The Python filter()
method is used to filter lists, sets, and tuples. filter()
follows any provided criteria to filter an iterable object, and returns the filtered result.
When you’re programming, you may have a list of values that you want to filter in some way. For example, you may have a list of cookie orders and only want to return those that involve chocolate, which are handled by a specific cook at your cookie store.
That’s where the Python filter()
method comes in. The filter()
method can be used to filter a particular list based on a predefined set of criteria and return an iterable with the filtered data.
In this tutorial, we will discuss the filter()
method and how you can use it in your code. We will also go through a couple of examples of the function in Python code.
Python Filter
Lists are a data type in Python that can be used to store multiple values with a common theme. For example, you could use a list to store all the clothes a local fashion store has on sale, or to store a list of programming language names.
Often, when you are working with lists, you’ll want to filter out the list based on a set of criteria. For example, you may be operating a movie theater and want to know how many people under the age of 18 attend movies at your cinema complex.
filter()
, a built-in function, can be used to filter out a list and returns an iterator. Here’s the syntax for the filter()
method:
filter(function, iterable_object)
The filter()
method takes two parameters:
- function is the code to be run on each item in the iterable you specify (required). The function will check if the iterable returns True or False.
- iterable_object is the object you want to filter (required).
The iterable object you specify can be any iterable such as Python lists
, sets
, and tuples
.
Python Filter Examples
Let’s run through an example to demonstrate how this method can be used.
Say that you are operating a magazine stand and you want to check whether or not you need to order new inventory. If you have less than 20 editions of any magazine, you need to place a new order; if you have more than 20 editions of a magazine, you don’t need to place a new order. You have a list of numbers that stores the quantities of magazines you have.
To check whether you need to place an order for any magazines, you could use the following code:
quantities = [25, 49, 21, 17, 14, 28] def checkQuantities(mags): if mags < 20: return True else: return False filtered_mags = filter(checkQuantities, quantities) print(list(filtered_mags))
Our code returns: [17, 14]
Let’s break down our code. On the first line, we define a variable called quantities
which stores how many editions of each magazine we have in our inventory.
Then, we define a function called checkQuantities
which will check whether we have fewer than 20 editions of a specific magazine in stock. If we have less than 20 editions, our checkQuantities
function returns True; otherwise, it will return False.
Next, we use the filter()
method and specify checkQuantities
as our function and quantities
as our iterable object. This tells our filter()
method to execute checkQuantities
on every item in the quantities array.
Then, we print out the result of our filter()
method, and use list()
to convert it to a list. This is important because filter()
returns a filtered object, not a list. So, if we want to see our data, we need to convert it to a list.
As you can see, our code returns two values: 17 and 14. Each of these is under 20, which means our program has worked as intended.
Let’s go through another example to show how this method works. Say we are a dance instructor and we want to know the ages of everyone in our class who is between seven and ten, inclusive of both seven and ten. We could use the following code to get this data:
student_ages = [7, 9, 8, 10, 11, 11, 8, 9, 12] def checkAges(ages): if ages >= 7 and ages <= 10: return True else: return False filtered_ages = filter(checkAges, student_ages) print(list(filtered_ages))
Our code returns: [7, 9, 8, 10, 8, 9]
. As you can see, our program has filtered out the students who are aged between seven and ten from our list elements, inclusive of both seven and ten. The resulting list contains our filtered data.
Python Filter with Lambda
To make our filter operation more efficient, we can use the list map Lambda function. Lambda is a special function that is defined without a name and can be used to write short, one-line anonymous functions in Python.
So, let’s take our magazine quantities example from above. In that example, we created a function that checked whether we had less than 20 editions of a magazine in stock and used filter()
to execute that function on our list of magazines. Then, our program returned a list of every magazine for which we had less than 20 editions.
We could use a Lambda function to perform this same action, but more efficiently. Here’s the code we could use:
quantities = [25, 49, 21, 17, 14, 28] filtered_mags = filter(lambda mag: mag < 20, quantities) print(list(filtered_mags))
Our code returns: [17, 14]
.
