your code more efficiently. This is important because the more organized your code is, the easier it will be to maintain.
How to Define a Python Function
Defining a function refers to creating the function. This involves writing a block of code that we can call by referencing the name of our function. A function is denoted by the def keyword, followed by a function name, and a set of parenthesis.
For this example, we’re going to create a simple function that prints out the statement It’s Monday! to the console. To do so, we can use this code:
def print_monday(): print("It's Monday!")
When we run our code, nothing happens. This is because, in order for our function to run, we need to call it. To do so, we can reference our function name like so:
def print_monday(): print("It's Monday!") print_monday()
Our code returns:
It's Monday!
Let’s break down the main components of our function:
- the def keyword is used to indicate that we want to create a function.
- print_monday is the name of our function. This must be unique.
- () is where our parameters will be stored. We’ll talk about this later.
- : marks the end of the header of our function.
Now, our functions can get as complex as we want them to be. Suppose we want to write a program that tells a user how many letters are in their name. We could do so using this code:
def calculate_name_length(): name = str(input("What is your name? ")) name_length = len(name) print("The length of your name is ", name_length, " letters.") calculateNameLength()
If we run our code and type in the name “Elizabeth”, the following response is returned:
The length of your name is 9 letters.
We define a function called calculate_name_length(). In the function body, we ask the user for their name, then use len() to calculate the length of the user’s name. Finally, we print “The length of your name is [length] letters.”, where length is the length of the user’s name, to the console.
Function Parameters and Arguments
In our first examples, we used empty parenthesis with our functions. This means that our functions did not accept any arguments.
Arguments allow you to pass information into a function that the function can read. The arguments for a function are enclosed within the parentheses that follow the function’s name.
Let’s walk through a basic example to illustrate how arguments work.
Python Parameters and Arguments Example
Suppose we want to create a program that multiplies two numbers. We could do so using this code:
def multiply_numbers(number1, number2): answer = number1 * number2 print(number1, " x ", number2, " = ", answer) multiply_numbers(5, 10) multiply_numbers(15, 2)
Our Python program returns:
5 x 10 = 50 15 x 2 = 30
First, we define a function called multiply_numbers. The parameter names in the function that our code accepts are: number1 and number2. We define these between brackets which is where the parameter list is defined.
Next, we declare a Python variable called “answer” which multiplies the values of number1 and number2. Then, we print a statement to the console with the full mathematical sum written out, followed by the answer to the math problem.
We have specified required arguments. This is because we have set no default value for each argument. We must specify a number of arguments equal to those in the parameter list otherwise the Python interpreter will return an error.
Toward the end of our program, we call our multiply_numbers function twice.
First, we specify the arguments 5 and 10. Our program multiplies these values together to calculate 50. Then, our program prints “5 x 10 = 50” to the console. Next, we specify the arguments 15 and 2, which our program multiplies. Then, our program prints “15 x 2 = 30” to the console.
By default, the order of the arguments you pass in a function is the order in which they are processed by your program. When we run “multiply_numbers(5, 10)”, the value of “number1” becomes 5. The value of “number2” becomes 10. We’ll talk about how to override this in the “keyword arguments” section.
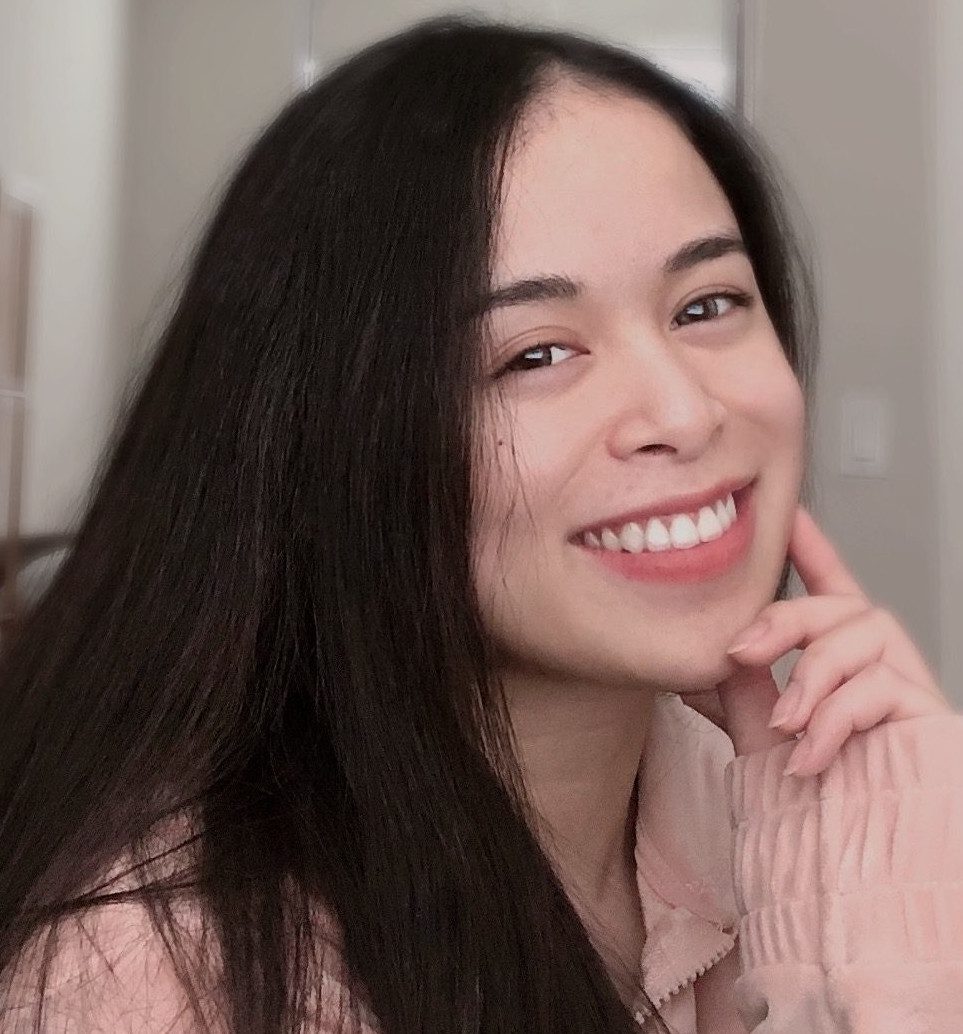
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
For more information on arguments, check out our Python optional arguments tutorial.
