How do you find an item in a Python list? It’s a question all Python coders encounter at some point. Luckily, the language offers a number of ways in which you can find an item in a list, such as using the in
operator or a linear search.
In this guide, we talk about four methods of finding an item in a list. We walk through an example of each of these methods so you can learn how they work.
Python Find in List Using “in”
Python has a special operator called in
. This operator checks to see whether a value is in a list. This operator is sometimes referred to as a “membership operator” because it checks whether a value is a member of a list.
In this example, we run a shoe store and we want to check whether a particular shoe is in stock. One way to do this is to use the in
operator.
Start by defining a list of shoes:
shoes = ["Adidas ZX Flux", "Adidas Ultraboost", "Adidas Gazelle", "Adidas Runfalcon"]
Next, we ask a user to insert a shoe to find:
to_find = input("What shoe are you looking for? ")
We use an input() statement to collect the name of the shoe. Next, we use an “if” statement to check whether the shoe a user has entered is in our list of shoes:
if to_find in shoes: print("{} shoes are in stock.".format(to_find)) else: print("{} shoes are not in stock.".format(to_find))
Our if
statement will execute if the shoe a user enters can be found in our list. Otherwise, the else
statement will run. Give our code a try:
What shoe are you looking for? Adidas Runfalcon Adidas Runfalcon shoes are in stock.
Our code successfully identifies that “Adidas Runfalcon” shoes are in stock. This causes our if
statement to execute.
Python Find in List Using a Linear Search
A linear search is a simple searching algorithm that finds an item in a list. A linear search starts at one end of a list and compares a value with every element in the list. If that value is in the list, a linear search returns the position of the item.
Start by writing a function that performs our linear search:
def linear_search(array, to_find): for i in range(0, len(array)): if array[i] == to_find: return i return -1
Our code uses a “for” loop to iterate through every item in the “array” list. If that item is found, the index position of that item is returned to the main program. If the item is not found after the entire list has been searched, -1 is returned to the main program.
Let’s go to our main program and call our function:
shoes = ["Adidas ZX Flux", "Adidas Ultraboost", "Adidas Gazelle", "Adidas Runfalcon"] to_find = input("What shoe are you looking for? ") found = linear_search(shoes, to_find)
This code asks the user for the shoe they are looking for. Then, our code calls the linear_search function to find whether that shoe is present in the list.
Finally, we use an if
statement to inform a user whether the shoe has been found:
if found != -1: print("{} shoes are in stock.".format(to_find)) else: print("{} shoes are not in stock.".format(to_find))
If our linear_search function returns -1, that means that an item has not been found in a list. Our if
statement will run if the value returned by the linear_search function is not equal to -1. If the value is equal to -1, the else
statement will run.
Try to run our code:
What shoe are you looking for? Adidas Samba Adidas Samba shoes are not in stock.
Our code cannot find Adidas Samba shoes in our list.
Python Find in List Using index()
The index() built-in function lets you find the index position of an item in a list. Write a program that finds the location of a shoe in a list using index()
.
To start, define a list of shoes. We ask a user to insert a shoe for which our program will search in our list of shoes:
shoes = ["Adidas ZX Flux", "Adidas Ultraboost", "Adidas Gazelle", "Adidas Runfalcon"] to_find = input("What shoe are you looking for? ")
Next, we use the index()
method to return the list index position of that shoe:
try: found = shoes.index(to_find) print("{} shoes are in stock. They are at index position {} in the list of shoes.".format(to_find, found)) except: print("{} shoes are not in stock.".format(to_find))
The index()
method returns a ValueError if an item is not found in a list. That’s why we use it as part of a “try…except” block.
If a shoe cannot be found, a ValueError is raised. At this point, the “except” block will be run. If a shoe is found, the “try” block will execute successfully.
Run our code:
What shoe are you looking for? Adidas Gazelle Adidas Gazelle shoes are in stock. They are at index position 2 in the list of shoes.
Our code successfully identifies that Adidas Gazelle shoes are in stock. Our program also tells us the position at which those shoes are stored in our list.
Python Find in List Using a List Comprehension
You find multiple items in a list that meet a certain condition using a list comprehension.
