Python f strings embed expressions into a string. An expression may be the contents of a variable or the result of a mathematical calculation, or another Python value. f strings are distinguished from regular strings as the letter f comes before the string.
When you’re writing a string, you may want to change part of a string to use a specific value. For instance, you may want a string to appear that contains the value the user has inserted into the console.
Python has supported string formatting for a while, but Python 3.6 introduced a new method of changing strings to include new values: f strings.
This tutorial will discuss, with reference to examples, the basics of f strings in Python. By the end of reading this tutorial, you’ll be an expert at using Python f strings.
Python String Formatting
Prior to Python 3.6, there were two ways you could format Python string. You could use the percentage (%) formatting, and str.format().
Percentage Formatting
Percentage formatting has been around since the beginning and allows you to format a string with one or multiple values.
Here’s an example of the percentage formatting approach in action:
email = "lindsay.ballantyne@gmail.com" new_string = "Your email address is %s." % email print(new_string)
Our code returns:
Your email address is lindsay.ballantyne@gmail.com
In our code, we use the %s sign as a placeholder for our new string in the “Your email address is %s.” string. At the end of that line of code, we use a percentage sign followed by “email” to replace %s with our user’s email address.
str.format()
In Python 2.6, a new method of formatting strings was introduced: the format() function.
Suppose we want to format a string with two values. We could do so using the format() method like so:
name = "Lindsay Ballantyne" email = "lindsay.ballantyne@gmail.com" new_string = "Hello, {}. Your email address is {}.".format(name, email)
Our code returns:
Hello, Lindsay Ballantyne. Your email address is lindsay.ballantyne@gmail.com.
In our code, we define two Python variables, “name” and “email”. These variables store the name and email address of someone using our program. Then, we use the .format() syntax to add in the values “name” and “email” to our string.
Python f String Format
Python f strings embed expressions into a string literal. You can use f strings to embed variables, strings, or the results of functions into a string. An f string are prefixed with “f” at the start, before the string iitself begins.
Introduced in Python 3.6, make it easier to format strings.
f strings use curly braces to store the values which should be formatted into a string. f strings can also use a capital “F” to represent a formatted string.
Consider this syntax:
f"This is a string."
We have just defined a formatted string literal. We can add values to the string using curly brackets: {}.
Some people refer to string formatting as string interpolation. These two concepts refer to the same idea: adding a value into another string.
Let’s take a look at an example of an f string in action.
f String Python Example
Suppose we want to add the name “Lindsay Ballantyne” to a string. We could do so using this code:
name = "Lindsay Ballantyne" print(f"Your name is {name}.")
Our code returns:
Your name is Lindsay Ballantyne.
In our code, we declare a variable called “name” which stores the name of our user. Then, we use an f string to add the value “Lindsay Ballantyne” into a string.
This shows that you can directly insert the value of a variable into an f string. The syntax is easier than all of the other formatting options, such as the format() method or a % string.
That’s not all. f strings can also support functions or any other expression, evaluated inside the string. Suppose we wanted to perform a Python mathematical function in an f string. We could do so using this code:
name = "Lindsay Ballantyne" print(f"Your name is {name}. On your next birthday, you will be {22 + 1}.")
Our code returns:
Your name is Lindsay Ballantyne. On your next birthday, you will be 23.
We were able to perform a mathematical function.
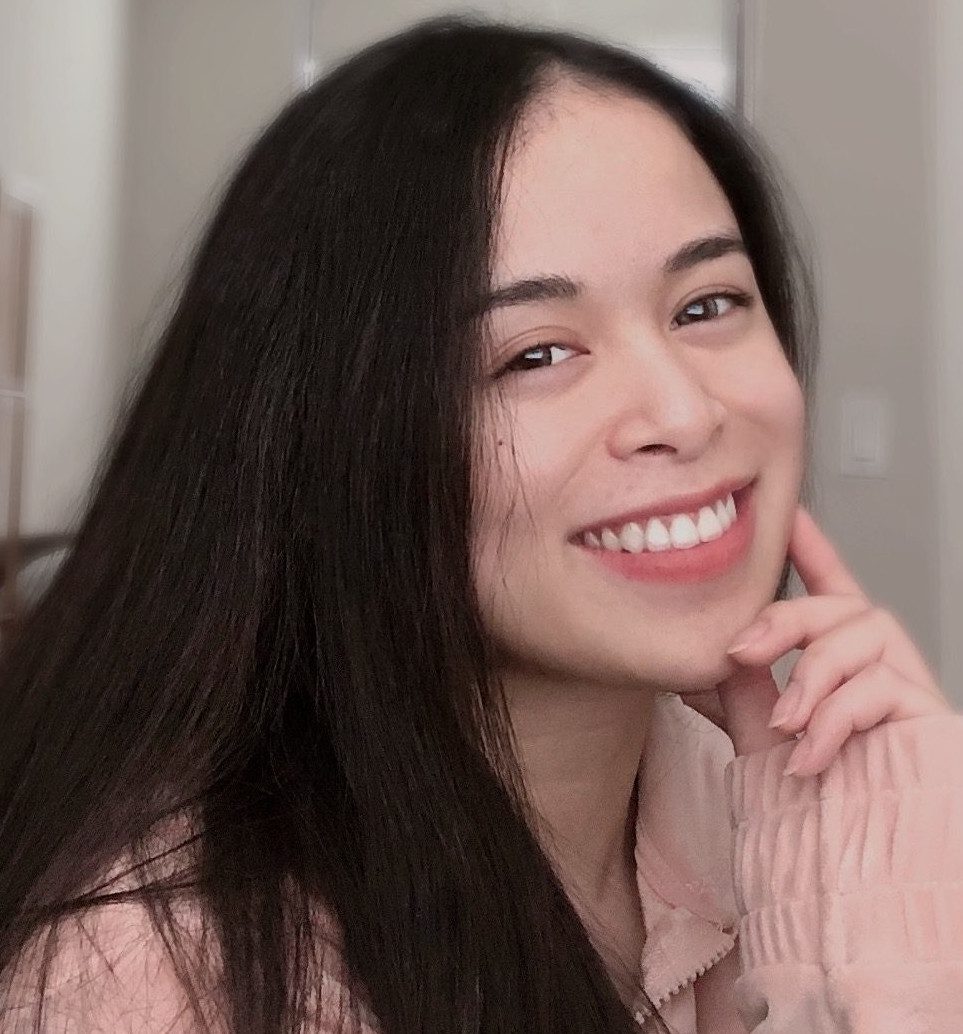
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