As you can see, our output is the same, but our code is significantly shorter. Instead of defining a whole function, we have used Lambda to define a one-line function.
This one-line function checks whether we have fewer than 20 copies of a magazine in stock, and returns True to the filter()
iterator if that is the case. After execution, our program returns a list with two values: 17 and 14.
Conclusion
The Python filter()
function can be used to filter an iterable object based on a set of predefined criteria and returns a filtered iterable. This method can be useful if you have a list of data from which you only want to retrieve values that meet certain criteria.
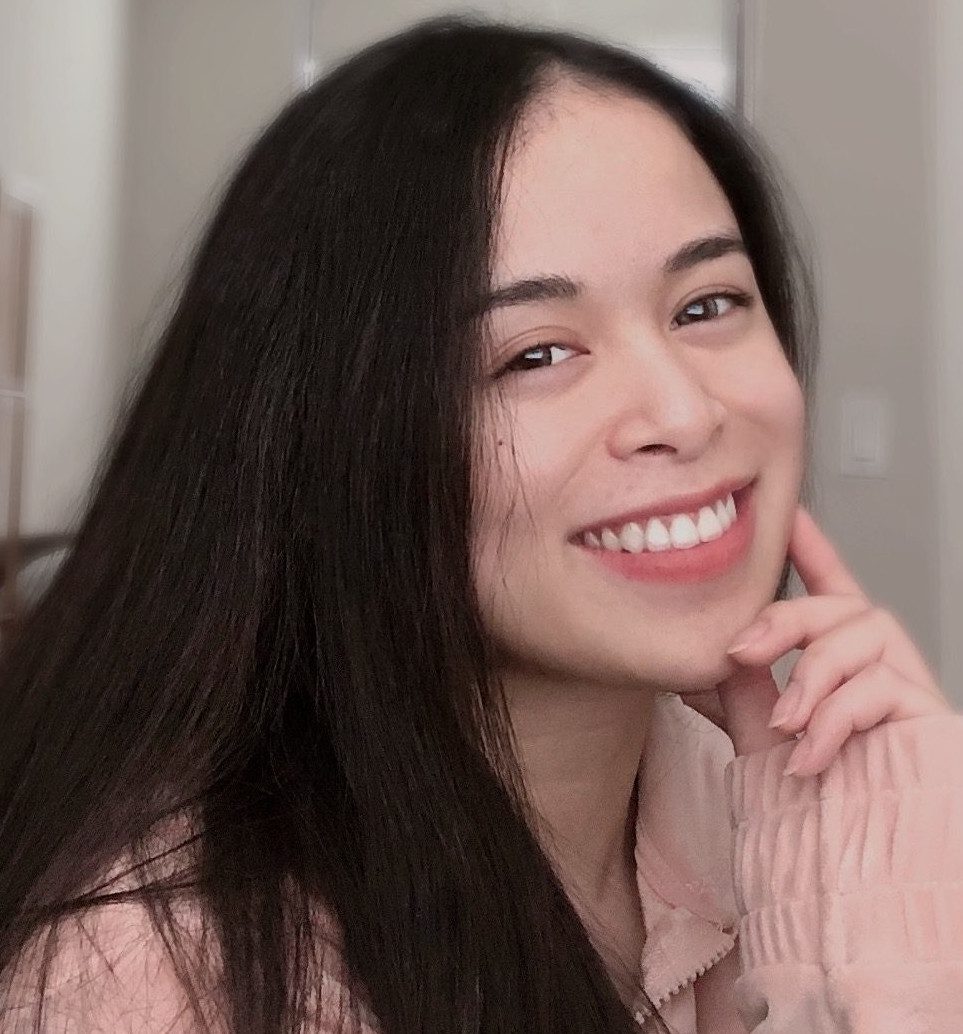
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
In this guide, we discussed the syntax for the filter()
method, and how it can be used in Python. We explored a few examples of filter()
in action, then we looked at how filter()
can be used with a Python Lambda function to make our code more efficient.
You’re now equipped with the information you need to use filter()
like a Python expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.