The Python CSV module is used to handle CSV files. CSV files can hold a lot of information, and the CSV module lets Python read and write to CSV files with the reader()
and writer()
functions.
Reading, writing, and manipulating data is an essential part of programming. Therefore, it can be useful to know how to handle files in Python, so that you can read and write data from external sources.
For example, let’s say that you are the owner of a local ice cream shop who wants to learn more about customer trends. You could use Python to analyze the data you have gathered on user orders to find out about which flavors of ice cream are popular, how much people spend on those flavors, and more.
This is where the Python CSV module comes in. CSV files are used to store a large amount of data, and the CSV module allows you to parse those files in Python. In this tutorial, we are going to explore how to read and write to CSV files using the CSV Python module.
CSV Refresher
When you’re working with data, you may have a list of that data separated by commas. For example, you may have a list of ice cream orders whose values are separated with commas. This data structure is referred to as CSV data or Comma-Separated Values.
Traditional text files can be useful for when you need to store data, but they cannot be used to store structured data. CSV files, on the other hand, allow you to store structured data in a table format that you can reference in your code, similar to how spreadsheets and databases allow you to store structured data. For example, you may have a CSV file that stores ice cream flavors or supplier information.
Here’s an example of a CSV file:
suppliers.csv Hendersons Creamery, 123 Main Street, Cream Peterson Chocolate, 129 Second Street, Chocolate Sprinkles
This file stores three items: the name of a supplier, their address, and the product with which they supply our business. Our file also stores two lines of data, one for Hendersons Creamery and the other for Peterson Chocolate.
Because our data is structured in a CSV file, we can parse it through a Python program and get data from individual columns or rows.
Python CSV Module
The Python CSV module can be used to parse CSV data in Python. So, if you have a CSV file of supplier information, for example, you can use the CSV module to retrieve and work with that data.
CSV is a built-in Python module, but before we start using it, we have to import CSV
into our program. We can do so using this code:
import csv
Reading CSV Files
Before you can work with data in a CSV file, you need to read the file. The CSV module comes with a method called reader()
which can be used to read a CSV file into our program. The reader function takes each line of a specified file and turns it into a list of columns.
Let’s say that we have a list of ice cream suppliers in a file called suppliers.csv
that we want to read into our program. The contents of the file is as follows:
Hendersons Creamery, 123 Main Street, Cream Peterson Chocolate, 129 Second Street, Chocolate Sprinkles HW Smith and Co, 17 Boston Avenue, Ice Cream Cones
We can retrieve this data using the following code:
import csv with open("suppliers.csv", "r") as f: contents = csv.reader(f) for c in contents: print(c)
There’s a lot going on here, so let’s break it down. On the first line, we import the csv
module, which allows us to use CSV methods in our code. On the next line, we use a with
statement to open up our file, and state that our file should be assigned the value f
.
Next, we use contents = csv.reader(f)
to read the contents of our file, making use of the csv.reader()
function. Finally, our program creates a for loop that loops through each line in the CSV file and prints it out to the Python shell.
Our program returns the following:
['Hendersons Creamery', ' 123 Main Street', ' Cream'] ['Peterson Chocolate', ' 129 Second Street', ' Chocolate Sprinkles'] ['HW Smith and Co', ' 17 Boston Avenue', ' Ice Cream Cones']
As you can see, our program has read the contents of our file and turned each line into an array. Now that we have this data, we can manipulate it in our program.
Let’s say that we are sending a notice to cancel our orders for next week because the shop will be closed. We could use this code to get the addresses of each supplier so we can send them the notice:
… for c in contents: print(c[1])
Our program returns:
123 Main Street 129 Second Street 17 Boston Avenue
In our code, we create a for
loop that goes through each line in our contents
variable. Then, we print out the value of each item with the index number 1
, which in this case contains the address of each supplier.
