Data types in Python are the different formats in which Python stores data. Some Python data types are tuples, floats, strings, and lists. Each data type has it’s own rules and uses and can store different data.
Data types are used to store a particular type of data in programming and include numbers, strings, and lists. Using the right data type is important because each type of data has its own rules and operations. So, if you use the wrong data type, you may not be able to perform certain functions on a string.
Python includes a number of built-in data types that can be used to store data. In this tutorial, we are going to explore the most commonly used data types in Python.
It’s important to note that this article is not a comprehensive guide to these data types—each one has many different features—but by the end of reading this you should be equipped with the knowledge you need to work with Python data types.
Strings
Strings are sequences of one or more characters and can include letters, numbers, symbols, and spaces. Strings in Python are declared within single quotes (‘’
) or double quotes (“”
), and should start and end with the same type of quote.
Here’s an example of a string in Python:
'This is an example string!'
Like any type of data, Python strings can be assigned to a variable. This is useful if we want to store our data for future use in our program. Here’s an example of a Python variable that contains a string:
example_string = 'This is an example string!'
Strings are used to store text values in Python. The string data type also features a number of operations that can be used to manipulate our text, such as string concatenation and string splits and joins.
Numbers
Python includes two data types that can be used to represent numbers: integers and floats. Integers are Python numbers without decimals, whereas floats are complex numbers with decimals.
When you enter a number in Python, it will automatically assign it to the right data type.
Integers
Integers are whole numbers that can be assigned any positive or negative value. Integers are commonly referred to as int
in Python, and do not include commas in larger numbers.
Here’s an example of an integer in Python:
print(5)
Our program returns: 5.
In addition, you can perform mathematical functions on integers. Below is an example of a basic addition calculation in Python:
example_addition = 10 + 10 print(example_addition)
Our program returns: 20.
Floats
Floats, or floating-point numbers, are real numbers. This means that they can store decimal and fractional values, unlike integers. In simple terms, floats can be used to store numbers that contain decimal points.
Here’s an example of a float in Python:
print(2.5)
Our code returns: 2.5.
Similar to integers, we can run mathematical calculations on our integers as well. So, if we wanted to add two decimal numbers, we could do so using the following code:
example_decimal_addition = 10.9 + 22.2
Our code returns: 33.1.
Boolean
Booleans can be used to store data that has one of two values. The Boolean data type can either be assigned a True or False value and are used in situations where something can only have one of two states.
Here’s an example of a Boolean in Python:
example_boolean = True
Notice that our Boolean value True
is capitalized. This is because True and False are special values in Python, and so whenever you use them, you should use capital letters.
Booleans are important because they allow us to evaluate whether or not a condition is met in a program. For example, let’s say you are a teacher who wants to figure out which of two students earned the highest grade. You could use the following statement to perform that action:
alex = 9 sophie = 10 highest_grade = alex > sophie print(highest_grade)
Our code returns: False.
As you can see, our program has compared the grades of Alex and Sophie. Our program evaluated whether Alex’s grade was greater than Sophie’s grade, and because Sophie scored higher in the test, our code returned False
.