We have a list of shoes and we only want to return those that are branded Adidas. We can do this using a list comprehension. Start by defining a list of shoes:
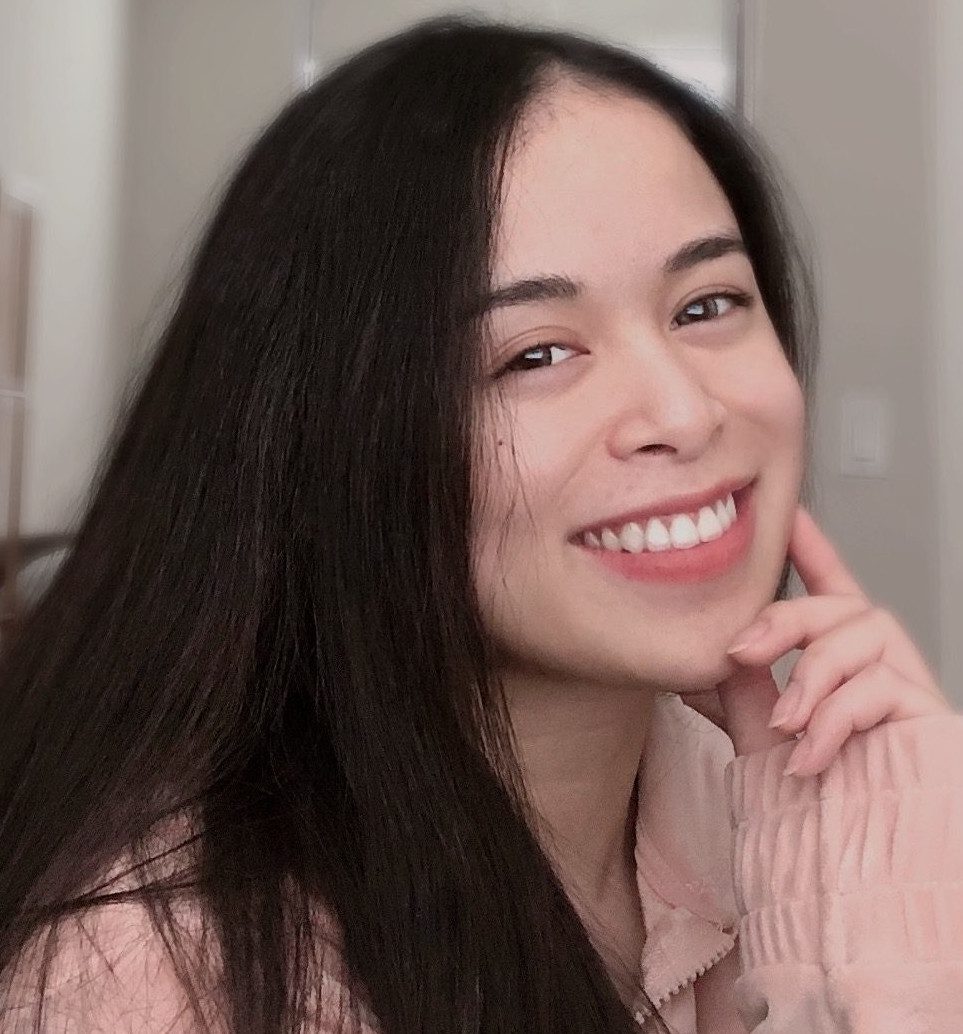
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
shoes = ["Nike PG 4", "Adidas Ultraboost", "Adidas Gazelle", "Nike Air Max Verona"]
Next, we write a list comprehension that retrieves all the shoes that contain the word “Nike”. List comprehensions use a syntax similar to a for loop:
nike_shoes = [shoe for shoe in shoes if "Nike" in shoe] print(nike_shoes)
The list expression iterates through every shoe in the “shoe” list. The expression checks if the word Nike is in each shoe name. If it is, that shoe is added to the “nike_shoes” list. Otherwise, nothing happens.
Let’s run our code:
['Nike PG 4', 'Nike Air Max Verona']
Our code returns a list of Nike shoes.
Conclusion
There are many ways in which you can find an item in a list. The most popular methods include:
- Using the
in
membership operator - Using a linear search
- Using the
index()
method - Using a list comprehension
Now you’re ready to search for items in a Python list like an expert coder!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.