Now, let’s say that we want to read data from our file into an array. This is a common function because it allows us to store our data in another variable for later use. Here’s the code we could use to perform this action:
import csv suppliers = [] with open("suppliers.csv", "r") as f: contents = csv.reader(f) for c in contents: suppliers.append(c) print(suppliers)
Our code returns the following:
[['Hendersons Creamery', ' 123 Main Street', ' Cream'], ['Peterson Chocolate', ' 129 Second Street', ' Chocolate Sprinkles'], ['HW Smith and Co', ' 17 Boston Avenue', ' Ice Cream Cones']]
Let’s explain what is going on in our code. Our program opens the file suppliers.csv
, reads the file, then appends each record into the array suppliers
that we declared at the start of our program. Then, our program prints out the variable suppliers
.
Writing to CSV Files
The CSV module contains a method that can be used to write data to a CSV file: writer()
. The writer()
function allows us to write data to the file we specify so that we can save our data for later use.
The writer()
function creates an object that we can use to write to a file. Then, in order to write data to our file, we use the writerow()
method.
Let’s say that we have written a program that adds a new value to each supplier record. This value holds whether or not the supplier is the primary supplier for a certain product. Here’s the code we could use to write the data we have to a file:
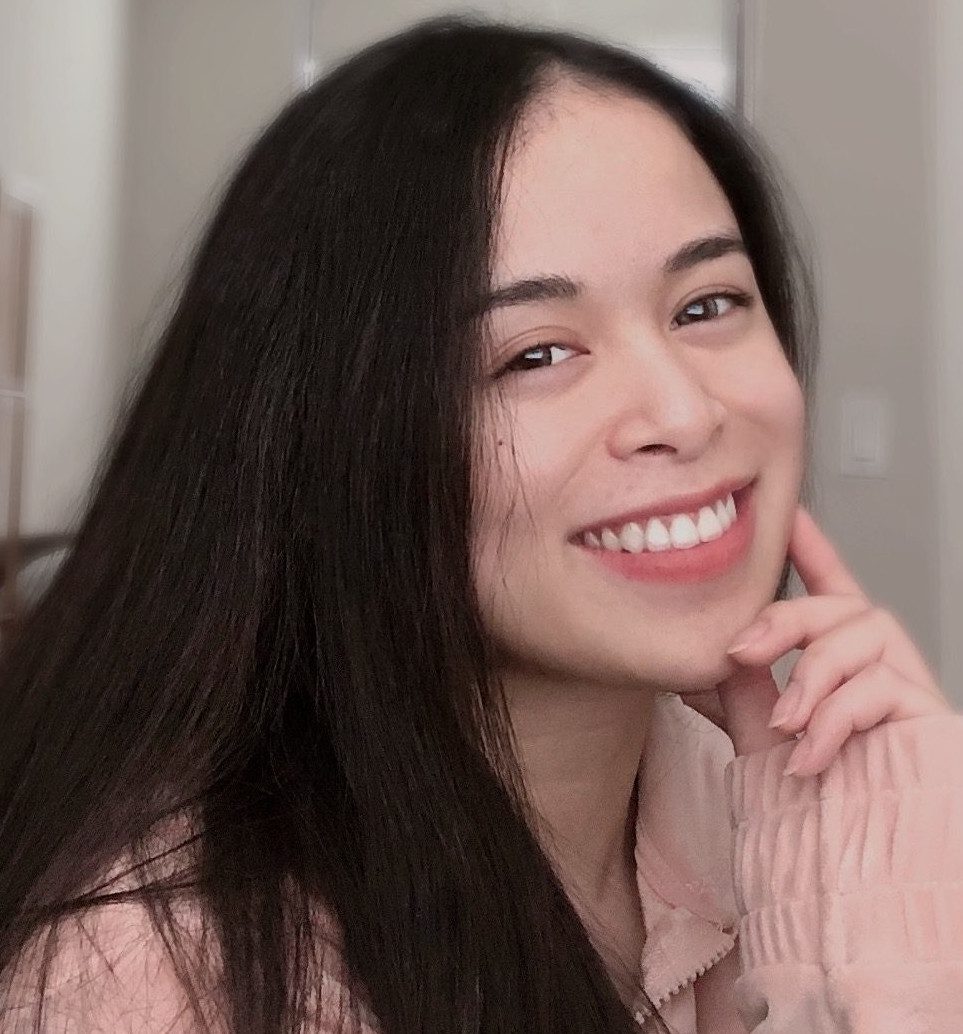
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
import csv suppliers = [] with open("suppliers.csv", "r") as f: contents = csv.reader(f) for c in contents: c.append("Yes") suppliers.append(c) with open("new_suppliers.csv", "w") as f: write_to_file = csv.writer(f) for s in suppliers: write_to_file.writerow(s)
The start of our code is very similar to our previous examples; it reads the file suppliers.csv
and moves its contents to the suppliers
array. The only difference is that the read function adds a new value called Yes
to each item in our suppliers array. This value denotes whether or not the supplier is the primary supplier of a certain product.