This is because expressions in string literals are evaluated at run-time, and they are part of the main program. Our mathematical function was “22 + 1”, which we performed by enclosing the function within curly brackets.
Multiline Python f Strings
The f string syntax supports multiline string formatting. You can create a multiline Python f string by enclosing multiple f strings in curly brackets.
Suppose we wanted to format values in a multiline string. We could do so using this code:
name = "Lindsay Ballantyne" email = "lindsay.ballantyne@gmail.com" age = 22 profile = ( f"Name: {name} \n" f"Email: {email} \n" f"Age: {age} \n" ) print(profile)
Our code returns:
Name: Lindsay Ballantyne Email: lindsay.ballantyne@gmail.com Age: 22
In our code, we declared three variables — name, email, and age — which store information about our user. Then, we created a multiline string which is formatted using those variables.
Notice how, in our code, we placed an f before each line in our multiline string. This is because, if you don’t place an f in front of each line, the f string syntax will not be used.
f String Special Characters
There’s still one thing you need to learn before you start using f strings in your code. You need to know how to handle special characters with f strings.
Here are a few rules you should keep in mind as you use f strings.
Quotation Marks
When you’re using f strings, you can use quotation marks in your expressions. But, you need to use a different type of quotation mark than you are using outside your f string. Here’s an example of quotation marks being used in an f string:
print(f"{'It is Thursday!'}")
Our code returns:
It is Thursday
Notice that we used single quotes (‘) inside our f string (which is denoted using curly braces), and double quotes (“”) to represent our full string.
Dictionaries
To reference a value in a Python dictionary, you’ll need to use quotation marks. You should make sure that you use a different kind of quotation mark when you reference each value in your dictionary.
Here’s an example of working with a dictionary and an f string:
user = {name: "Lindsay Ballantyne", email: "lindsay.ballantyne@gmail.com"} print(f{"Hello, {user['name']}. Your email address is: {user['email']}.")
Our code returns:
Hello, Lindsay Ballantyne. Your email address is: lindsay.ballantyne@gmail.com
Note that, when we’re referring to values in our dictionary, we use single quotes (‘’). Our main f string uses double quotes (“”). This prevents any string formatting errors from arising in our code.
Curly Braces
To use a curly brace in an f string, you need to use double braces. Here’s an example of curly braces in an f string:
print(f"{{This is a test}}")
Our code returns:
{This is a test}
You can see that only one set of braces appeared in our end result. This is because one set of braces is used by the f string to denote that string formatting is going to take place. So, only the one set of braces inside your curly braces will appear.
Comments
When you’re writing an f string, your f string should not include a hashtag (#) sign. This sign denotes a comment in Python, and will cause a syntax error.
If you want to use a hashtag in a string, make sure it is formatted in the string, instead of the f string. Here’s an example of this in action:
user_id = "202" print(f"User ID: #{user_id}")
Our code returns:
User ID: #202
We added our hashtag in before our f string curly braces. We did this to make sure that our hashtag would appear correctly in our string.
View the Repl.it from this tutorial:
Conclusion
Python f strings are a new tool you can use to put expressions inside string literals.
The advantages of using f strings over previous options are numerous. f strings are easier to read. They work well even when you’re working with many values.
This tutorial discussed, with reference to examples, how f strings compare to other string formatting options, and how to use f strings in your code. Now you have the knowledge you need to start working with Python f strings like a professional developer!
If you want to learn more about Python, check out our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.