Flattening a list refers to the process of removing a dimension from a list. A dimension refers to an additional co-ordinate needed to locate an item in a list. You can flatten a Python list using a list comprehension, a nested for loop, or the itertools.chain() method.
A Python list can contain multiple dimensions. This means that you have a list within lists. These lists, sometimes referred to as ‘nested lists,” can be converted back into a regular list.
This means you can move all the values from lists within a list to a single list. Turning a list of lists into a list is called “flattening a list.”
Python Flatten List
There are three ways to flatten a Python list:
- Using a list comprehension.
- Using a nested for loop.
- Using the itertools.chain() method.
In this guide, we talk about how to flatten a list using a list comprehension, a for loop, and the itertools.chain() method. We walk through two examples so you can start flattening lists in your own programs.
Python: Flatten List Using a List Comprehension
A Python list comprehension defines a list based on the contents of an existing list. You can customize each element that goes into your new list by specifying conditions or changes within the comprehension.
Comprehensions are syntactic sugar for using a for loop to iterate over a list and generate a new list. This means they work in the same fundamental way as a for loop but use different syntax.
Here is the syntax for a list comprehension:
numbers = [1, 2, 3] new_numbers = [number * 2 for number in numbers]
This comprehension multiplies each number in our “numbers” list by 2.
Flatten List: List Comprehension Example
We have a list of lists that contains different sandwich fillings available at a restaurant. Currently, our list of lists looks like this:
foods = [ ["Tomato and Cucumber", "Hummus, Beetroot, and Lettuce"], ["Cheese", "Egg"], ["Ham", "Bacon", "Chicken Club", "Tuna"] ]
The first list contains vegan sandwich fillings; the second list contains vegetarian sandwich fillings; the third list contains all sandwich fillings that contain meat.
We want to change this list into a single list of foods. It is easier to work with a list with one dimension rather than two. There is no need to have more than one dimension in this list. To remove the second dimension from our list, we can use a list comprehension:
new_foods = [food for sublist in foods for food in sublist] print(new_foods)
This list comprehension iterates over every list in the “foods” Python variable. Each value is then added to a main list. Once our list is generated, we print it out to the console.
Let’s our code and see what happens:
['Tomato and Cucumber', 'Hummus, Beetroot, and Lettuce', 'Cheese', 'Egg', 'Ham', 'Bacon', 'Chicken Club', 'Tuna']
Our list of lists has been successfully transformed into a flat list. All ingredients now appear inside one list instead of three.
Python: Flatten List Using a Nested For Loop
We also achieve this same result by using a nested Python for loop. “Nested for loop” is another way of saying “a for loop within a for loop.” Our list comprehension is another way of representing the following for loop:
new_foods = [] for sublist in foods: for food in sublist: new_foods.append(food) print(new_foods)
Our code uses two for loops to iterate over each list item in each list in the original list of lists. Let’s run our code:
['Tomato and Cucumber', 'Hummus, Beetroot, and Lettuce', 'Cheese', 'Egg', 'Ham', 'Bacon', 'Chicken Club', 'Tuna']
Our program returns the same result as our list comprehension. In most cases, a list comprehension is a better solution than a for loop. List comprehensions are shorter and, in this case, are easier to read than a nested for loop.
Python: Flatten List Using Itertools
Itertools is a module in the Python standard library. The module provides a range of methods, making it easy to work with iterable objects and generators.
For our purposes, we only refer to the chain() method. This method accepts a list of lists and returns a flattened list.
Start by importing the itertools module into our code using a Python import statement:
import itertools
Next, define the list of lists and use the chain() method to flatten the list:
foods = [ ["Tomato and Cucumber", "Hummus, Beetroot, and Lettuce"], ["Cheese", "Egg"], ["Ham", "Bacon", "Chicken Club", "Tuna"] ] new_foods = itertools.chain(*foods)
In our code, we use the * symbol to unpack our list. This converts our list into function arguments that can be parsed by the chain() method.
The chain() method returns an itertools.chain object. To see our flattened list, we have to convert this object to a list. We do this using the list() method:
print(list(new_foods))
Let’s execute our code:
['Tomato and Cucumber', 'Hummus, Beetroot, and Lettuce', 'Cheese', 'Egg', 'Ham', 'Bacon', 'Chicken Club', 'Tuna']
Our code has flattened our list.
