The most efficient way to learn Python is to write Python code. Practice as many Python exercises as possible until you have perfected the programming language. Even advanced programmers benefit from Python exercises when they hit a wall or experiment with something new.
This article will show you where to get help with Python skills and answer the pressing question, where can I practice Python? Below is a list of some of the best Python exercises for beginners and explanations for why these exercises are important.
Python Exercises to Help You Learn Python
The Internet is full of fantastic Python problems you can solve to understand the programming language better. Practicing Python will help you avoid bad practice anti-patterns and give you proficiency in concepts of programming. You can also learn to make your own encryption program to send and receive secret messages.
10 Python Exercises and Practice Problems (With Solutions)
1. Print-Multiple Arguments
Python arguments are independent variables that pass through a function when they are called. There are arbitrary positional arguments, default arguments, arbitrary keyword arguments, keyword arguments, and positional arguments. This is an important concept to practice if you want to gain functional programming skills.
def CK(name, num): print(“Hello from “, name + ‘, ‘ + num) CK(“Career Karma”, “25”) |
2. Set the Time With Python
This is one of the most common exercises for this dynamic programming language. Use it to test your skills with the pytZ module. It has to do with writing Python lines of code to tell the current time. The term ‘current time’ is used here because the program will be dynamic. This means that the time will change on its own without the need for further coding.
from datetime import * import pytz tz_NEWYORK = pytz.timezone(‘United States of America’) datetime_NEWYORK = datetime.now(tz_NEWYORK) print(“NEWYORK time:”, datetime_NEWYORK.strftime(“%H:%M:%S”)) |
3. Python Input Date Exercise
You can write a simple Python program to create a sequence of dates or one that displays a complete date. It should include the day, month, and year. They all need to have the current status so the output can change every day to suit the current time.
# importing date class from datetime module from datetime import date # creating the date object of today’s date todays_date = date.today() # printing today’s date print(“Current date: “, todays_date) # fetching the current year, month, and day of today print(“Current year:”, todays_date.year) print(“Current month:”, todays_date.month) print(“Current day:”, todays_date.day |
4. Make a Calendar for the Month
Once you’ve mastered setting the current time and date with Python, you can take things up a notch by making your calendar for a month. The code for writing a calendar is similar to the others but generally requires a few tweaks. You can make a calendar for multiple months using the same principle.
import calendar y = int(input(“Input the year: “)) m = int(input(“Input the month: “)) print(calendar.month(y, m)) |
5. Change Time Units Into Seconds
This is another simple Python task to sharpen your Python skills, and it is also an opportunity to learn something new about time. You can write a program to change every unit of time in a day or even a month to seconds.
days = int(input(“Input days: “)) * 3600 * 24 hours = int(input(“Input hours: “)) * 3600 minutes = int(input(“Input minutes: “)) * 60 seconds = int(input(“Input seconds: “)) time = days + hours + minutes + seconds print(“The amounts of seconds”, time) |
6. Write a Body Max Index (BMI) Calculator
A body mass index calculator is one of the most popular health checkers. BMI is based on a person’s height and weight. You can write software to calculate BMI to check if someone would qualify as overweight, obese, or underweight.
height = float(input(“Input your height in Feet: “))weight = float(input(“Input your weight in Kilogram: “))print(“Your body mass index is: “, round(weight / (height * height), 2)) |
7. Sum Up Tuple Elements
Python can be used for anything including a dashboard with figures that tuple elements. With the right code, you can automatically get the sum of tuple elements that are immutable.
# Python3 code to demonstrate working of # Tuple summation # Using list() + sum() # initializing tup test_tup = (7, 8, 9, 1, 10, 7) # printing original tuple print(“The original tuple is : ” + str(test_tup)) # Tuple elements inversions # Using list() + sum() res = sum(list(test_tup)) # printing result print(“The summation of tuple elements are : ” + str(res)) |
8. Matrix Grouping of Python Elements
Matrix grouping of elements is a common Python practice that you can use to improve your Python programming skills. Grouping elements with similar attributes is a great way to solve any rendering problem that may occur due to misrepresentation.
# Python3 code to demonstrate working of # Group similar elements into Matrix # Using list comprehension + groupby() from itertools import groupby # initializing list test_list = [1, 3, 5, 1, 3, 2, 5, 4, 2] # printing original list print(“The original list : ” + str(test_list)) # Group similar elements into Matrix # Using list comprehension + groupby() res = [list(val) for key, val in groupby(sorted(test_list))] # printing result print(“Matrix after grouping : ” + str(res)) |
9. Reverse Words in a Python String
One of the most important things you will learn in Python is how to manipulate strings. A string is a data type that is used to store elements and used to represent text. Unlike lists, strings cannot be changed. However, you can write a program to reverse string methods.
# Function to reverse words of string def rev_sentence(sentence): # first split the string into words words = sentence.split(‘ ‘) # then reverse the split string list and join using space reverse_sentence = ‘ ‘.join(reversed(words)) # finally return the joined string return reverse_sentence if __name__ == “__main__”: input = ‘geeks quiz practice code’ print (rev_sentence(input)) |
10. Make an Empty Class With Python
Classes in Python programming are vital for creating objects in object-oriented programming. Think of the class as your template that describes all the objects within. When a class is empty, it does not contain data members yet. Without the right class, you will receive a syntax error. You’ll need to understand classes before you move on to more advanced topics.
