The Python dictionary keys() method returns an object which contains the keys in a dictionary. You can use the list() method to convert the object into a list. The syntax for the keys() method is: dictionary.keys().
You may want to retrieve a list of all the keys stored in a dictionary.
For instance, say you have a dictionary with information on a book in a library. You may want to retrieve all the keys so you know what kind of information you store.
That’s where the dictionary keys() method comes in. This tutorial will discuss, with reference to examples, how to use the dictionary keys() method in Python.
Python Dictionary keys()
The Python dictionary keys() method returns all the keys in a dictionary. This method returns keys as a special object over which you can iterate. keys() accepts no arguments.
The syntax for the keys() method is:
dict_name.keys()
We add the keys() method after the dictionary name.
The keys() method returns a view object rather than a list of keys. This object is iterable so you can view its contents using a for statement. But, it may be necessary to convert this object to a list.
Suppose you want to print all the keys in the dictionary to the console. You should convert the keys to a list to make the output more readable:
key_list = list(dictionary.keys()) print(key_list)
This code returns a list of keys. We know our data is in a list because the values are enclosed within square brackets.
Alternatively, say you want to find out the first key in the dictionary. You can do this using indexing. But, you would first need to convert the collection of keys to a list:
key_list = list(dictionary.keys()) print(key_list[0])
This code finds the key at the index position 0 in the list of dict keys.
Dictionary keys() Python Example
We have a dictionary that stores information about an order at a bakery. This dictionary stores three keys: product, price, and mayo. Each of these keys has a value.
We want to know what information we collect about each order. This is because the bakery is considering adding new information to each order ticket. Before they do so, they want to know all the information they collect.
To retrieve a list of keys in the dictionary, we can use the keys() method:
order = { 'product': 'Ham Sandwich', 'price': 2.10, 'mayo': False } order_keys = order.keys() print(order_keys)
Our code returns:
['product','price','mayo']
First, we have defined a Python dictionary called order. Then, we use order.keys() to retrieve a list of keys in the dictionary. We assign this list to the Python variable order_keys. Next, we print out the value of the order_keys variable to the console.
Our code returns all the keys in the dictionary.
Updating the Dictionary
The keys() method keeps up to date as we add new keys to our dictionary.
Suppose we add a new key to our dictionary which stores whether the customer paid for their bakery order using a gift card. Here is the code we could use to add this key:
order = { 'product': 'Ham Sandwich', 'price': 2.10, 'mayo': False } order_keys = order.keys() order["gift_card"] = False print(order_keys)
We use the indexing notation to add a “gift_card” key which is associated with the value False. This statement comes after we have assigned the list of keys to the order_keys variable.
Our code returns:
['product','price','mayo','gift_card']
We have added one new line. This line creates a new item in our dictionary with the key name gift_card and the value False.
Then, we print out the contents of the order_keys variable to the console. Although we retrieved the keys in our dictionary using order.keys() before we added our new value, our program still kept track of the change.
Even after you add new keys, the keys() method will store an up-to-date list of all the keys in a dictionary.
Check if Key in Dictionary Python
One common use of the keys() method is to check whether a key is in a dictionary. We can check if a key is in a dictionary using the Python in statement. This statement is often used with an if statement.
Suppose we want to check whether the key mayo is stored in our order dictionary. We could do so using this code:
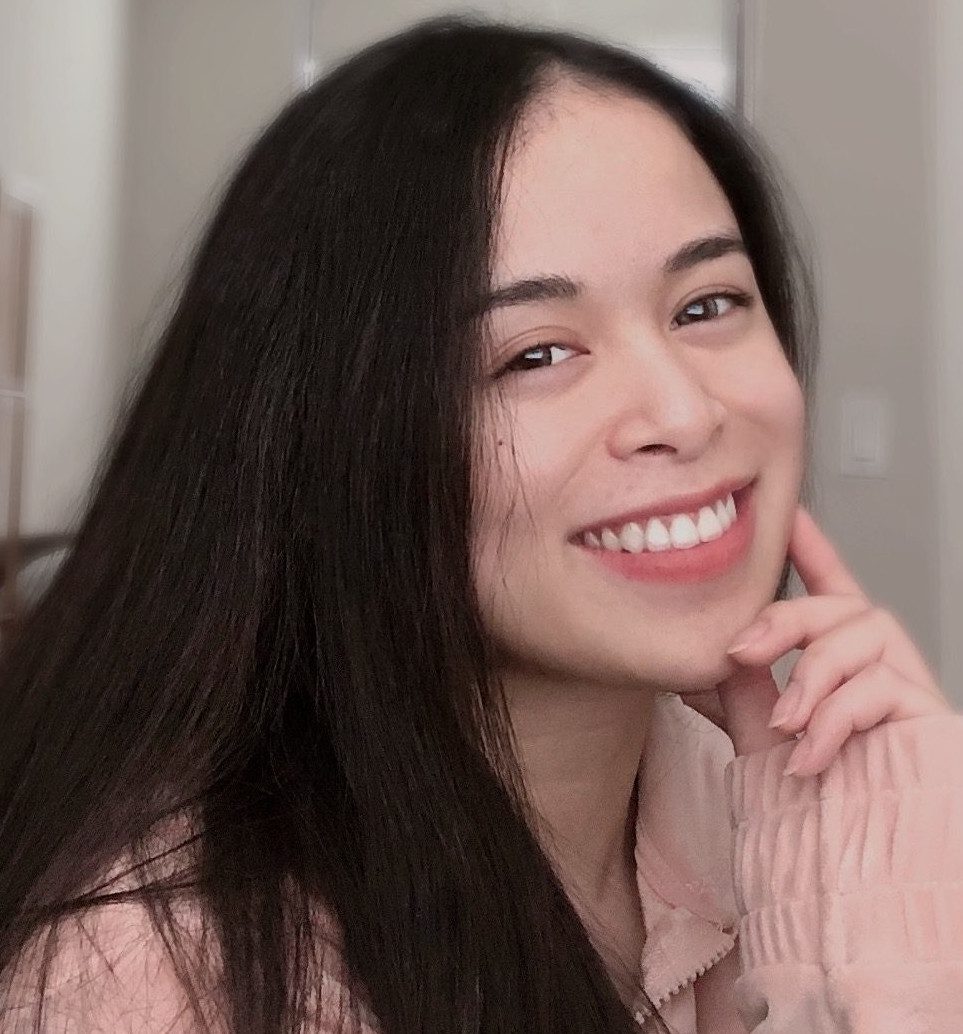
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
order = { 'product': 'Ham Sandwich', 'price': 2.10, 'mayo': False } if "mayo" in order.keys(): print("The 'mayo' key is in the order dictionary.") else: print("The 'mayo' key is not in the order dictionary")
Our code returns:
"The 'mayo' key is in the order dictionary."
In our code, we first define our order dictionary. We use a Python if…in statement to check whether the mayo key can be found in the list of keys generated by order.keys().
mayo is a key in the order dictionary. So, our if statement evaluates to true and the code in our if statement runs. If we were to remove the mayo key, the following response would be returned:
"The 'mayo' key is not in the order dictionary.
Our code successfully realizes that mayo is no longer a key in the dictionary. So, the contents of our else statement run.
Conclusion
The dictionary keys() method retrieves a list of the keys in a Python dictionary. When used with an if…in statement, the keys() method allows you to check if a key exists in a Python dictionary. The keys() method accepts no arguments.
Do you want to learn more about coding in Python? Read our How to Learn Python guide. You’ll find expert learning advice and guidance on top online courses and Python resources.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.