While itertools is an effective way at flattening a list, it is more advanced than the last two approaches we have discussed.
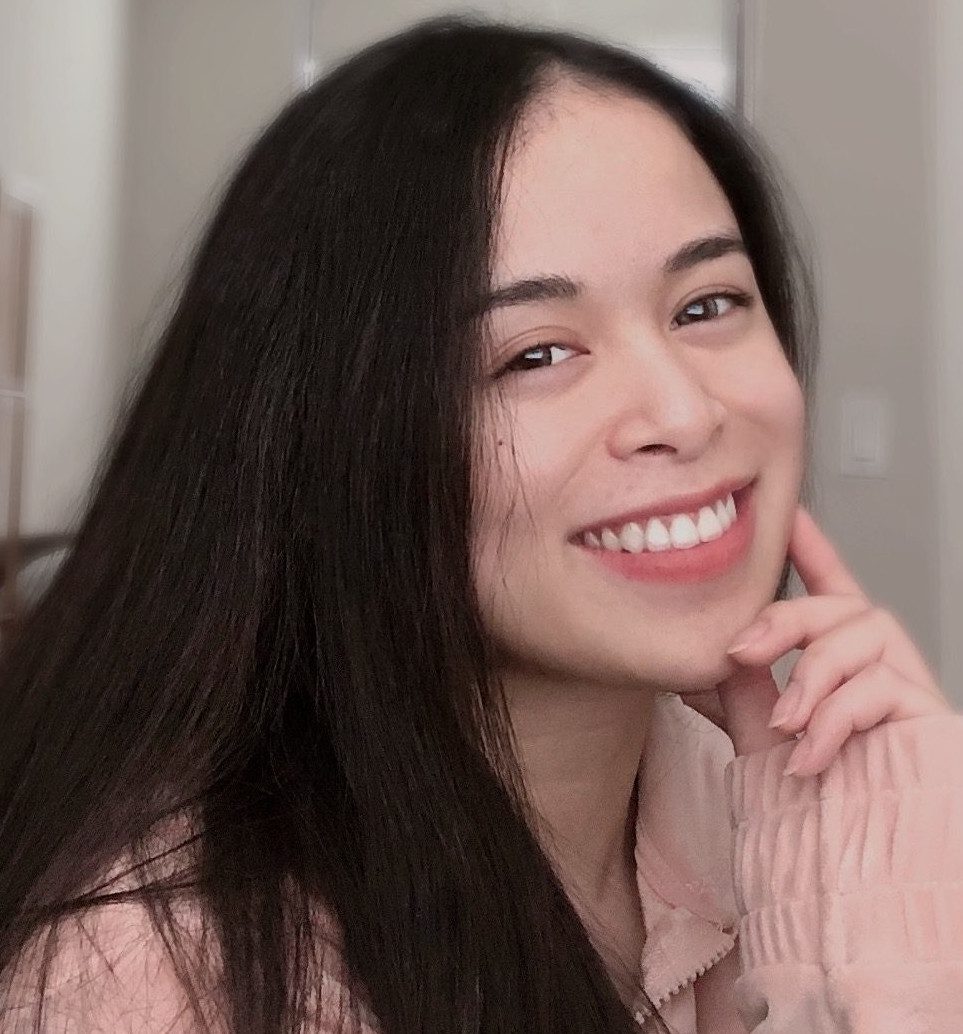
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
This is because you must import itertools into your code which introduces a new dependency. What’s more, the chain() method involves unpacking which can be difficult to understand.
Conclusion
You can flatten a Python list using a list comprehension, a nested for loop, and the itertools.chain() method. The list comprehension is the most “Pythonic” method and is therefore favoured in most cases.
While nested for loops are effective, they consume more lines of code than a list comprehension. The itertools.chain() method is similarly effective but it can be difficult for beginners to understand.
There’s usually no need to import a new library — itertools — when Python gives you all the tools you need to flatten a list.
Do you want to learn more about Python? Check out our complete How to Learn Python guide. You’ll find expert learning tips and a list of top courses and books from which you can learn.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.