The Python ceil() function rounds a number up to the nearest integer, or whole number. Python floor() rounds decimals down to the nearest whole number. Both of these functions are part of the math Python library.
When programming in Python, you may encounter a scenario where you want to round a number to the nearest integer.
That’s where the Python math.floor() and math.ceil() methods come in. You can use the math.floor() method to calculate the nearest integer to a decimal number. The math.ceil() method rounds a number down to its nearest integer.
This tutorial will discuss using the floor and ceil methods to return the floor or ceiling of a provided value. We’ll walk through examples of each of these methods in a program to showcase how they work.
Python Floor Function
The Python math.floor() method rounds a number down to the nearest integer. This method takes one argument: the number you want to return. In Python 3, math.floor() returns an integer value.
Calculating the floor of a number is a common mathematical function in Python. The floor of a number refers to the nearest Python integer value which is less than or equal to the number. To put it another way, the floor of a number is the number rounded down to its nearest integer value.
The Python math module includes a method that can be used to calculate the floor of a number: math.floor(). math.floor() takes in one parameter, which is the number whose floor value you want to calculate.
Here’s the syntax for the floor() function:
import math math.floor(number)
The Python floor() division function is part of the math library. In order to use it, we first need to import the math library. You can import the math library into your program using a Python import statement.
Floor() Python Example
Say that we are working in a coffee shop. We want to create a calculator that rounds down the quantities of the beans we have available to their nearest whole number. This makes it easier for us to understand the quantity of much coffee we have left.
We could round down the quantity of a bean to its nearest whole number using the following code:
import math quantity = 250.92 rounded = math.floor(quantity) print(rounded)
Our code returns the smallest integer closest to 250.92, which is: 250.
On the first line, we import the math library. Next, we define a Python variable called quantity. This variable stores the quantity of the bean we have in storage. We use the math.floor() function to round down the quantity variable to its nearest whole number.
In this case, the nearest whole number to 250.92 is 250. Our code returned 250.
We can use the math.floor() method on negative numbers. Let’s say that we are writing a program that calculates the quantity of many beans we’ll have left at the end of the month.
Our program has projected that, given how many sales we have seen so far, we will have a negative amount of beans. In other words, we will run out of beans. We print the math.floor() output to the console.
We want to round down our value to the nearest whole number. This will let us know the quantity of beans to order, based on current demand:
import math quantity_projection = -25.21 rounded = math.floor(quantity_projection) print(rounded)
Our code returns: -26.
The program has rounded down our negative value to the nearest whole integer, which in this case is -26.
Python Ceil
The math.ceil() method is the opposite of the math.floor() method. math.ceil() rounds a number up to the nearest integer. Like math.floor(), math.ceil() returns an integer value.
Whereas floor rounds down a number to its nearest whole value, ceil rounds a number up to its nearest whole value.
Here’s the syntax for the math.ceil() method:
import math math.ceil(number)
The syntax for the ceil function is the same as the syntax for math.floor(). Both methods take in one parameter: the number you want to process using the method. The ceil() function returns the ceiling number of a float, or the largest integer closest to it.
ceil() Python Example
Let’s discuss an example of the math.ceil() method in action. Say that we have decided we want to calculate the ceiling value of each bean quantity. In other words, we want to know the smallest whole number above each bean quantity. We want to calculate this so we know how many beans to order in our next shipment.
We could use the following code to round up the bean quantity we have to the nearest whole number:
import math quantity = 22.15 rounded = math.ceil(quantity) print(rounded)
Our code returns: 23. The math.ceil() function has rounded up our quantity to the nearest whole integer not less than the quantity, which in this case is 23.