Next, we open up a new file called new_suppliers.csv
and write the contents of our suppliers
to that file. We use the writer()
function to prepare our file for writing.
Then, we create a for
loop that goes through our suppliers
array and uses the writerow()
method to add each supplier to the new_suppliers.csv
file.
Our code returns the following:
new_suppliers.csv Hendersons Creamery, 123 Main Street, Cream,Yes Peterson Chocolate, 129 Second Street, Chocolate Sprinkles,Yes HW Smith and Co, 17 Boston Avenue, Ice Cream Cones,Yes
However, it’s not necessary to loop through each item if we want to write our entire suppliers
variable to a file. Instead, we can use the writerows()
method to write every item in the suppliers
variable to our new_suppliers.csv
file.
Here’s the code we would use:
… write_to_file = csv.writer(f) write_to_file.writerows(suppliers) …
Our code returns:
new_suppliers.csv Hendersons Creamery, 123 Main Street, Cream,Yes Peterson Chocolate, 129 Second Street, Chocolate Sprinkles,Yes HW Smith and Co, 17 Boston Avenue, Ice Cream Cones,Yes
CSV File Quoting
In the above example, our code wrote the suppliers
array to a new file as it appeared.
However, there may be occasions when you want each value to be written to a file in quotation marks. For example, you may have a list of suppliers where a few supplier names contain commas, which would result in our CSV file becoming malformed.
The CSV module contains four quoting functions that can be used to surround the values to be written to your file in quotation marks so this does not happen. These functions are passed as an argument to the csv.writer()
method, and are as follows:
csv.QUOTE_ALL
: Surround every string in quotationscsv.QUOTE_NONNUMERIC
: Quote all string fieldscsv.QUOTE_MINIMAL
: Quote fields with special characterscsv.QUOTE_NONE
: Do not quote any values
So, let’s say that we want to quote every value in our suppliers
array when it is written into our file. We could use the following code:
… write_to_file = csv.writer(f, quoting=csv.QUOTE_ALL) …
Now, when we run our program, our new_suppliers.csv
file contains the following:
"Hendersons Creamery"," 123 Main Street"," Cream","Yes" "Peterson Chocolate"," 129 Second Street"," Chocolate Sprinkles","Yes" "HW Smith and Co"," 17 Boston Avenue"," Ice Cream Cones","Yes"
Conclusion
Reading data from and writing data to files allows you to retrieve and store data generated from your Python program. For example, if you have a menu of ice cream flavors and prices that you want to work within a Python program, you may want to store the data in a file.
In this tutorial, we explored the basics of the Python CSV module and how it works. We also discussed how to read and write files using the reader()
and writer()
objects.
Now you’re ready to read data from and write data to CSV files using Python like a professional!
Companies all over the world are hiring people who know how to code in Python. Download the free Career Karma app today to find out more about how learning Python could help you break into a career in tech!
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.