A Python dictionary stores data in key-value pairs. A key is a label for a value. Dictionaries are declared as a set of keys and values between a pair of curly brackets. The syntax for a key and value is: “key_name”: value.
When you take a look at a dictionary (Merriam-Webster’s, Oxford), what do you notice? It’s a book or list in an app of words and their corresponding definitions and etymology:
careerkarma
noun ca·reer·kar·ma | \ kə-ˈrir-ˈkär-mə \
Definition
Definitions go here
Etymology
Etymology and/or other information goes here
In Python, dictionaries are very similar. A dictionary in Python is a data structure that holds key/value pairs together to tell us something about the structure. This article takes a look at dictionaries and some of their built-in functions.
What is a Python Dictionary?
A Python dictionary is key-value pairs, separated by commas, and are enclosed in curly braces. Dictionary keys must be string labels. A value can contain any data type, such as a string, an integer, or another dictionary.
Dictionaries are unordered: if you want to control the order in a dictionary, use a list with square brackets instead
To create a dictionary, you need to create a variable and set it equal to two curly braces. The variable can be whatever you would like to name it – in these examples we are naming it “dictionary”.
dictionary = {} print(dictionary)
Here is an example dictionary with some values:
word = { "name": "banana", "definition": "a yellow fruit" }
Our dictionary contains two keys and values. “name” and “definition” are keys and the strings that come after them are our values.
Duplicate keys cannot exist in a dictionary. Each key must have a unique name.
Python Add to Dictionary
Right now, if we were to print this, it would be an empty dictionary. Let’s add some stuff to it:
dictionary = {} dictionary["name"] = "Christina" dictionary["favorite_animal"] = "cat" dictionary["num_pets"] = 1 print(dictionary)
We use the bracket notation to create the key/value pairs. Inside the brackets is a string that identifies our dictionary key. What the dictionary key is assigned to is the value of that key.
We can use the same notation to update the dictionary as well. It’ll just overwrite the previous value.
Access Items in a Dictionary
We use the same notation to access items present in a dictionary.
dictionary = {} dictionary["name"] = "Christina" dictionary["favorite_animal"] = "cat" dictionary["num_pets"] = 1 print(dictionary["name"])
Using dictionary[“name”] accesses only the name property of the dictionary and prints that in the interpreter.
You can also use the Python dictionary get() method to retrieve an item from a dictionary:
dictionary = {} dictionary["name"] = "Christina" dictionary["favorite_animal"] = "cat" dictionary["num_pets"] = 1 print(dictionary.get("name"))
This code prints out the value of the “name” key, which is “Christina”.
Python Remove from Dictionary
There are essentially three different ways to remove a key from a dictionary: the individual entry, all the entries, or the entire dictionary.
The first method removes just the entry. We do that by accessing the key we would like to get rid of using bracket notation: del dictionary[“<key name>”]
Here’s an example using the del statement with the key name to delete the key we have specified:
dictionary = {} dictionary["name"] = "Christina" dictionary["favorite_animal"] = "cat" dictionary["num_pets"] = 1 print(dictionary) # remove entry with key 'name' del dictionary["name"] print(dictionary) # {'favorite_animal': 'cat', 'num_pets': 1}
The second method removes all the entries in the dictionary. We do that with the method dictionary.clear(). Remember that this dictionary method is that it merely clears out the dictionary. The instance of the dictionary still remains so it can be used again.
