Python queue is a built in library that allows you to create a list that uses the FIFO rule, first in first out. Python deque uses the opposite rule, LIFO queue, or last in first out. Both operate on stacks and queues.
When you’re working in Python, you may want to create a queue of items instead of a list. For example, let’s say you were writing a program that tracks registrations to a conference. When we add someone to the list, you want to place them at the back of the queue, and then you want them to advance through the queue as people at the front start to get in.
There is a built-in library in Python designed to help you with these types of problems: queues. Queues are similar to stacks in Python, with the main difference being that with a queue, you remove the item least recently added. In a stack, on the other hand, you remove the item most recently added.
In this tutorial, we are going to break down the basics of queues in Python and how you can implement one.
Queue Primer
Helpful when working with a Python list, queues are useful when you want to get things out of a list in the order that you put them in. To use our previous example, when you’re at a conference, you should be placed at the back of the line when you first register. But as people on the list start to get in, you should move up further on the list.
Queues are different from arrays and lists in that queues are not random access—the data stored in a queue has a particular order. So if you want to add an item to a queue, it will be added to the end. This is called first-in
, first-out
, or a FIFO queue for short.
In Python, you can use a standard list as a queue. However, lists can be quite slow if you are inserting and removing elements because changing elements at the start of the list requires moving all the other elements in the list down. Therefore, if you need to implement a first-in, last-out list, you should use a queue.
Python Queue
So, how do you implement a queue in Python? In order to do so, we have to make use of the built-in queue library. The queue module includes a number of useful classes for queues, but for this tutorial we are going to focus on the queue.Queue
class.
Let’s say that we are building a program that tracks people who want to go and see the latest movie at the local theater. We could use a queue to keep track of the waitlist of people who want to watch the movie.
Firstly, we need to define our queue class. We can do that using the following code:
from queue import Queue waitlist = Queue()
Now we’re ready to create our queue. The put()
function allows data to be put
into the queue. In the below code, we are going to add five people to the waitlist who have just signed up to see the movie:
waitlist.put('Erin') waitlist.put('Samantha') waitlist.put('Joe') waitlist.put('Martin') waitlist.put('Helena')
Now we have added to the queue our five names. Erin is first in our queue, then Samantha, and so on until we reach Helena, who is last. We can demonstrate this by using the get()
function, like so:
print(waitlist.get())
Our code returns the following:
Erin
As you can see, Erin is the first in our queue. If we wanted to print out the first two names that are in the queue, we would use the get()
function twice:
print(waitlist.get()) print(waitlist.get())
Our code returns the following:
Erin Samantha
Python Deque Example
But what if we want to add and remove a number of items from either end of our queue? This is where the deque function comes in. By using deque, we can create a double-ended queue where we can add and remove elements from the start or end of the queue. Deques are last-in
, first-out
, or LIFO for short.
Let’s use the same example as above: storing waitlisted names for a movie. Firstly, we’ll declare our deque function:
from collections import deque waitlist = deque()
Now we have initialized our deque, we can add our list of waitlisted names to our deque:
waitlist.append('Erin') waitlist.append('Samantha') waitlist.append('Joe') waitlist.append('Martin') waitlist.append('Helena')
As you can see, we used the append()
function to put an item into our queue. To see the values stored in our waitlist, we can use the following code:
print(waitlist)
Our code returns the following:
deque(['Erin', 'Samantha', 'Joe', 'Martin', 'Helena'])
As you can see, our data has been stored in the order we inserted it into our deque. But what if we want to remove the first item from our queue? We can use the popleft()
function to accomplish this goal. Here’s an example:
waitlist.popleft() print(waitlist)
Our code has removed the first item in our list — Erin
— and returns the following:
deque(['Samantha', 'Joe', 'Martin', 'Helena'])
If we want to remove all of the items in our deque, we can use the clear() function:
deque.clear() print(waitlist)
The result of our code is as follows:
deque([])
As you can see, our deque is empty, but the object still exists.
Conclusion
That’s it! In this article, we have discussed how queues are a type of data structure that allows you to adopt a first-in, first-out storage approach to storing data. One example use of a queue would be to keep a waitlist for a new product.
We also discussed how you could use deque to create a double-ended queue where you can add and remove elements from your queue. Now you’re ready to start writing your own queries and deques!
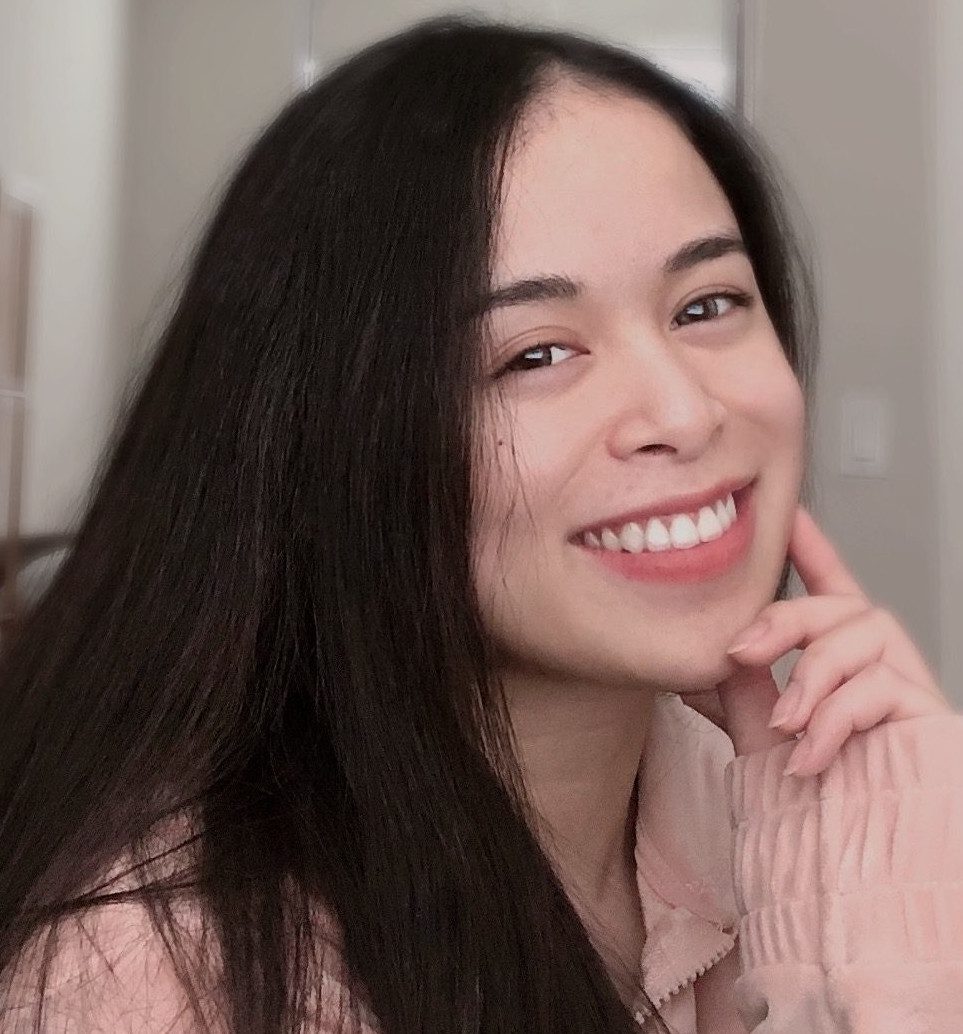
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Python is used in a variety of professional programming environments. Download the free Career Karma app today to learn about how Python can help you break into your dream career in tech!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.