# Python program to demonstrate # empty class class Career Karma: pass # Driver’s code obj = Career Karma() print(obj) |
How to Get Help with Python
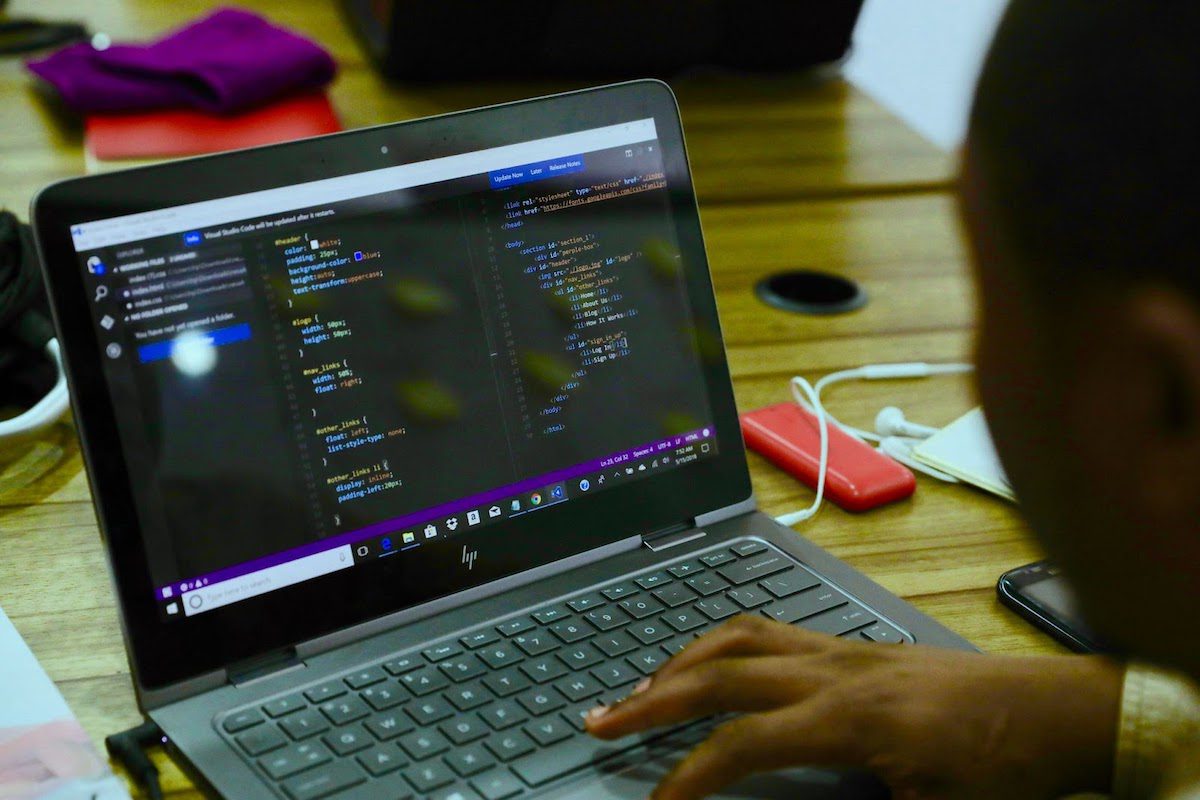
Python exercises can help you understand the difference between crucial coding concepts like loops, strings, and arrays, and will help you develop your skill if you’re a beginner. Once your skillset develops, you could be building an arcade game or delving into AI machine learning. Understanding the fundamentals will guide you into mastering Python.
Python Exercises
Python exercises are simple codes or instructions written with a code editor. There is no limit to the number of exercises you can do, and there are several online resources with exercise ideas. The more advanced your knowledge of Python becomes, the more complex your exercises will be.
Python Projects
While exercises are simple instructions, projects involve creating complete Python software. For example, you can use Python for game development or the backend programming language for an ecommerce platform. All your Python projects can be hosted publicly or privately on GitHub, the world’s largest Git repository.
Python Quizzes
Quizzes are interactive ways to learn Python. It makes the process more engaging and challenging. All you have to do is find a website that hosts Python quizzes and find the solution to those quizzes. You can make it more interactive by joining the official Python forum and taking quizzes with other Python developers.
