The Python os.mkdir() method creates a blank directory on your file system. You cannot use this method to create a folder in a folder that does not exist. The os.mkdirs() method recursively creates a blank directory.
Files let you store data outside a program that can be referenced by a program in the future. When you’re working with files, you may decide to create a new directory in which a file should be stored.
That’s where the Python os module comes in. The os module includes two methods called os.mkdir() and os.mkdirs() which can be used to create directories in Python.
This tutorial will explore how to create a directory in Python using the os.mkdir() and os.mkdirs() methods. We will also use a few examples to show how these methods work.
Python os Refresher
Before you can work with files in Python, you need to import the os module. The os module is built-in to Python. It contains a number of methods that can be used to interface with a computer’s underlying operating system.
In this case, we are interested in the os module’s file system methods, which can be used to work with files. We can use a Python import statement to import os into our program:
Python Create Directory
The os.mkdir() method can be used to create a single directory. For example, if we were creating a program that needs a new folder to store its outputs, we would use os.mkdir().
The syntax for the os.mkdir() method is as follows:
os.mkdir(path, access)
The os.mkdir() function takes in two parameters:
- path is the file path at which you want to create your directory (required)
- access refers to the access rights you want to grant your directory (optional)
Python os.mkdir() Example
Let’s explore an example to showcase how to use the os.mkdir() method.
Say we are creating a program that analyzes a list of math student test scores. This program stores whether each student has passed the latest test.
To start, we want to create a directory where our data will be stored. This will ensure that our data will be separate from the rest of our program.
Here’s the code we could use to create a directory for our data:
import os path = "/home/school/math/grades/final" os.mkdir(path) print("/home/school/math/grades/final has been created.")
Our code creates a new directory called final in the /home/school/math/grades folder in our operating system. Then, it returns the message:
/home/school/math/grades/final has been created.
The mkdir() method can only be used to create one directory at a time. We could not create a folder called final and then create a folder within final called jan2020.
os.mkdir() Permissions Example
We can use the optional access parameter to specify the permissions we want our directory to have. By default, the access permissions for the directory mkdir() creates is 777. This means the directory is readable and writable by the owner and all other users.
But what if we want to set our own permissions?
Say that our operating system is accessible to multiple teachers, and we only want to grant write access to the owner. We need to use the 755 permission. This permission states a file is readable and accessible by all users. But, only the owner can write to the file.
Here’s the code we could use to create a working directory with custom permissions:
import os path = "/home/school/math/grades/final" access = 0o755 os.mkdir(path, access) print("/home/school/math/grades/final has been created.")
We have created a target directory called /home/school/math/grades/final. This directory has the access permission 755. This means that our file can be read by all users, but only the owner can write to the file. Then, our program prints the message:
/home/school/math/grades/final has been created.
It’s important to note that our access variable is equal to 0o755 in our code. We have done this because access rights use the octal prefix, and so we need to specify 0o before our access parameter.
To learn how to check if a file or directory exists, check out our tutorial on checking if files exist in Python.
Python Create Multiple Directories
The Python os.makedirs() method recursively creates folders. You can use this method to create a folder inside a folder that does not exist. os.mkdirs() accepts one argument: the path of the folder you want to create.
Let’s take a look at the syntax for os.mkdirs():
os.mkdirs(path, access)
This method accepts the same arguments as os.mkdir().
Python os.mkdirs() Example
There are often cases where we need to create directories that exist within other new directories.
For instance, say we are creating a program that stores our math student test score data. Instead of storing all of our data in one folder, we could store data in multiple folders.
