How to Calculate a Python Factorial
You may remember the word “factorial” from your high school math class. They’re not very easy to calculate without a calculator. Who wants to calculate the factorial of 10 by manually multiplying 1x2x3x4 and so on?
There are a few ways you can calculate a factorial in Python. In this guide, we’re going to talk about how to calculate a factorial using three approaches: the math.factorial method, a recursive function, and an iterative method.
Without further ado, let’s begin!
What is a Factorial?
A factorial is the product of all the whole numbers between one and another number.
Expressed as a mathematical formula, a factorial is:
n! = 1x2x3...n
The exclamation mark indicates that we are calculating a factorial. “n” is the number whose factorial we are calculating. Our calculation stops when we have multiplied all integers less than or equal to “n” are multiplied together.
Factorials cannot be calculated on negative numbers.
Python Factorial: math.factorial()
You can calculate a factorial using the Python math module. This library offers a range of methods that you can use to perform mathematical functions. For instance, you can use the math library to generate a random number.
The math.factorial()
method accepts a number and calculates its factorial. Before we can use this method, we need to import the math library into our code:
import math
Now, let’s write a Python program to find the factorial of 17:
number = 17 fact = math.factorial(number) print("The factorial of {} is {}.".format(number, str(fact)))
Our code returns: The factorial of 17 is 355687428096000.
The factorial()
method returns the factorial of a number.
We print that number to the console with the message: “The factorial of 17 is ”. We use a format()
statement so that we can add our number inside our string.
Python Factorial: Iterative Approach
Factorials can be calculated without the use of an external Python library. You can calculate a factorial using a simple for statement that calculates the product of all numbers in a range multiplied together.
Let’s start by declaring two variables:
number = 17 fact = 1
The first variable corresponds with the number whose factorial we want to calculate. The second variable will track the total of the factorial.
Next, we need to create a for loop which loops through every number in the range of one and our number:
for num in range(1, number+1): fact = fact * num print("The factorial of {} is {}.".format(number, str(fact)))
The for loop calculates the factorial of a number. The print statement shows us the total factorial that has been calculated in our for loop.
Our code returns: The factorial of 17 is 355687428096000.
This method is slightly less efficient than the math.factorial()
method. This is because the math.factorial()
method is implemented using the C-type implementation method. This offers a number of performance benefits.
If you want to calculate the factorial of a number without using an external library, the iterative approach is a useful method to use.
Python Factorial: Recursive Approach
A factorial can be calculated using a recursive function. A recursive function is one which calls upon itself to solve a particular problem.
Recursive functions are often used to calculate mathematical sequences or to solve mathematical problems. This is because there is usually a defined formula that is used to calculate the answer to a problem.
Open up a Python file and paste in the following function:
def calculate_factorial(number): if number == 1: return number else: return number * calculate_factorial(number - 1)
This function recursively calculates the factorial of a number. Next, we need to write a main program that uses this function:
number = 17 fact = calculate_factorial(number) print("The factorial of {} is {}.".format(number, str(fact)))
We have declared two variables: number and fact. Number is the number whose factorial we want to calculate. “fact” is assigned the result of the calculate_factorial()
function which calculates our factorial. Next, we print the answer to the console.
Our code returns: The factorial of 17 is 355687428096000.
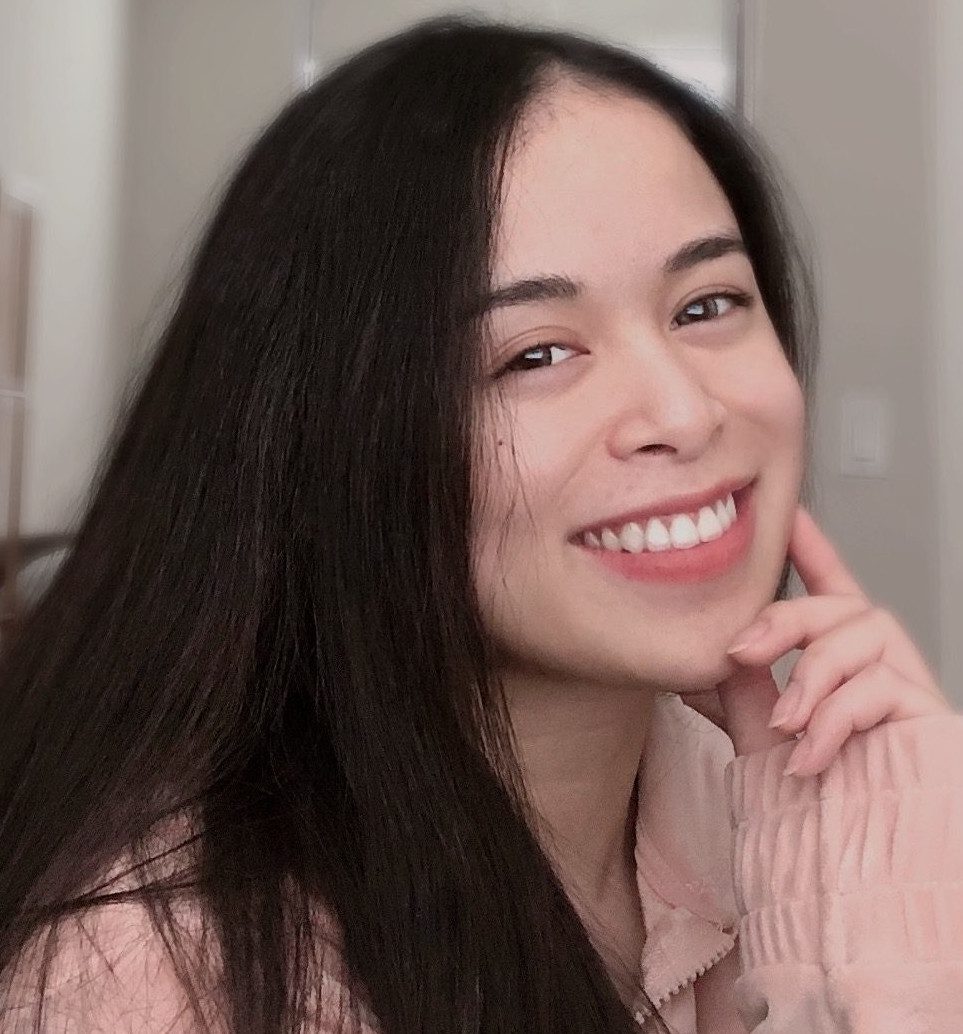
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Conclusion
Factorials are commonly used in mathematics. They are the product of all the whole numbers from one to another number when multiplied together.
You can calculate a factorial in Python using math.factorial()
, an iterative method, or a recursive function. The iterative and recursive approaches can be written in so-called “vanilla Python.” This means that you don’t need to import any libraries to calculate a factorial with these approaches.
Now you’re ready to calculate factorials in Python like an expert!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.