A Note: Parameters vs. Arguments
The terms parameter and argument refer to the same thing: passing information to a function. But, there is a subtle difference between the two.
A parameter is the variable inside the parenthesis in a function. An argument is the value that is passed to a function when it is called. So, in our last example, “number1” and “number2” are parameters, and 5 and 10 are arguments.
Function Keyword Arguments
As we discussed, the order in which you pass arguments is the order in which your program will process them. So, the first parameter will be assigned to the first argument, and so on. However, there is a way to override this rule.
You can use keyword arguments in a function call, which allows you to assign the value of an argument based on its parameter name. Using keyword arguments allows you to specify the value of keywords in any order you want.
Keyword arguments work because you’ll use keywords to match values to parameters, instead of relying on the order of the arguments to pass values.
Suppose we are creating a program that prints out the name and email address of someone who has signed up to a mailing list. We could write this program using the following code:
def print_info(name, email): print("Name: ", name) print("Email: ", email) print_info(email="alex.hammond@gmail.com", name="Alex Hammond")
Our code returns:
Name: Alex Hammond Email: alex.hammond@gmail.com
We declare a function that accepts two parameters: name and email. We print “Name:” to the console, followed by the value in the name parameter. Then, we print “_Email:”_to the console, followed by by the value in the email parameter. We use Python print() statements to print these values to the console.
Next, we call our function and specify two arguments. The email argument is made equal to alex.hammond@gmail.com, and the name argument is made equal to Alex Hammond.
In our code, we separated the name of the argument and its value using an equals sign (=). This meant that we no longer had to specify our arguments in the order our parameters appear (name, email). We could use any order we wanted.
Default Argument Values
In addition, you can specify a default argument value for a parameter in a function.
Suppose we want the value of email to be default@gmail.com by default. We could make this happen using the following code:
def print_info(name, email="default@gmail.com"): print("Name: ", name) print("Email: ", email) print_info("Alex Hammond")
Our Python code returns:
Name: Alex Hammond Email: default@gmail.com
We set the default value of the email parameter to be default@gmail.com. When we run our code and call the print_info() function, we don’t need to specify a value for the email argument. In this example, when we execute print_info(), we only specify one argument: the name of the user.
Returning Values to the Main Program
So far, we’ve discussed how we can pass values into a function. But a function can also be used to pass values to the rest of a program.
The return statement exits a function and allows you to pass a value back to the main program. If you use the return statement without an argument, the function will return the value None.
Suppose we want to create a program that multiplies two numbers. Then, when those two numbers have been multiplied, we want to return them to our main program. We could do so using this code:
def multiply_numbers(number1, number2): answer = number1 * number2 return answer ans = multiply_numbers(5, 6) print(ans)
Our code returns:
30
First, we define a function called multiply_numbers. This function accepts two parameters: number1 and number2. When this function is called, the values of “number1” and “number2” are multiplied. Then, we use the return statement to pass the multiplied number to the main program.
We call the multiply_numbers() function and specify two arguments: 5 and 6. Notice that we also assign the function’s result to the variable “ans”. When this line of code is run, our function is called, and its result is assigned to “ans”. Then, our code prints out the value of “ans”, which is 30 in this case.
The Python return statement stops a function from executing, even if it is not returning a value. Here’s an example of this behavior in action:
def run_ten(): for i in range(0, 10): if i == 4: return print("Finished") run_ten()
Our code prints nothing to the console. Although there is a “print(“Finished”)” statement in our code, it does not run.
This is because, when our for loop executes four times (when i is equal to 4), the return statement is executed. This causes our function to halt execution and stops our loop from running.
After our function stops running, the code in our main program will continue to run.
Conclusion
Python functions are blocks of code that execute a certain action. Functions can be called as many times as you want in a program. This means that you can run the same block of code multiple times without having to repeat your code.
Functions allow you to reduce repetition in your code, thereby making your programs easier for both you and other coders to read.
For a challenge, write a function that prints every number between 1 and 10 to the console (inclusive of 10). This function should contain a for loop. When the function is done, you should print “Done!” to the console. Call your function once at the end of your program.
The output should be:
1 2 3 4 5 6 7 8 9 10
This tutorial discussed the basics of functions in Python, how to write and call a function, and how to work with arguments and parameters. Now you’re ready to start writing functions in Python like an expert!
For advice on the top Python courses, books, and learning resources, check out our comprehensive How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.