Here’s an example using the clear() method:
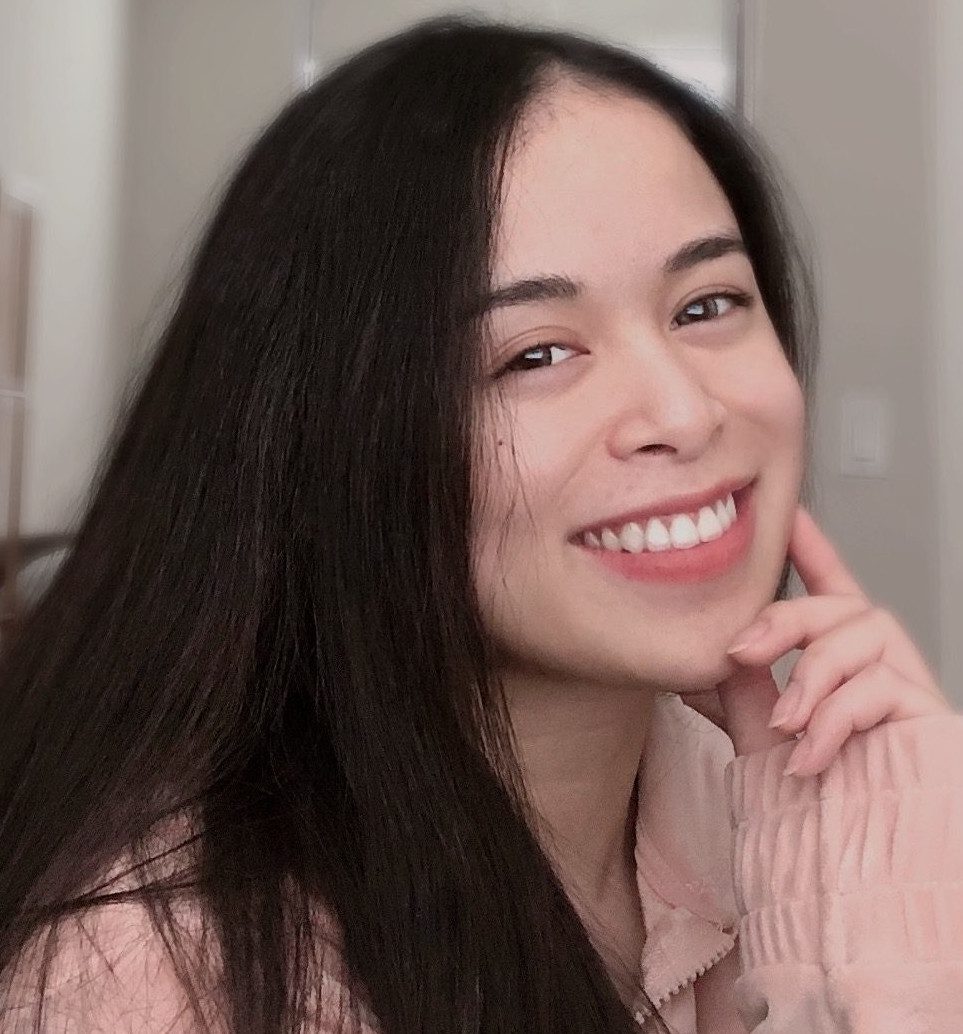
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
# remove all entries in dict dictionary.clear() print(dictionary) # {}
The last method gets rid of the entire instance of the dictionary. If we try to access the dictionary after it has been deleted, it will throw an error letting you know that it doesn’t exist. Do this by using the del keyword: del dictionary.
Here’s an example using the del keyword:
# delete entire dictionary del dictionary print(dictionary)
Your code will throw KeyError exceptions if you try to access a dictionary item that does not exist using this syntax. You can read about how to solve a KeyError in our Python KeyError guide.
oop Through Dictionary
You can use a for…in loop to loop through dictionaries in Python:
for key in dictionary: print(f'{key}: {dictionary[key]}')
Python Dictionary Methods Glossary
We’ve prepared a list of functions often used with the Python dictionary data type. This list should help you learn how you can manipulate your dictionaries in your code.
Dictionary functions:
len(dictionary1): Gives the total length of the dictionary. This is equal to the number of key-value pairs in the dictionary.
str(dictionary1): Produces a printable string representation of a dictionary. This is comparable to the JavaScript JSON stringify method, if you are used to using JavaScript.
type(variable): Returns the type of the passed variable. If the passed variable is a dictionary, it will return a dictionary type.
Dictionary built-in methods
dictionary.clear(): Removes all elements of dictionary1. Leaves the actual dictionary intact so that it can still be used. It’s just an empty dictionary.
dictionary.copy(): Returns a shallow copy of dictionary dict. Does not mutate the original dictionary.
dictionary.fromkeys(set(), value): Creates a new dictionary with the parameters passed into set(), and value.
dictionary.get(key, default = None): For key, returns the value or default if the key is not in the dictionary.
dictionary.has_key(key) : Returns true if a key exists in the dictionary and false if otherwise.
dictionary.items(): Returns dictionary’s (key, value) tuple pairs in a list ([ (key1, value1), (key2, value2), etc.])
dictionary.keys(): Returns a list of keys.
dictionary.pop(‘name’): Removes the key/value pair from the dictionary. The removed item is the pop method’s return value.
dictionary.setdefault(key, default = None): Similar to get(), but it will set dictionary1[key]=default if the key does not already exist in the dictionary.
dictionary.update(dictionary2) : Merges dictionary2’s key-values pairs with dictionary1.
dictionary.values(): Returns list of dictionary values.
Use the built-in methods and functions listed above to play with the sample dictionaries outlined below.
Conclusion
A Python dictionary lets you store related values together. Data in a dictionary is stored using key-value pairs. You can add as many key value pairs in a dictionary as you want.
Python dictionaries can be a little confusing at first, but with time and effort you will be an expert in no time!
As a challenge, try to declare a dictionary with the following keys and values:
- lentil: 3.00
- vegetable: 2.90
- pea: 3.10
Then, use the bracket notation to print the value of “lentil” to the console. For an extra challenge, delete the value of “pea” from the dictionary. Then, print the dictionary to the console to make sure the value has been deleted.
To learn more about how to code in the Python programming language, check out our How to Learn Python guide.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.