Similarly, we can use math.ceil() on negative numbers. Let’s use the example of the program we discussed earlier to show how this works. Instead of finding the floor value of our remaining quantity, we want to find the ceiling value. We could do so using this program:
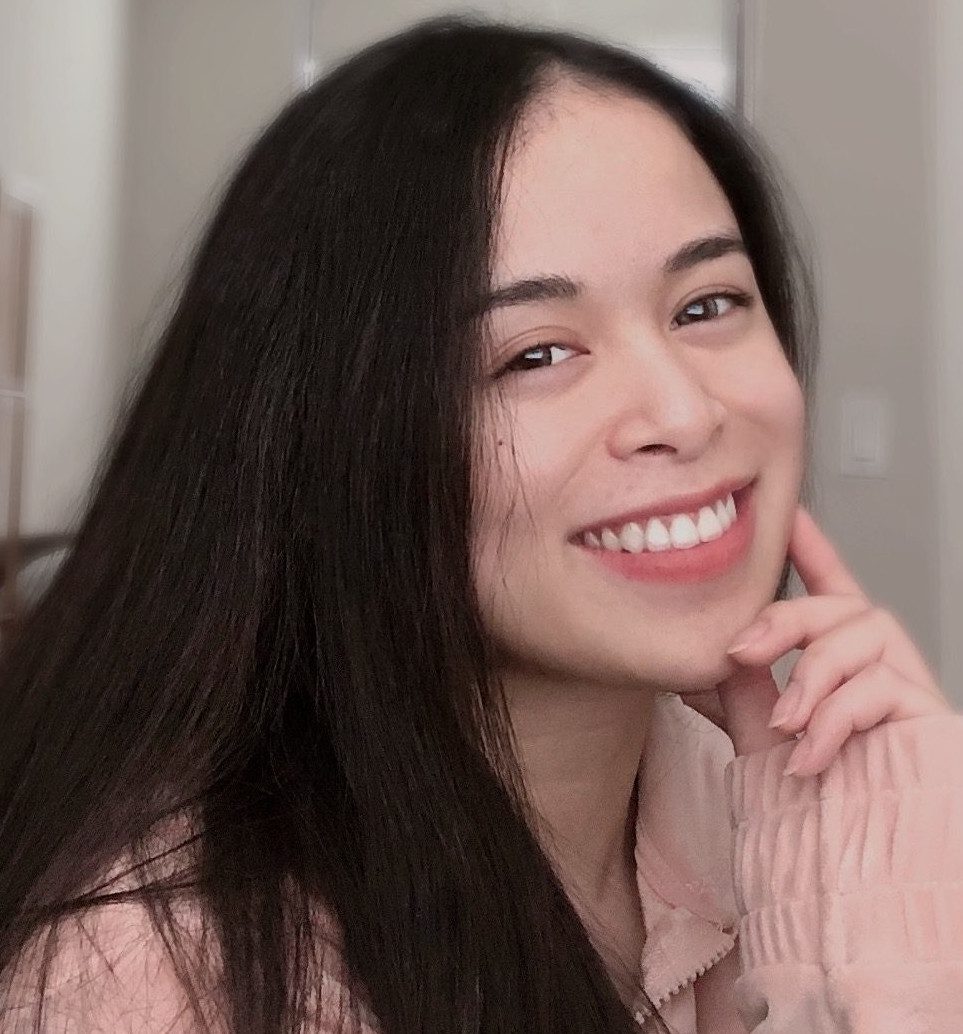
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
import math quantity_projection = -25.21 rounded = math.floor(quantity_projection) print(rounded)
Our code returns: -26. Our program has rounded our quantity projection up to the nearest whole number, which in this case was -26.
Python Floor Division and Ceil vs. Round
The Python round() method searches for the nearest number, which could include decimals, while math.floor() and ceil() round up and down to the nearest integer(), respectively.
If you wanted to round a number like 105.2529 to two decimal places, you’d want to use round() instead of floor() or ceil().
If you’re interested in learning more using the round() method, check out our tutorial on Python round.
Conclusion
The math.floor() method allows you round a number down to its nearest whole integer. math.ceil() method allows you to round a number up to its nearest whole integer.
This tutorial discussed using both the math.floor() and math.ceil() functions to round numbers in Python. We walked through an example of each of these methods in a program.
To learn more about coding in Python, read our complete How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.