Booleans are often used in statements that compare values. For example, if you want to find out whether a value is less than, greater than, or equal to another value, you may evaluate the values and store the response in a Boolean.
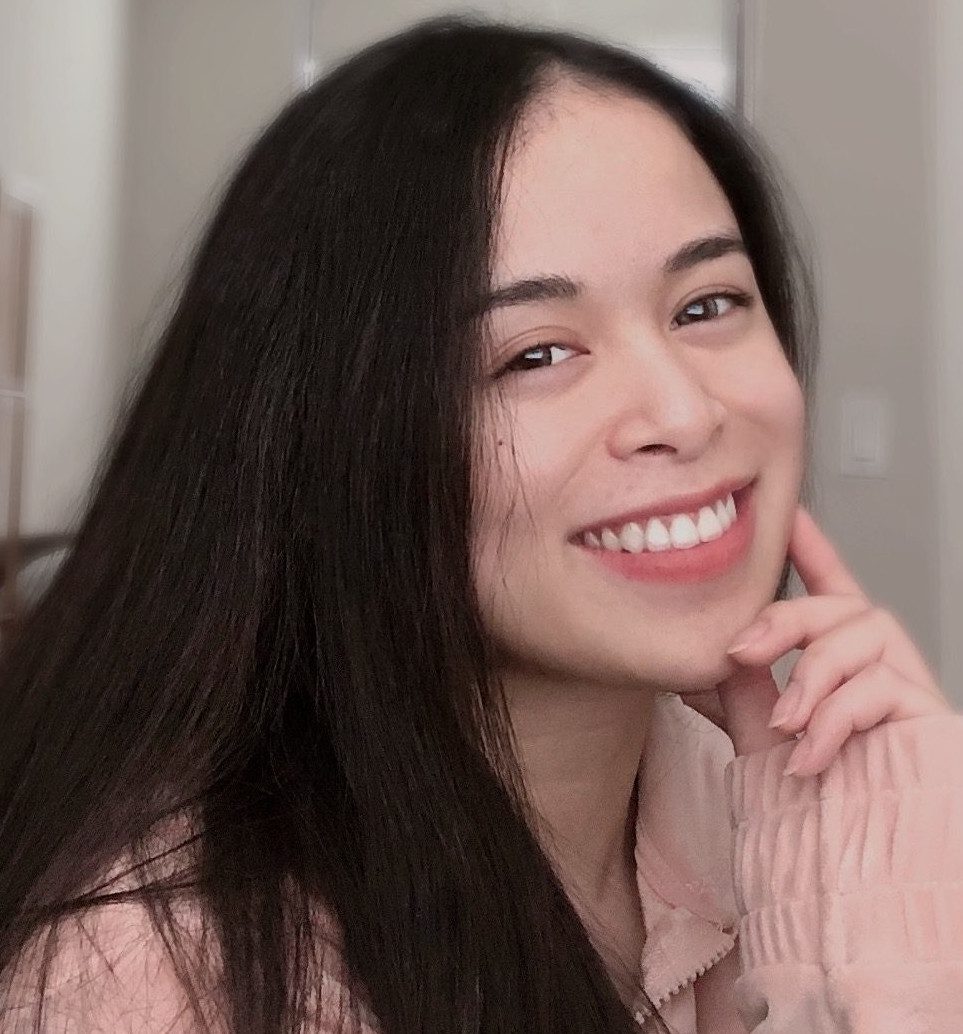
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Lists
Lists are ordered sequences of elements, or items
. Lists are also mutable, which means they can be changed. In Python, lists are defined by enclosing a set of comma-separated values within square brackets.
Here’s an example of a list of student names in Python:
students = ['Dale', 'Chloe', 'Alice', 'Jim']
Lists can store any data type. So, if we wanted to store a list of booleans or floats, we could do so by enclosing it within square brackets. Here’s an example of a list of integers:
student_grades = [25, 28, 22, 24]
Lists are a useful data type because they allow you to store sequences of values in one variable. So, you don’t have to declare multiple variables to store different values. In addition, lists can be changed, which means that if you need to manipulate the values in a list, you are able to do so.
Tuples
Tuples are ordered sequences of elements. Unlike lists, however, tuples are immutable, so you cannot change the contents of a tuple. Tuples are declared as a list of comma-separated values enclosed within parentheses (())
.
Here’s an example of a tuple in Python:
desserts = ('Chocolate Cake', 'Toffee Brownie', Apple Pie')
Elements can be added or removed from a tuple, but the exact values stored in a tuple cannot be changed. This data type is useful if you have a list of items you want to store, but that you do not want to change later.
Dictionaries
Python dictionaries are collections of items that are unordered, indexed and mutable. This means that there is no specific order to the items in a dictionary, and they have their own index values (or keys
) that can be used to reference individual elements. In addition, the contents of a dictionary are changeable.
Dictionaries are declared as a list of values enclosed within curly braces ({}
).
Dictionaries are often used to hold data that are related. Let’s say you own a shoe store and want to store the brand name, shoe name, and prices of shoes in your inventory. Here’s an example of a dictionary that would store one of these shoes:
jordan_6s = { 'name': 'Jordan 6 Rings' 'price': 57.50, 'brand_name': 'Jordan' }
Notice that our dictionary above includes colons. This is because our dictionary contains two parts: keys and values. In the above example, our keys are name
, price
, and brand_name
, and we can use them to get the value of a key.
So, if we wanted to retrieve the price of our Jordan 6 Rings, we could use the following code:
print(jordan_6s['price'])
Our code returns: 57.5. The key/value pair structure in dictionaries has a wide variety of applications in Python, and can be useful when you’re storing related data.
Sets
Python sets are unordered collections of elements. Every item in a Python set is unique, which means that no duplicates are allowed in a set. In addition, sets are immutable, and so their values cannot be changed after the set is declared.
Sets are defined as a list of values separated by commas and enclosed within curly braces ({}
).
Sets are commonly used in Python to perform specific mathematical operations such as unions or intersections. Here’s an example of a set in Python:
colors = {'Red', 'Orange', 'Yellow', 'Green'}
Conclusion
Python contains a number of built-in data types that can be used to store specific types of data. The most commonly used data types in Python are: string, integer, float, list, dictionary, set, and tuple.
In this tutorial, we explored the basics of each of these data types and discussed where they may be used in a Python program. Now you’re ready to start working with Python data types like an expert!
Python is an in-demand skill in the technology industry. Download the free Career Karma app today to talk with one of our expert coaches about how learning Python could help you break into a career in tech.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.