A Python for loop iterates over an object until that object is complete. For instance, you can iterate over the contents of a list or a string. The for loop uses the syntax: for item in object, where “object” is the iterable over which you want to iterate.
Loops allow you to repeat similar operations in your code. One of the most common types of loops in Python is the for loop. This loop executes a block of code until the loop has iterated over an object.
This tutorial will discuss the basics of for loops in Python. We’ll talk about to use the range() function and iterable objects with for loops.
What is a Python for Loop?
A Python for loop runs a block of code until the loop has iterated over every item in an iterable. For loops help you reduce repetition in your code because they let you execute the same operation multiple times.
Here is the basic structure of a for loop in Python:
for [item] in [sequence]: # Run code
Let’s look at our for loop:
- for tells Python we want to declare a for loop.
- item tracks the individual item each iteration is viewing.
- in separates the item from the sequence.
- sequence refers to the object over which you want to iterate.
The code that is within our for loop will run until every item in our sequence has been read by our program.
Python for Loops Example
You can use a for loop to run through a list of items stored in an iterable object.
The term iterable object is another way of saying any object that stores a sequence of items. Here are a few types of iterable object:
Suppose we have a list of cat breeds that we want to print out to the console individually. We could do so using this code:
breeds = ['Persian', 'Maine Coon', 'British Shorthair', 'Ragdoll', 'Siamese'] for b in breeds: print(b)
Our code returns:
Persian Maine Coon British Shorthair Ragdoll Siamese
We have specified a list as the sequence our for loop should run through. Our for loop goes through every item in our list, then prints out that item to the console.
We used the Python variable b to refer to each item in our list. However, we could use any name for our variable, such as breed or x. The variable name must be valid. It should not have the same name as any other variable being used in our loop.
You can also iterate through strings and other sequential data types like dictionaries. Suppose we wanted to print out each character in a string individually. You could do so using this code:
persian = 'Persian' for l in persian: print(l)
Our code returns:
P e r s i a n
Our code loops through every letter in the Persian string. This is because our loop body contains a print statement that prints out each character in the string.
You can add a break statement and a continue statement inside a loop. A break statement stops a loop from executing whereas a continue statement skips to the next iteration of a loop. To learn more about break and continue statements, read our guide to Python break and continue statements.
for Loops Python: Using range()
The range() function creates a sequence of numbers in a given range. You can use range() to specify how many times a loop should iterate. When used with len(), range() lets us create a list with a length equal to the number of values in an object.
Let’s use a simple example of a for loop to illustrate how this operation works. Suppose we want to print out a list of every number between 1 and 5. We could do so using this code:
for item in range(5): print(item)
Our code returns:
0 1 2 3 4
In our code, we use item to keep track of the item the for loop is reading. Then, we use range(1, 6) to create a list of all numbers in the range of 1 and 6 (because range() starts counting from 0, we need to specify 6 as our high value if we want to see all numbers between 1 and 5).
This function accepts three arguments, which are as follows:
- start: The starting value at which the sequence should begin. By default, this is 0. (optional)
- stop: The value at which the sequence should end. (required)
- gap: The gap between each value in the sequence. By default, this is 1. (optional)
You can learn more about the range() built-in function in our complete guide to Python range().
Now, suppose we want to run our loop 5 times. We could do so using this code:
Our iterates over a sequence of numbers created by range() and returns:
In our code, we use the range() function to specify that we want to run our for loop 5 times. Then, we print out the value of each item to the console. The range() function starts counting at 0 by default.
Nested for Loops: Python
Python nested loops are loops that are executed within another loop. They are often used to iterate over the contents of lists inside lists. A nested for loop is another way of saying “a loop inside another loop.”
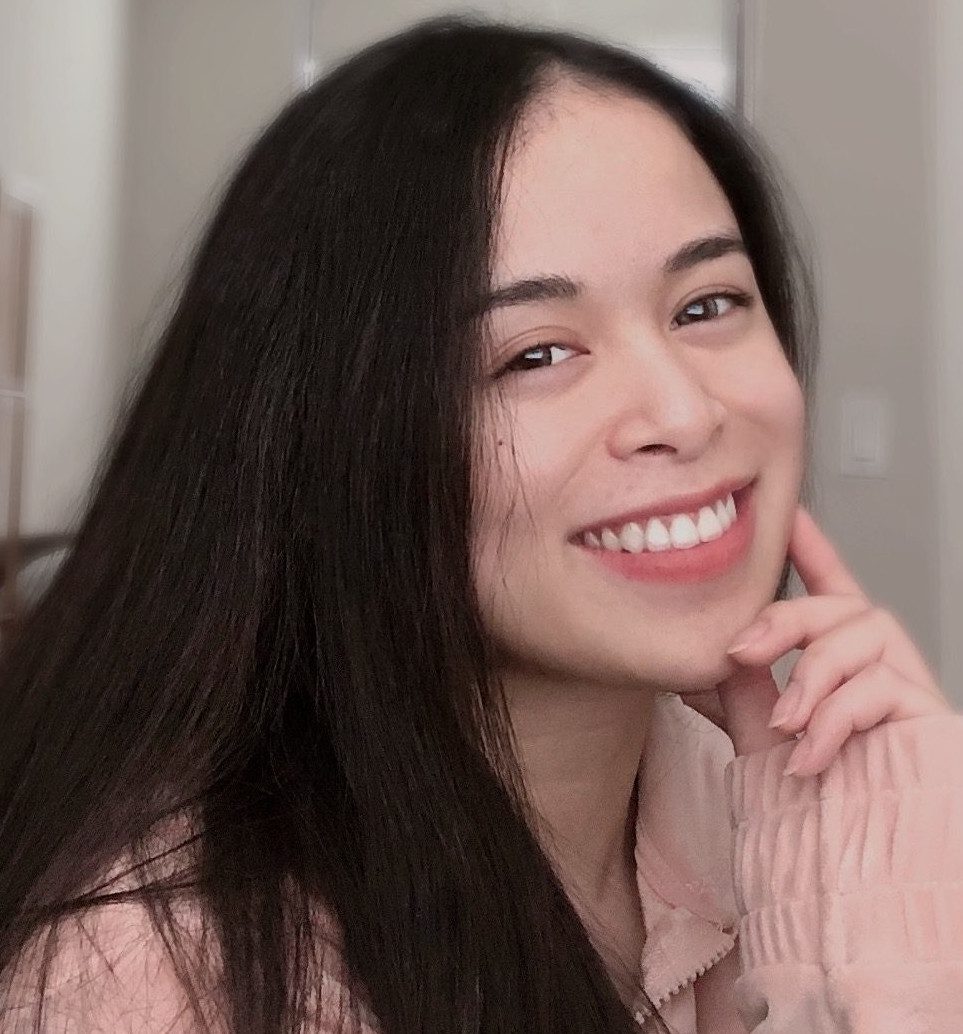
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Here is the structure of a nested for loop in Python:
for [outer_item] in [outer_sequence]: for [inner_item] in [inner_sequence]: // Run code
In a nested for loop, the program will run one iteration of the outer loop first. Then, the program will run every iteration of the inner loop, until all the code in the outer loop has been executed.
Once this point is reached, the outer loop will be executed again, and this process will continue until the program has been run.
Suppose we have a list of lists whose values we want to print to the console.
The first list contains a list of our most popular cat breeds. Our second list contains the cat breeds we are no longer breeding. The third list contains a list of cat breeds we are thinking of breeding. We could print these lists using this code:
breeds = [ ['Persian', 'British Shorthair', 'Siamese'], ['Cornish Rex', 'Malayan', 'Maine Coon'], ['Himalayan', 'Birman'] ] for outer_list in breeds: for breed in outer_list: print(breed)
Our code returns:
Persian British Shorthair Siamese Cornish Rex Malayan Maine Coon Himalayan Birman
We have defined a list of lists called breeds. We use a nested for loop to iterate through every item in the outer list and every item in each inner list. We print out each value from our lists to the console.
View the Repl.it from this tutorial:
Conclusion
Python for loops execute a block of code until the loop has iterated over every object in an iterable. for loops help reduce repetition in your code. You can iterate over lists, sets, dictionaries, strings, and any other iterable.
When used with the range() statement, you can specify an exact number of times a for loop should run. If you use a for loop with an iterable object, the loop will iterate once for each item in the iterable.
We have a challenge for you:
Write a for loop that prints out all of the values in the following list to the console:
[1, 9, 2, 3, 4]
Once your loop prints these values, you should add a line of code into your loop that multiplies each number by two.
Your code should return:
1 2 9 18 2 4 3 6 4 8
Now you have the knowledge you need to start using for loops using the Python programming language. For advice on top online Python learning resources, courses, and books, check out our comprehensive How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.
I found this guide so informative. I have been battling to understand but now I managed to get and idea.