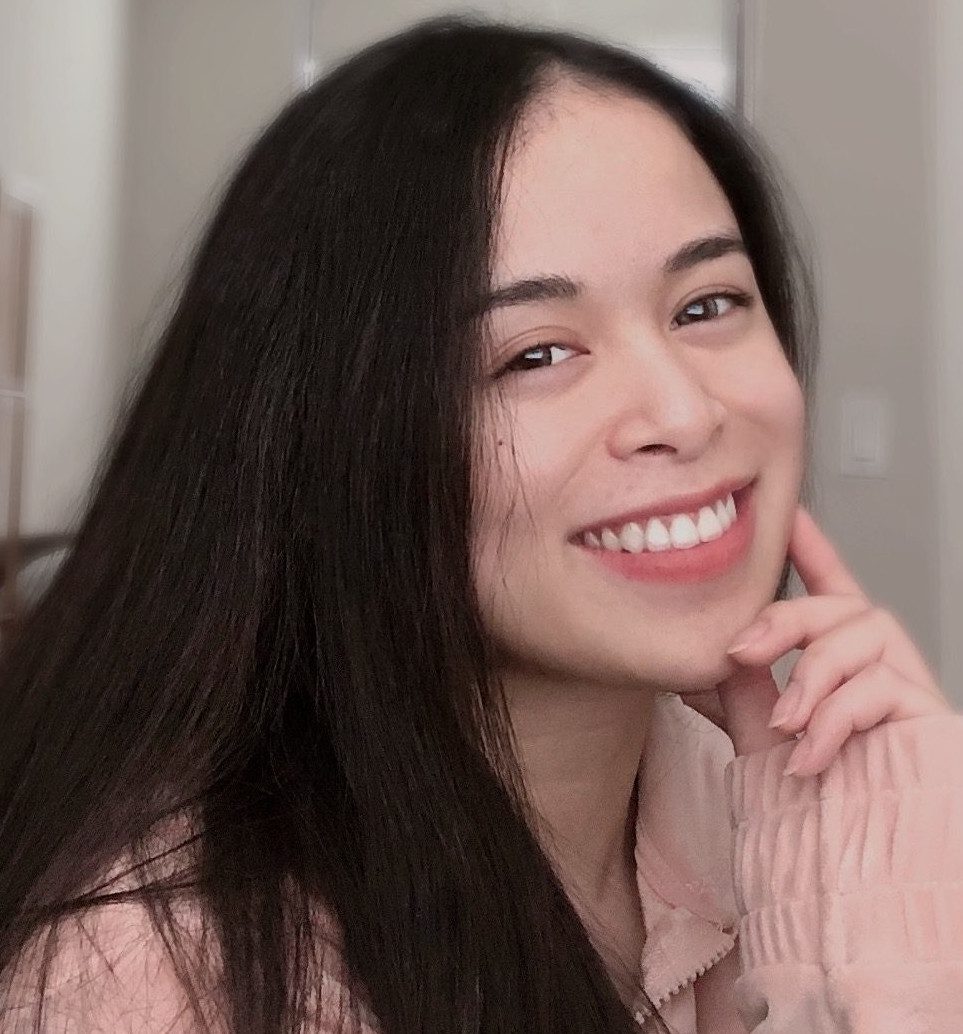
"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
Each folder would correspond with the year and month of the test. Storing data in this way would make our files easier to find if and when we want to refer back to them.
Let’s use our school math test example to illustrate how the os.makedirs() method works.
The specifications of our program have changed. Now we want to store data in folders depending on the year and month of the test. We could accomplish this task using the following code:
import os path = "/home/school/math/grades/2020/02" access = 0o755 os.mkdir(path, access) print("/home/school/math/grades/2020/02 has been created.")
Let’s say that we do not yet have a 2020 folder or an 02 folder. This code would first create the 2020 folder, then it would create the folder called 02 within 2020. We assign this folder the access permissions 755.
After our program creates our folder, it prints out the message:
/home/school/math/grades/2020/02 has been created.
Conclusion
Creating a directory is a common operation in Python when you’re working with files. The os.mkdir() method can be used to create a single directory, and the os.makedirs() method can be used to create multi-level directories.
This tutorial discussed how to use both os.mkdir() and os.makedirs() to create folders in Python. We also explored an example of each of these methods in action.
Are you interested in learning more about Python? Check out our complete How to Learn Python guide for expert tips and advice on how to learn this programming language.
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication.