The Python dictionary values() method returns an object containing the values in a dictionary. This method is useful if you want to iterate over only the values in the dictionary. If you change a dictionary later, the data stored in the values() method will change to reflect the new dictionary.
When you’re working with Python, you may decide to store data in a dictionary. For instance, suppose you are building a restaurant management system. You may want to use a dictionary to store data about each order.
You may be wondering: How can I retrieve the values stored in a dictionary? That’s where the Python dictionary values()method comes in. The values() method returns a list of all the values stored in a dictionary.
This tutorial will discuss, with reference to examples, the basics of Python dictionaries and how to use the values() method.
Python Dictionary values()
The Python dictionary values() method returns a list of the values in a dictionary. values() accepts no arguments. You specify values() after the name of a dictionary.
Let’s take a look at the syntax for this method:
dict_name = { "name1": "value1" } dict_name.values()
As you can see, the built-in function does not accept any parameters. Instead, the method is appended to the end of a dictionary.
values() does not return a list. It returns a dict_values object. To convert the values into a regular list, you can use the Python list() method:
dict_name = { "name1": "value1" } new_list = list(dict_name.values())
The value of new_list is a regular list of all the values in the dictionary we have specified. This list is not a dict_values object, unlike the one created from our first syntax definition.
Alternatively, you can keep the dict_values object and iterate over it.
The values() method is useful commonly used with a Python for loop. When used with a for loop, you can iterate over every value in a dictionary. For instance, you could check if each key in the dictionary has a value.
Dictionary values() Python Example
We have a Python dictionary that stores information about a student at a school.
simon = { 'name': 'Simon Henderson', 'birthday': '02/09/2002', 'class': 'Senior Year', 'honor_roll': True }
name is a key, and its associated value is Simon Henderson. birthday is also a key, and has been assigned the value 02/09/2002. We also store information on the students’ class and whether they are on the school’s honor roll.
Suppose we want to retrieve a list of all the values stored in our dictionary. This would let us work with each value without having to deal with the dictionary keys. We could retrieve these values using the values() method:
simon = { 'name': 'Simon Henderson', 'birthday': '02/09/2002', 'class': 'Senior Year', 'honor_roll': True } print(simon.values())
Our code returns:
dict_values([‘Simon Henderson’, ’02/09/2002′, ‘Senior Year’, True])
First, we declare a dictionary with four keys and values. The name of this dictionary is simon. Then, we retrieve the values stored in our dictionary using the values() method and print them out to the console.
Our code returns a dict_values object that contains a list of all the values in our dictionary.
Iterating Using a for Loop
Suppose we want to print out a list of all the values in our dictionary to the console. Each value appearing on a new line. This is where a for loop comes in handy.
We can use a for loop to iterate over every item in our dict_values object and print each item to the console:
simon = { 'name': 'Simon Henderson', 'birthday': '02/09/2002', 'class': 'Senior Year', 'honor_roll': True } for item in simon.values(): print(item)
Our code returns:
Simon Henderson 02/09/2002 Senior Year True
Because the values() method returns a dict_object with a list of our keys, we can iterate over it using a for loop.
In this example, we created a for loop which runs through every value in the simon dictionary. Each time the for loop is executed, a value from the dictionary is printed to the console until all values have been traversed.
After all values have been printed to the console, our code stops executing
Validating Data Using a for Loop
We could use a for loop and the values() method to validate whether our dictionary has any blank strings.
Consider the following code:
simon = { 'name': 'Simon Henderson', 'birthday': '02/09/2002', 'class': 'Senior Year', 'honor_roll': True } blank = 0 for item in simon.values(): if item == "": blank += 1 print("There are {} blank values in the dictionary.".format(blank))
We declare a Python variable called blank. This variable tracks how many blank values there are in our dictionary.
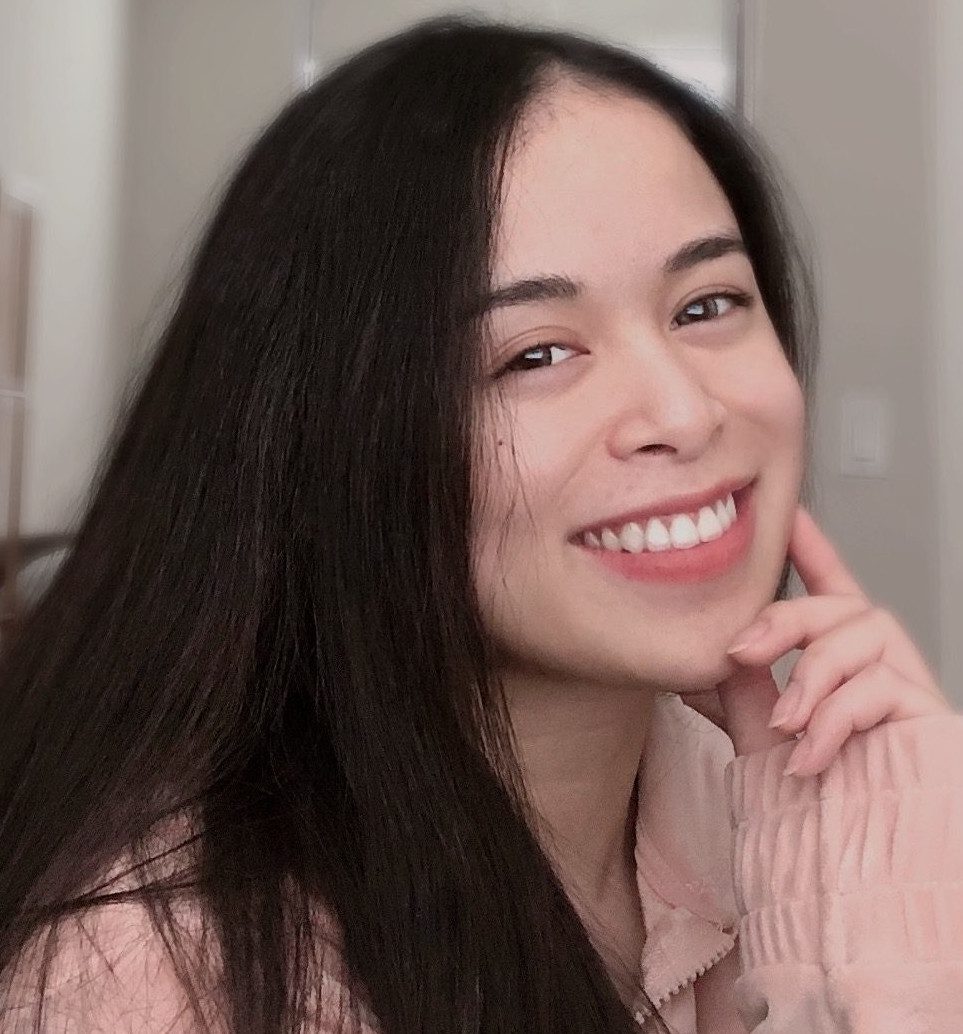
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
We use a Python if statement in our for loop to evaluate whether any item has the value “”. This value is a blank string. If there is a blank string in our list, our code adds 1 to our “blank” variable.
Once our loop has executed, our code tells us how many blank values are in the dictionary. We use a Python format() statement to add the number of blank values into the string we print to the console.
Conclusion
The Python dictionary values() method retrieves the values in a dictionary. The values are formatted in a dict_values object. You can read each value returned by the values() method using a for loop.
This tutorial discussed how you can use the dictionary.values() method to retrieve the values in a dictionary. Now you’re ready to start working with the values() method like a Python expert!
Do you want to learn more about Python? Read our How to Learn Python guide. This guide contains a list of curated courses, learning tips, books, and resources you can use to advance your knowledge of Python.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.