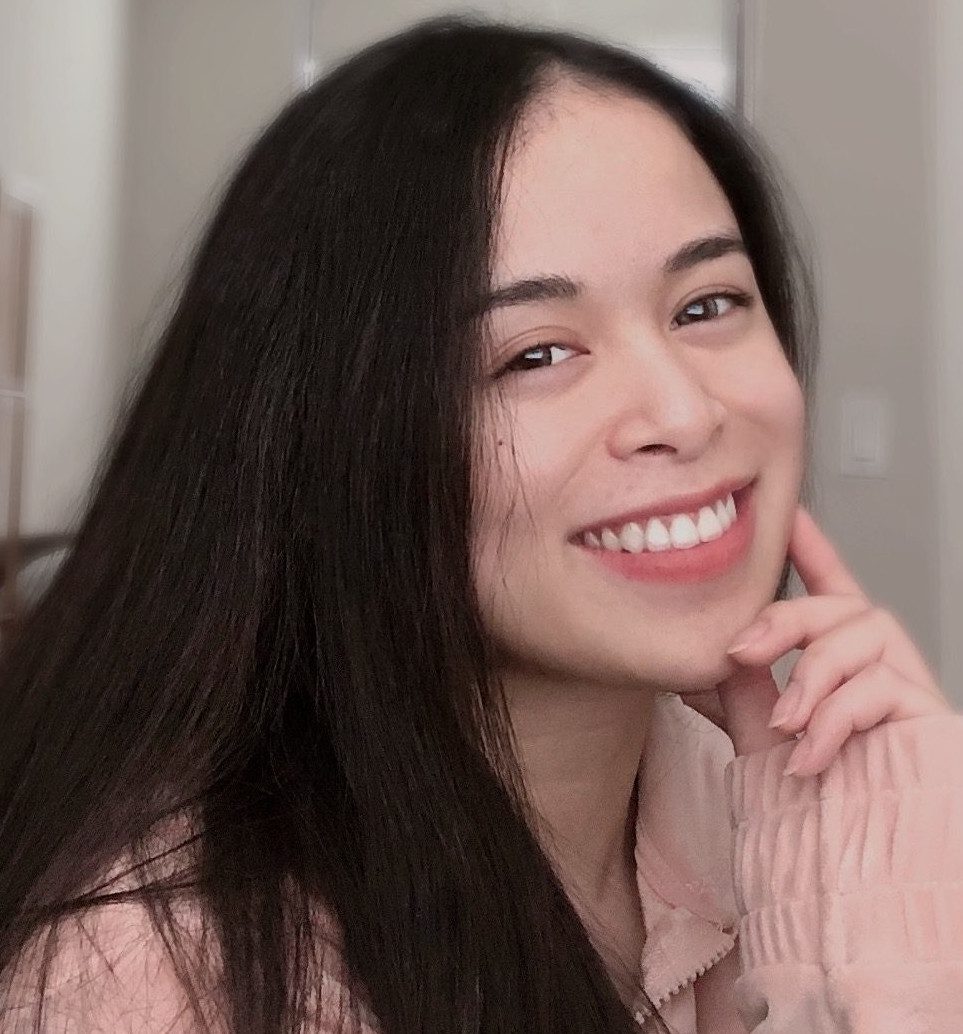
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Python Forums and Blogs
Forums are vast and ever-growing communities that provide opportunities to learn about Python. Apart from the official Python forum, other great options are PythonAnywhere, Stack Overflow, Source, Python Forums, and Reddit. Blogs also provide a wealth of information about Python.
Where Can I Practice Python?
You can practice Python in an online text editor or integrated development environment. There are also interactive websites that allow you to practice your Python skills. As it is such a popular programming language, there are many resources out there to help you gain a deeper understanding of Python.
Websites to Practice Python
- Edabit. Edabit is an interactive platform that allows you to practice Python at different difficulty levels. Apart from the Python challenges, tutorials are available to those who need more information. There are also sample Python problems.
- Practice Python. This platform had over thirty-six beginner Python exercises at the time of writing. New exercises are posted at intervals. The archive of programming problems also has free resources for learners.
- HackerRank. HackerRank is more than a platform to master Python. It is also a bootcamp for extensive Python lessons for beginners and intermediate developers. As for the challenges, you can choose from easy, medium, or hard depending on your current programming level.
- The Python Tutorial. This is a vast resource containing information on all the essential basics of Python that you need to practice your skills. You can learn about Python libraries and there is a glossary to refer to if you get stuck.
- Geeksforgeeks. This is another incredible resource for Python programmers. It has several categories of Python exercises with different difficulty levels. All you have to do is pick the ones you want. There are also sample solutions available for each one.
What’s the Best Way to Learn Python?
The best way to learn Python is to practice writing Python code and answer detailed programming questions. Python is one of the easiest languages to learn because its syntax is full of human-readable language. Learn the best practices to avoid bugs in code by keeping things simple and creating code that you can test frequently.
Python Exercises FAQ
Yes, you can learn Python by yourself and become a professional developer. The timeline for learning will vary depending on your current experience with programming. Someone who already knows how to code will learn Python faster than someone with no experience.
Beginners can practice Python by doing online quizzes and beginner exercises. Search for exercises on websites like Edabit, Practice Python, HackerRank, and Geeksforgeeks.
Udemy, Codecademy, and Coursera are some of the best places to learn Python online. Google and Microsoft also provide Python free classes for beginners and intermediate developers.
Learning Python usually takes between two to six months, depending on the level of experience and method of teaching. Most Python bootcamps run for three months full-time, but self-paced learning may require more time.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.